Vue.js Prop One-Way Data Flow
Last Updated :
13 Oct, 2023
In VueJS, all the props are bonded between the child and properties is one-way-down. When we update the prop in the parent component, it is updated to all the child components, but the reverse does not happen and VueJS warns the use. This is used as protection from altering the state of a parent-by-child component.
Different Cases where the Child Component tries to change Props
The following are the cases where the child component tries to modify the Props:
- When the Child component gets props from the parent and wants to use it as a local data property. In such a case, we can use a local variable of the child component that is initialized with props value.
props = defineProps( ['props1'] );
// Variable intialize with props value
temp = props1;
- When we need some props that are not in the final data, it is raw data for final data. In such a case, we can use computed property with the prop’s value.
props = defineProps( ['firstName', 'lastName' ]);
// Applying computed property on props
fullName = computed( () => firstName + lastName );
Mutating Object / Array Props
One-way data binding only prevents the String, Number, and Boolean, but, it does not prevent the mutation of array and object. Vue.js does not prevent the array and object mutation because the array and object are passed as a reference to the child and prevention is expensive as compared to others. Such mutation affects the parent state and makes the process of understanding the flow of data between components more difficult. We should allow such mutation only when parent and child are tightly bound or the child should trigger an event that tells the parent to change props data.
Example 1: In this example, we get the props data from the parent, and use it as a local variable, and change some props value by local variables.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student Sname = "Sam"
SAge = "23"
SBatch = "3rd"
SCourse = "Mtech" />
</ template >
< script >
import Student from './components/Student.vue';
export default {
name: 'App',
components: {
Student,
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >
Chick on botton to view the props
</ h2 >
< button v-on:click.left = "temp = !temp" >
Show
</ button >
< h2 v-if = "temp" >
Name : {{ name }} < br />
Course : {{ Course }} < br />
Age : {{ Age }}< br />
Batch : {{ Batch }}< br />
< button @ click = "name='Satyam'" >
change Name
</ button >
</ h2 >
</ template >
< script >
export default {
name: 'StudentC',
props: ['Sname', 'SAge', 'SCourse', 'SBatch'],
data() {
return {
name: this.Sname,
Age: this.SAge,
Batch: this.SBatch,
Course: this.SCourse,
temp: false
}
},
}
</ script >
|
Output:
.gif)
props
Example 2: In this example, we will get props from the user as raw data and perform some operations on values.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student Sname = "Sam Snehil"
Age = "23"
SCourse = "Btech"
SBatch = "3rd" />
</ template >
< script >
import Student from './components/Student.vue';
export default {
name: 'App',
components: {
Student,
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >
Chick on botton to view the props
</ h2 >
< button v-on:click.left = "temp = !temp" >
Show
</ button >
< h2 v-if = "temp" >
Name : {{ name }}< br />
Course : {{ course }} < br />
Age : {{ Age }}< br />
Batch : {{ batch }}< br />
< button @ click = "name='Satyam'" >
change Name
</ button >
</ h2 >
</ template >
< script >
export default {
name: 'StudentC',
props: ['Sname', 'Age', 'SCourse', 'SBatch'],
computed: {
name: function () {
return this.Sname.toUpperCase();
},
course: function () {
return this.SCourse.toUpperCase()
},
batch: function () {
return this.SBatch.toUpperCase()
},
},
data() {
return { temp: false };
}
}
</ script >
|
Output:
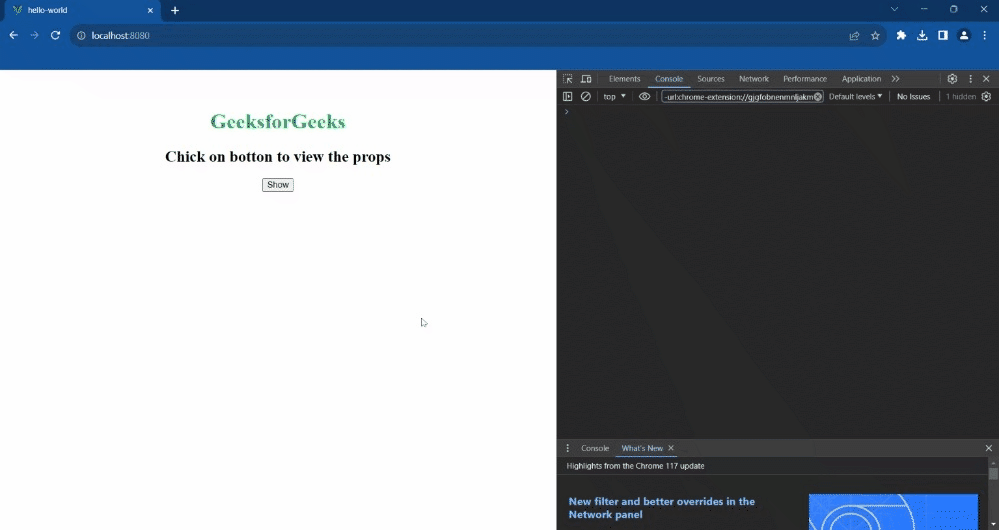
one way
Reference: https://vuejs.org/guide/components/props.html#one-way-data-flow
Share your thoughts in the comments
Please Login to comment...