Traffic Light Control Project using Arduino
Last Updated :
18 Apr, 2024
Arduino is an open-source electronics platform based on easy-to-use hardware and software. Arduino boards can read digital & analog inputs from the sensors. With Arduino, you can write and upload computer code to a physical programmable circuit board (commonly called a microcontroller which is ATmega328P 8-bit Microcontroller) using a piece of software called the Arduino IDE (Integrated Development Environment), which runs on your computer.
The Arduino is the most widely used open-source microcontroller board for numerous electronics and do-it-yourself projects. The Arduino traffic light controller is a simple project. Nowadays, everyone desires to drive their own car. As a result, the number of cars on the road is constantly rising, resulting in traffic bottlenecks.
A traffic light controller assists in traffic management and traffic control. These devices are installed at road crossroads or crossings to prevent traffic congestion and accidents. The systems alert the motorist by employing various colours of light. As a result, avoiding congestion at junctions is straightforward.
In this project, we are going to make a traffic light control for a four-way intersection. We will learn about traffic lights and how they work through an electronic project. This project is a condensed version of the four-side or direction traffic light system that we have exhibited.
Required components:
- Arduino
- 12 pieces of 220-ohm resistors
- 4 pieces of  breadboard
- Connecting wires
- Red, yellow, and green LEDs
Circuit Diagram:
Connect the components as mentioned in the circuit diagram
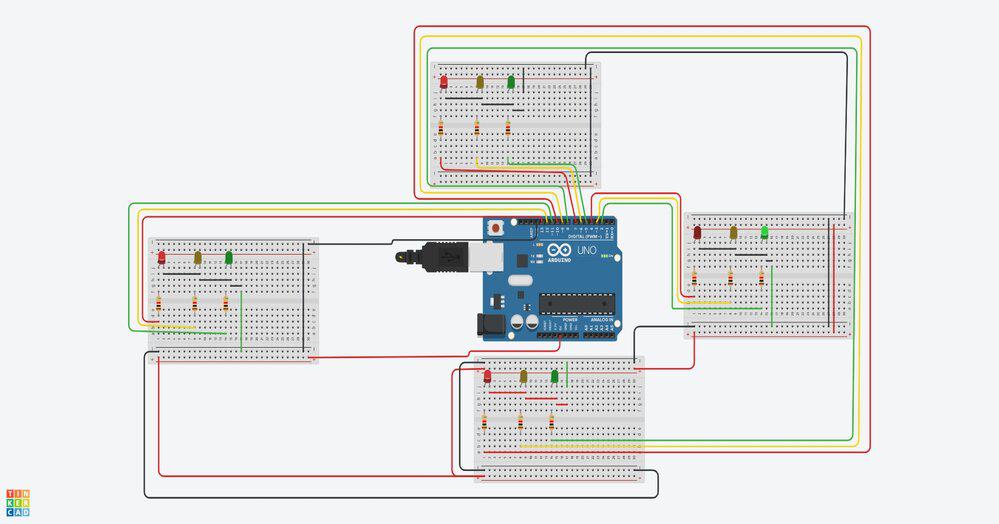
Circuit Diagram
Code for the Arduino Traffic LightÂ
1-Assign the traffic lights pins to variablesÂ
int d_red =10;
int d_yellow =9;
int d_green =8;
int r_red =4;
int r_yellow =3;
int r_green =2;
int l_red =13;
int l_yellow =12;
int l_green =11;
int u_red =7;
int u_yellow =6;
int u_green =5;
2-Configure the traffic lights as outputs
void setup()
{
pinMode(d_red, OUTPUT);
pinMode(d_yellow, OUTPUT);
pinMode(d_green, OUTPUT);
pinMode(r_red, OUTPUT);
pinMode(r_yellow, OUTPUT);
pinMode(r_green, OUTPUT);
pinMode(l_red, OUTPUT);
pinMode(l_yellow, OUTPUT);
pinMode(l_green, OUTPUT);
pinMode(u_red, OUTPUT);
pinMode(u_yellow, OUTPUT);
pinMode(u_green, OUTPUT);
}
3-Use loop function to keep the lights in a loop and use changeLIght() function to carry out the logic
void loop()
{
changeLights();
}
void changeLights()
{
//Start (all yellow)
digitalWrite(u_red,LOW);
digitalWrite(d_red,LOW);
digitalWrite(r_red,LOW);
digitalWrite(l_green,LOW);
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
delay(5000);
//upper lane go
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_green,HIGH);
digitalWrite(r_red,HIGH);
digitalWrite(l_red,HIGH);
digitalWrite(d_red,HIGH);
delay(10000);
//ALL YELLOW
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
digitalWrite(u_green,LOW);
digitalWrite(r_red,LOW);
digitalWrite(l_red,LOW);
digitalWrite(d_red,LOW);
delay(5000);
//RIGHT LANE GO
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_red,HIGH);
digitalWrite(l_red,HIGH);
digitalWrite(d_red,HIGH);
digitalWrite(r_green,HIGH);
delay(10000);
//ALL YELLOW ON
