How to get url after “#” in Express Middleware Request ?
Last Updated :
03 Jan, 2024
In Express, the fragment identifier (the part of the URL after the # symbol) is not directly accessible on the server side because it is not sent to the server as part of the HTTP request. The fragment identifier is typically used for client-side navigation and is processed on the client side only.
If we need to access data from the fragment identifier on the server side, we can use JavaScript on the client side to send that data to the server, either through an AJAX request or by including it in a regular HTTP request.
Prerequisites:
Approach to get URL after “#” in Express:
Client-Side (Browser):
- Retrieve Fragment Identifier:
- Use window.location.hash to get the fragment identifier.
- Store the value in a variable (e.g., fragmentValue).
- Send Data to Server (AJAX):
- Create an XMLHttpRequest (xhr) object.
- Open a POST request to the server endpoint (/processFragment).
- Set the request header to ‘application/json’.
- Send a JSON object containing the fragment value to the server.
Server-Side (Express):
- Receive and Process Data:
- Use Express to handle incoming POST requests at the endpoint (/processFragment).
- Utilize the body-parser middleware to parse JSON data from the request body.
- Access Fragment Value:
- Retrieve the fragment value from req.body.fragment.
- Process and Respond:
- Process the fragment value as needed on the server side.
- Respond to the client, indicating that the fragment value has been received and processed.
Steps to Create an Express Application:
Step 1: In the first step, we will create the new folder by using the below command in the VScode terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this, the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm install express body-parser
Project Structure:
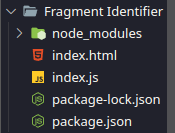
File Structure
The updated dependencies in the package.json file will look like:
"dependencies": {
"body-parser": "^1.20.2",
"express": "^4.18.2",
}
Example: Below is the code of above explained approach
Javascript
const express = require( "express" );
const bodyParser = require( "body-parser" );
const cors = require( "cors" );
const app = express();
const port = 3000;
app.use(cors());
app.use(bodyParser.json());
app.post( "/processFragment" , (req, res) => {
const fragmentValue = req.body.fragment;
console.log( 'Fragment value:' , fragmentValue);
const responseMessage =
`Fragment value received and processed.
\nInput Value: ${fragmentValue}`;
res.send(responseMessage);
});
app.listen(port, () => {
console.log(`Server listening
at http:
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Express Fragment Identifier Example</ title >
</ head >
< style >
body {
font-size: 40px;
}
input,
button {
font-size: 25px;
}
</ style >
< body >
< p id = "responseContent" ></ p >
< form id = "myForm" >
< label for = "fragmentInput" >Example Fragment:</ label >
< input type = "text" id = "fragmentInput"
name = "fragment" placeholder = "enter here" >
< button type = "button" onclick = "submitForm()" >
Submit
</ button >
</ form >
< script >
function submitForm() {
var fragmentValue =
document.getElementById('fragmentInput').value;
var xhr = new XMLHttpRequest();
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
// Display the response content
document
.getElementById('responseContent')
.innerText = xhr.responseText;
} else {
// Update the status message on error
document.getElementById('status')
.innerText = 'Error processing fragment value.';
// Clear the response content on error
document.getElementById('responseContent').innerText = '';
}
}
};
// Send the request with the entered fragment value
xhr.send(JSON.stringify({ fragment: fragmentValue }));
}
</ script >
</ body >
</ html >
|
Step 4: Run the below command to start the server
node index.js
Output:
.gif)
Output
Share your thoughts in the comments
Please Login to comment...