URL Monitoring Service with Node and Express.js
Last Updated :
26 Feb, 2024
Learn to set up a URL Monitoring Service with NodeJS and ExpressJS. It tracks URL statuses and notifies of any changes. This course emphasizes simplicity for beginners while guiding on scalable and clean code practices. The system conducts health checks on multiple URLs to ensure availability over time.
Output Preview: Let us have a look at how the final output will look like.
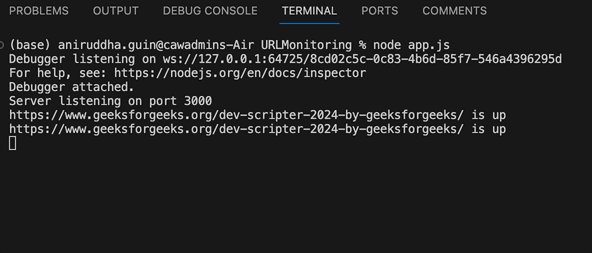
Desired Output
Prerequisites:
Approach to create URL Monitoring Service
- We will use a in memory data structure (kind of map) to store the url and its health check status
- setTimeOut is a very handy function that we will use here to check the url status at regular intervals
- Basically, at every interval we will use axios library to make a HTTP call to the target url for their status check.
- We will need to have another endpoint to stop the monitoring else it will keep on going to monitor.
Steps to Create URL Monitoring Service in Nodejs and Expressjs
Step 1: Create a project directory:
mkdir url-monitoring
cd url-monitoring-app
Step 2: Initialize Node.js project:
npm init -y
Step 3: Install dependencies:
npm install express axios body-parser cors request
Project Structure:
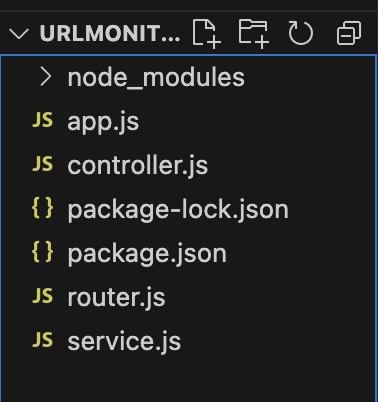
Project Structure
The updated dependencies in package.json file will lo:
"dependencies": {
"axios": "^1.6.7",
"cors": "^2.8.5",
"express": "^4.18.2",
"request": "^2.88.2"
}
Example: Below is the code example of the URL Monitoring Service:
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const cors = require( 'cors' );
const router = require( './router' );
const app = express();
const port = process.env.PORT || 3000;
app.use(bodyParser.json());
app.use(cors());
app.use(router);
app.listen(port, () => {
console.log(`Server listening on port ${port}`);
});
|
Javascript
const monitoringService = require( './service' );
const monitorUrl = (req, res) => {
const { url, interval } = req.body;
if (!url || !interval) {
return res.status(400)
.send( 'Missing URL or interval' );
}
monitoringService.startMonitoring(url, interval);
res.send(
`Started monitoring ${url}
every ${interval} milliseconds`
);
};
const stopMonitoring = (req, res) => {
const { url } = req.body;
if (monitoringService.stopMonitoring(url)) {
res.send(
`Stopped monitoring ${url}`
);
} else {
res.status(404)
.send(`Was not monitoring ${url}`);
}
};
module.exports = { monitorUrl, stopMonitoring };
|
Javascript
const express = require( 'express' );
const router = express.Router();
const monitoringController = require( './controller' );
router.post( '/monitor' , monitoringController.monitorUrl);
router.post( '/stop' , monitoringController.stopMonitoring);
module.exports = router;
|
Javascript
const axios = require( 'axios' );
const monitoredUrls = {};
const startMonitoring = (url, interval) => {
if (!monitoredUrls[url]) {
monitoredUrls[url] =
setInterval(async () => {
try {
await axios.get(url);
console.log(`${url} is up`);
} catch (error) {
console.log(`${url} might be down`);
}
}, interval);
}
};
const stopMonitoring =
(url) => {
if (monitoredUrls[url]) {
clearInterval(monitoredUrls[url]);
delete monitoredUrls[url];
return true ;
}
return false ;
};
module.exports = { startMonitoring, stopMonitoring };
|
Steps to Run the App:
node index.js
Output:
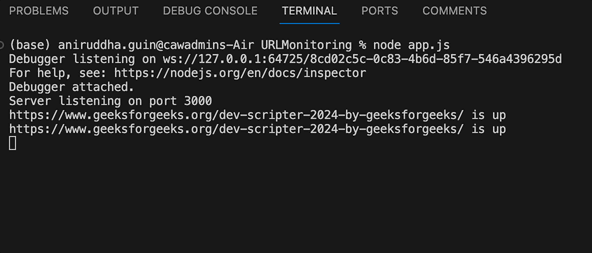
Share your thoughts in the comments
Please Login to comment...