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
digitalWrite(u_red,LOW);
digitalWrite(l_red,LOW);
digitalWrite(d_red,LOW);
digitalWrite(r_green,LOW);
delay(5000);
//DOWN LANE GO
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_red,HIGH);
digitalWrite(l_red,HIGH);
digitalWrite(r_red,HIGH);
digitalWrite(d_green,HIGH);
delay(10000);
//ALL YELLOW
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
digitalWrite(u_red,LOW);
digitalWrite(l_red,LOW);
digitalWrite(r_red,LOW);
digitalWrite(d_green,LOW);
delay(5000);
//LEFT LANE GO
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_red,HIGH);
digitalWrite(d_red,HIGH);
digitalWrite(r_red,HIGH);
digitalWrite(l_green,HIGH);
delay(10000);
}
Explanation:-Here the loop start with all the yellow light turned on and the green light moving in a clockwise direction when one of the green light is on the rest of the direction will be red and it all will do a transition with the yellow light turned on in every direction.
4-Compile the code and upload it to the Arduino
C++
int d_red =10;
int d_yellow =9;
int d_green =8;
int r_red =4;
int r_yellow =3;
int r_green =2;
int l_red =13;
int l_yellow =12;
int l_green =11;
int u_red =7;
int u_yellow =6;
int u_green =5;
void setup()
{
pinMode(d_red, OUTPUT);
pinMode(d_yellow, OUTPUT);
pinMode(d_green, OUTPUT);
pinMode(r_red, OUTPUT);
pinMode(r_yellow, OUTPUT);
pinMode(r_green, OUTPUT);
pinMode(l_red, OUTPUT);
pinMode(l_yellow, OUTPUT);
pinMode(l_green, OUTPUT);
pinMode(u_red, OUTPUT);
pinMode(u_yellow, OUTPUT);
pinMode(u_green, OUTPUT);
}
void loop()
{
changeLights();
}
void changeLights()
{
//Start (all yellow)
digitalWrite(u_red,LOW);
digitalWrite(d_red,LOW);
digitalWrite(r_red,LOW);
digitalWrite(l_green,LOW);
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
delay(5000);
//upper lane go
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_green,HIGH);
digitalWrite(r_red,HIGH);
digitalWrite(l_red,HIGH);
digitalWrite(d_red,HIGH);
delay(10000);
//ALL YELLOW
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
digitalWrite(u_green,LOW);
digitalWrite(r_red,LOW);
digitalWrite(l_red,LOW);
digitalWrite(d_red,LOW);
delay(5000);
//RIGHT LANE GO
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_red,HIGH);
digitalWrite(l_red,HIGH);
digitalWrite(d_red,HIGH);
digitalWrite(r_green,HIGH);
delay(10000);
//ALL YELLOW ON
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
digitalWrite(u_red,LOW);
digitalWrite(l_red,LOW);
digitalWrite(d_red,LOW);
digitalWrite(r_green,LOW);
delay(5000);
//DOWN LANE GO
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_red,HIGH);
digitalWrite(l_red,HIGH);
digitalWrite(r_red,HIGH);
digitalWrite(d_green,HIGH);
delay(10000);
//ALL YELLOW
digitalWrite(u_yellow,HIGH);
digitalWrite(d_yellow,HIGH);
digitalWrite(r_yellow,HIGH);
digitalWrite(l_yellow,HIGH);
digitalWrite(u_red,LOW);
digitalWrite(l_red,LOW);
digitalWrite(r_red,LOW);
digitalWrite(d_green,LOW);
delay(5000);
//LEFT LANE GO
digitalWrite(u_yellow,LOW);
digitalWrite(d_yellow,LOW);
digitalWrite(r_yellow,LOW);
digitalWrite(l_yellow,LOW);
digitalWrite(u_red,HIGH);
digitalWrite(d_red,HIGH);
digitalWrite(r_red,HIGH);
digitalWrite(l_green,HIGH);
delay(10000);
}
Output:
FAQs
Share your thoughts in the comments
Please Login to comment...