Send Email Using yagmail in Python
Last Updated :
07 Oct, 2021
In this article, we are going to see how to send email using yagmail. yagmail(Yet Another Gmail) is a module in python which used to send emails using Python. This module is nothing, just a Gmail/SMTP(Simple Mail Transfer Protocol) client that removes the issues of sending emails through Python. This helps the Web App to interact with Gmail without any issues.
Note: This makes Gmail accounts vulnerable to some unauthorized access, so to impose security in Gmail accounts, use OAuth2 credentials to get access rights.
Installation:
pip install yagmail
Register User Email ID:
By registering, we allow the yagmail to access our Gmail account in consent to send emails. There is a need for an SMTP client to provide the authentication to the client for sending an email.
yagmail.register(“Sender’s Gmail Username”, “Sender’s Gmail Password”)
Connect to SMTP server:
To initiate a connection with the SMTP server using the SMTP client, use the command below.
yag = yagmail.SMTP(“Sender@gmail.com”)
Adding Content and Delivering:
- In 1st argument in send() function, pass the receiver’s email address.
- Then in the 2nd one, pass the Subject of the Mail your sender is sending.
- Now in the 3rd one, pass the Content of Mail i.e text or media.
yag.send(“Receiver@gmail.com”,”Subject Of Mail”,”Content(Text, Media etc)”)
Sending simple email:
Python3
import yagmail
yag = yagmail.SMTP( "Sender's Email Address" ,
"Sender's Email Address Password" )
yag.send( "Receiver's Email Address" ,
"Subject of Email to be send" ,
"Content(Text,Media, etc. files) of Email to be send" )
|
Sending Email With Multiple Attachments
Here we will send an email with multiple attachments. In attachments, attributes pass through the list of attachments that have to be sent to the receiver.
Syntax: yag.send(“Receiver@gmail.com”,”Subject Of Mail”,”Content Of Mail”, attachments= [‘Attachment1.png’,’ Attachment2.png’,’ Attachment3.png’])
Code:
Python3
import yagmail
yag = yagmail.SMTP( "Sender's Email Address" ,
"Sender's Email Address Password" )
yag.send( "Receiver@gmail.com" , "Subject Of Mail" , "Content Of Mail" ,
attachments = [ 'Attachment1.png' , 'Attachment2.png' , 'Attachment3.png' ])
|
Output:
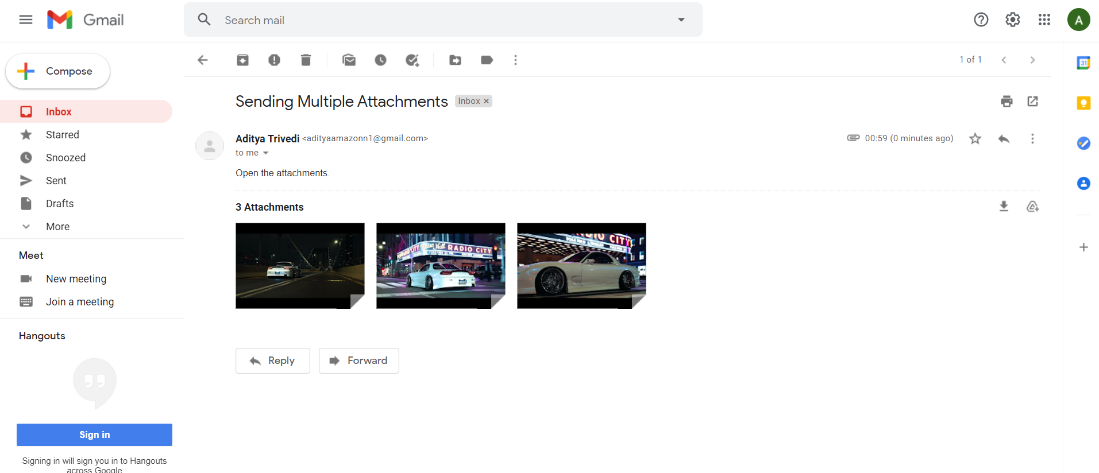
Sending Emails to Multiple Recipients
In “to” argument in send() function, pass the list of multiple receivers email addresses.
Syntax: yag.send(to=[“recipient1@gmail.com”,”recipient2@gmail.com”,”recipient3@gmail.com”], “Subject Of Mail”,”Content Of Mail”)
Code:
Python3
import yagmail
yag = yagmail.SMTP( "Sender's Email Address" ,
"Sender's Email Address Password" )
yag.send(to = [ "recipient1@gmail.com" , "recipient2@gmail.com" ,
"recipient3@gmail.com" ], "Subject Of Mail" , "Content Of Mail" )
|
Output:
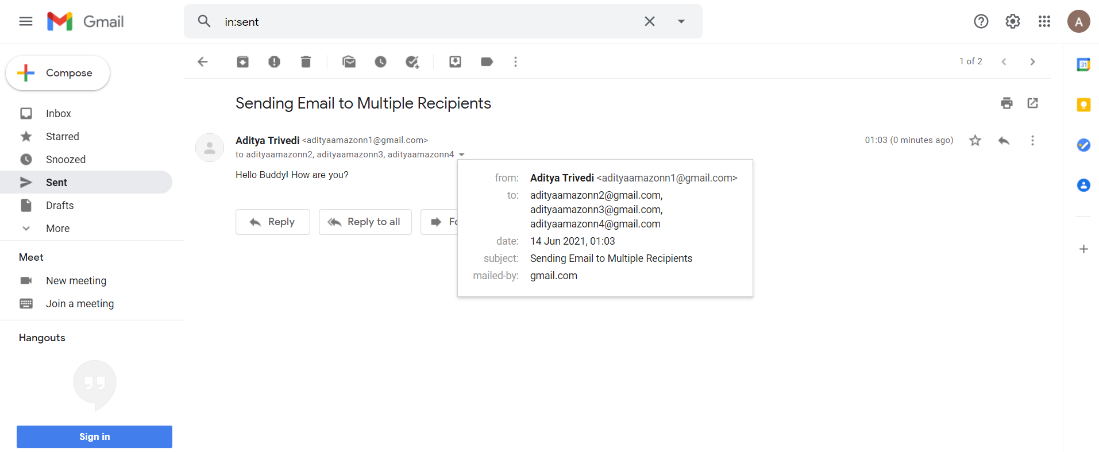
Sending Emails with CC and BCC Fields
In cc(carbon copy) pass the receiver2 email address and in the 3rd one i.e in bcc(blind carbon copy) pass the receiver3 email address..
