Sentiment Analysis using JavaScript and API
Last Updated :
03 Oct, 2023
Sentiment Analysis – Sentiment analysis, also known as opinion mining, is a computational technique used to determine and categorize the emotional tone or attitude expressed in a piece of text. Analyzing sentiment in text is a crucial task in natural language processing, allowing us to understand the emotional tone and attitude of the content. In this article, we will explore how to utilize the MeaningCloud API along with JavaScript to analyze the sentiment of an inputted text. MeaningCloud provides powerful sentiment analysis capabilities that can help us gain insights into the sentiment expressed in textual data.
Final Output Preview:
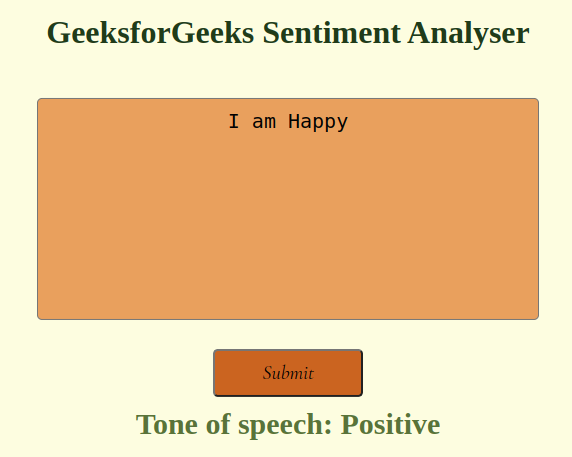
Prerequisites:
Before getting started, make sure you have the following:
- A MeaningCloud API key: Sign up on the MeaningCloud website to obtain an API key that grants access to their sentiment analysis service.
- Basic knowledge of JavaScript, HTML and CSS.
Approach:
- JavaScript Code Integration: Write JavaScript code to handle the user’s input, communicate with the MeaningCloud API, and retrieve the sentiment analysis results.
- MeaningCloud API Integration: Incorporate the MeaningCloud API into your JavaScript code. Make an API request using the input text to send it for sentiment analysis.
- API Response Parsing: Receive the API response, which will contain sentiment-related information such as polarity (positive, negative, or neutral).
Below is the guidance for creating a web page that allows users to input text, and analyze the sentiment of the text using the MeaningCloud API.
Step-1 Create an HTML Page with Input – Start by creating an HTML page that includes a form where users can input text.
Step-2 Create a MeaningCloud API Account – Go to the MeaningCloud website and create an account. Once you have an account, you’ll be able to access the API key required for making API requests.
Step-3Make an API Request – Use JavaScript to make an API request to analyze the sentiment of the input text using the MeaningCloud API. Replace ‘YOUR_API_KEY’ with your actual API key.
Project Structure :
--index.html
--style.css
--script.js
Example : Write the following code in respective files:
- index.html: Web page structure with HTML elements.
- style.css: Adds visual styles and layout to the page.
- script.js: Makes API call and fetches content, generating dynamic updates.
Javascript
function analyzeSentiment(event) {
event.preventDefault();
const apiKey = "YOUR_API_KEY" ;
const text = document.getElementById( "content" ).value;
const url =
`https:
text
)}&lang=en`;
fetch(url)
.then((response) => {
if (response.ok) {
return response.json();
} else {
throw new Error( "Request failed" );
}
})
.then((data) => {
const sentiment = data.score_tag;
if (sentiment == "P+" ) {
document.getElementById( "result" ).innerHTML = "Strong Positive" ;
}
if (sentiment == "P" ) {
document.getElementById( "result" ).innerHTML = "Positive" ;
}
if (sentiment == "NEU" ) {
document.getElementById( "result" ).innerHTML = "Neutral" ;
}
if (sentiment == "N" ) {
document.getElementById( "result" ).innerHTML = "Negative" ;
}
if (sentiment == "N+" ) {
document.getElementById( "result" ).innerHTML = "Strong Negative" ;
}
if (sentiment == "NONE" ) {
document.getElementById( "result" ).innerHTML =
"Without Polarity" ;
}
})
. catch ((error) => {
console.error( "Error:" , error);
});
}
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Sentiment Analysis</ title >
< link rel = "stylesheet" href = "./style.css" />
</ head >
< body >
< div >
< form onsubmit = "analyzeSentiment(event)" >
< h1 >GeeksforGeeks Sentiment Analyser</ h1 >
< textarea id = "content"
placeholder = "Type the text here to analyze sentiments!" >
</ textarea >
< br />
< input type = "submit" />
< div id = "resultStyle" > Tone of speech: < span id = "result" ></ span ></ div >
</ form >
</ div >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
CSS
body,
html {
margin : 0 ;
background-color : #fefae0 ;
}
h 2 {
margin : 0 ;
}
.hero-text,
form {
text-align : center ;
position : absolute ;
top : 50% ;
left : 50% ;
transform: translate( -50% , -50% );
color : #283618 ;
}
.hero-text button,
input[type= "submit" ] {
outline : 0 ;
display : inline- block ;
width : 150px ;
padding : 10px 0px ;
border-radius: 5px ;
color : #000000 ;
background-color : #bc6c25 ;
text-align : center ;
cursor : pointer ;
margin-top : 5% ;
font-size : 20px ;
font-family : 'Cormorant' , serif ;
}
.hero-text button:hover,
input[type= "submit" ]:hover {
border : 1px solid #dda15e ;
}
textarea {
outline : 0 ;
padding : 10px 0px ;
border-radius: 5px ;
color : #000000 ;
background-color : #dda15e ;
text-align : center ;
margin-top : 5% ;
font-size : 20px ;
resize: none ;
}
#resultStyle {
margin : 10px ;
font-size : 30px ;
color : #606c38 ;
font-weight : bolder ;
}
a {
color : white ;
text-decoration : none ;
}
::-webkit-input-placeholder {
color : white ;
}
:-ms-input-placeholder {
color : white ;
}
::placeholder {
color : white ;
}
@media ( min-width : 420px ) {
textarea {
width : 400px ;
height : 170px ;
}
}
@media ( min-width : 520px ) {
textarea {
width : 500px ;
height : 200px ;
}
}
|
Output:
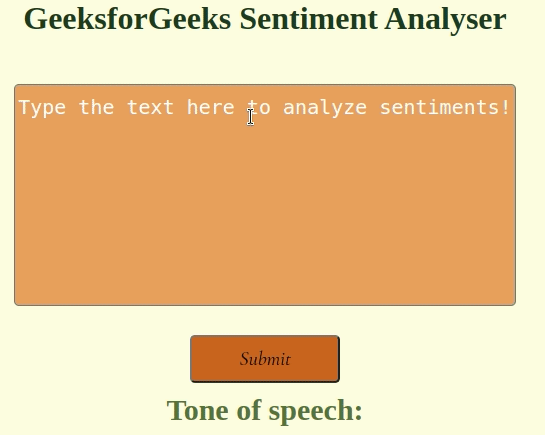
Share your thoughts in the comments
Please Login to comment...