How to handle URL parameters in Express ?
Last Updated :
04 Jan, 2024
In this article, we will discuss how to handle URL parameters in Express. URL parameters are a way to send any data embedded within the URL sent to the server. In general, it is done in two different ways.
Steps to Create Express Application:
Let’s implement the URL parameter in an Express Application and see it in action.
Step 1: Create an Express Application with the below commands.
npm init -y
Step 2: Install Express library with npm and add it to dependency list
npm install --save express
Example: Adding server.js
- In the project directory, create a file named index.js.
- Create an express server in the file and listen for connections.
Javascript
const app = require( "express" )();
const PORT = 8080;
app.listen(PORT, () => {
console.log(`server is listening at port:${PORT}`);
});
|
- In the above code, an express app is created by importing the express library.
- Server is listening in the 8080 Port for any incoming request, on successful start server will print a log in the console.
Approach 1: Using queries
In this method, the URL parameter will be send in the URL as queries. Queries are appended at the end of actual url with a question character “?” appended at the last. Every query value will be mapped as key – value pairs and will be delimited with an ampersand “&” symbol.
http://example.com/api/?id=101&name=ragul
In the above example the queries are passed like below key – value pairs
Example: Using queries:
- To use URL parameters in type of queries, use the “req.query” property from request object in Express.
- Express will automatically add the queries from URL in the req.query as a Javascript Object.
Javascript
const app = require( "express" )();
const PORT = 8080;
app.get( "/api" , (req, res) => {
const queries = req.query;
res.send(queries);
});
app.listen(PORT, () => {
console.log(`server is listening at port:${PORT}`);
});
|
- queries are being read from req.query and just simply returned as response.
Output:
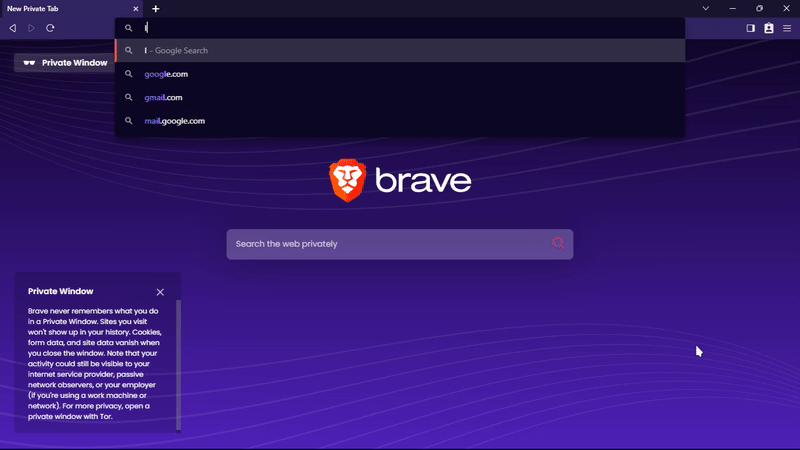
using URL parameters with req.query
Approach 2: Using Route parameter
In route parameters, instead of sending as queries, embed the value inside the actual URL itself.
For example
http://example.com/api/:id/:name
Here, userid and username are placeholder for route parameter. To use the above URL parameter send the request like below.
http://example.com/api/101/ragul
- :userid is mapped to 101
- :username is mapped to ragul
Example: Using route params
- To use the route parameter, define the parameters in the URL of the request.
- Every parameter should be preceded by a colon “:”
- Access the route parameters from the request object’s params property, it will return a Javascript Object.
Javascript
const app = require( "express" )();
const PORT = 8080;
app.get( "/api/:id/:name" , (req, res) => {
const params = req.params;
res.send(params);
});
app.listen(PORT, () => {
console.log(`server is listening at port:${PORT}`);
});
|
The above code will map the id to the immediate value next to “/api/” and for the name it will add the second next value in the URL parameter.
Output:
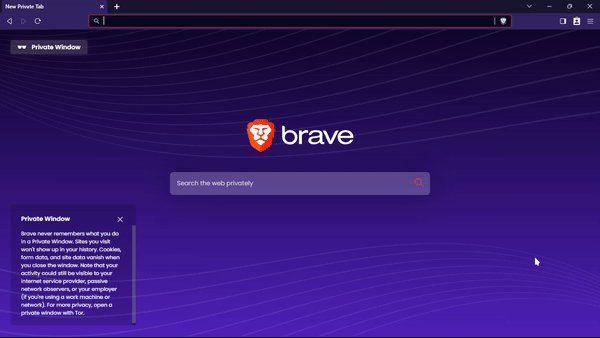
using URL parameters with req.params
Share your thoughts in the comments
Please Login to comment...