Syntax: yag.send(to=”Receiver1@gmail.com”,cc=”Receiver2@gmail.com”, bcc=”Receiver1@gmail.com”,”Subject Of Mail”,”Content Of Mail”)
Code:
Python3
import yagmail
yag = yagmail.SMTP( "Sender's Email Address" ,
"Sender's Email Address Password" )
yag.send(to = "Receiver1@gmail.com" , cc = "Receiver2@gmail.com" ,
bcc = "Receiver1@gmail.com" , "Subject Of Mail" , "Content Of Mail" )
|
Output:
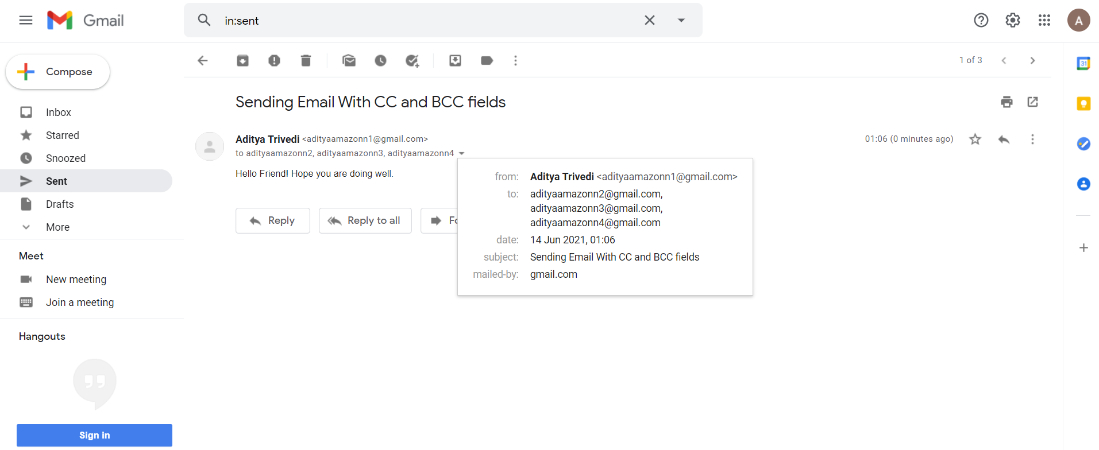
Send an HTML Email
Pass the Content of Mail inside HTML tags. So, the message will be formatted following the HTML syntax that you have given.
Syntax: yag.send(“Receiver@gmail.com”,”Subject Of Mail”,”<h2>Content Of Mail</h2>”)
Code:
Python3
import yagmail
yag = yagmail.SMTP( "Sender's Email Address" ,
"Sender's Email Address Password" )
yag.send( "Receiver@gmail.com" , "Subject Of Mail" ,
"<h2>Content Of Mail</h2>" )
|
Output:
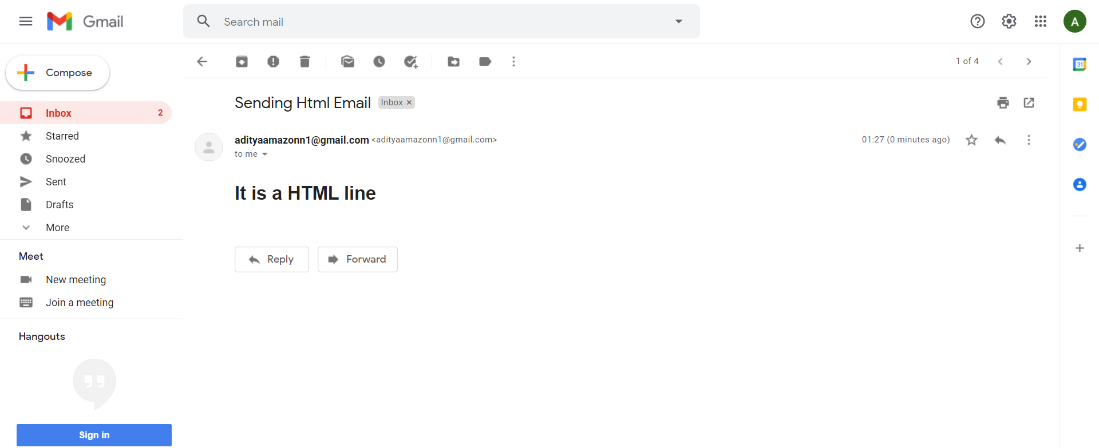
Share your thoughts in the comments
Please Login to comment...