Star Rating using HTML CSS and JavaScript
Last Updated :
22 Dec, 2023
Star rating is a way to give a rating to the content to show its quality or performance in different fields. This article will demonstrate how to create a star rating project using JavaScript.
Output Preview:
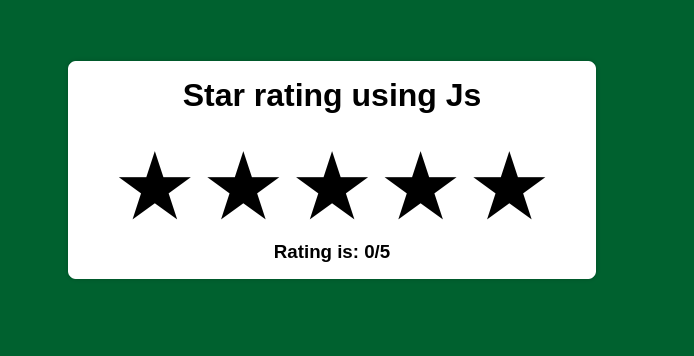
Prerequisites
Approach
- Create the basic structure using HTML entities, divs, and spans along with the corresponding class names. Using CSS styling properties, style the components with CSS properties like display, color, font size, margin, padding, and border for respective classes.
- In JavaScript access the html element using a document.getElementByClass name to get the list of span elements and getElementById.
- Use onclick attribute as an event listener to call the function according to the no. of star clicked.
- Remove already applied CSS by calling the remove method.
- Update the color of the stars and the output corresponding to the author’s action.
Example: In this example, we will create the star rating with the above approach.
Javascript
let stars =
document.getElementsByClassName( "star" );
let output =
document.getElementById( "output" );
function gfg(n) {
remove();
for (let i = 0; i < n; i++) {
if (n == 1) cls = "one" ;
else if (n == 2) cls = "two" ;
else if (n == 3) cls = "three" ;
else if (n == 4) cls = "four" ;
else if (n == 5) cls = "five" ;
stars[i].className = "star " + cls;
}
output.innerText = "Rating is: " + n + "/5" ;
}
function remove() {
let i = 0;
while (i < 5) {
stars[i].className = "star" ;
i++;
}
}
|
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >Star Rating</ title >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 " />
< link rel = "stylesheet"
href = "styles.css" />
</ head >
< body >
< div class = "card" >
< h1 >JavaScript Star Rating</ h1 >
< br />
< span onclick = "gfg(1)"
class = "star" >★
</ span >
< span onclick = "gfg(2)"
class = "star" >★
</ span >
< span onclick = "gfg(3)"
class = "star" >★
</ span >
< span onclick = "gfg(4)"
class = "star" >★
</ span >
< span onclick = "gfg(5)"
class = "star" >★
</ span >
< h3 id = "output" >
Rating is: 0/5
</ h3 >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
* {
margin : 0 ;
padding : 0 ;
box-sizing: border-box;
}
body {
min-height : 50 vh;
display : flex;
align-items: center ;
text-align : center ;
justify- content : center ;
background : hsl( 137 , 46% , 24% );
font-family : "Poppins" , sans-serif ;
}
.card {
max-width : 33 rem;
background : #fff ;
margin : 0 1 rem;
padding : 1 rem;
box-shadow: 0 0 5px rgba( 0 , 0 , 0 , 0.2 );
width : 100% ;
border-radius: 0.5 rem;
}
.star {
font-size : 10 vh;
cursor : pointer ;
}
.one {
color : rgb ( 255 , 0 , 0 );
}
.two {
color : rgb ( 255 , 106 , 0 );
}
.three {
color : rgb ( 251 , 255 , 120 );
}
.four {
color : rgb ( 255 , 255 , 0 );
}
.five {
color : rgb ( 24 , 159 , 14 );
}
|
Output:
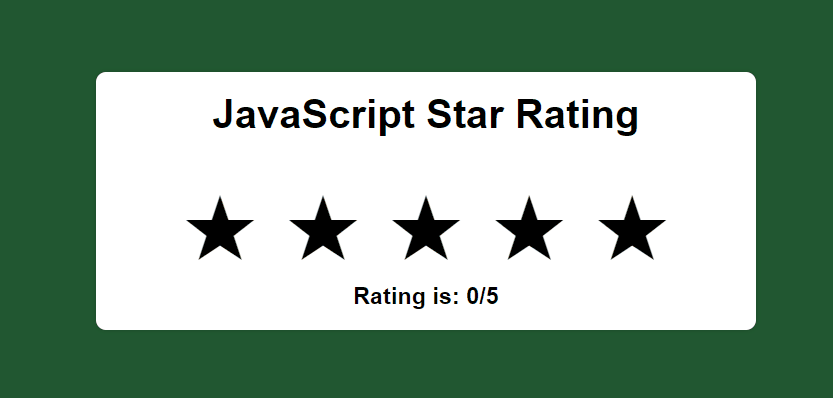
Share your thoughts in the comments
Please Login to comment...