Return Data in JSON Format Using FastAPI in Python
Last Updated :
26 Feb, 2024
FastAPI is a modern, fast, web framework for building APIs with Python 3.7+ based on standard Python type hints. It is designed to be easy to use and efficient, providing automatic generation of OpenAPI and JSON Schema documentation. In this article, we will see how to return data in JSON format using FastAPI in Python.
How to Return Data in Json Format Using Fastapi in Python
Below is the step-by-step procedure by which we can return data in JSON format using FastAPI in Python:
Step 1: Installation
Here, we will install the following libraries and modules before starting.
- Install Python
- Install FastAPI
- Install uvicorn
Step 2: Create a Virtual Environment
In this step, we will create the virtual environment in Python. To create a virtual environment, write the following command in your terminal.
python3 -m venv venv
Now, activate the virtual environment by using the following command:
venv\Scripts\activate
Now run a command to create and automatically write down dependency in requirements.txt
pip freeze > requirements.txt
Step 3. Write the FastAPI Python Code
In this code, a FastAPI application is created with a single endpoint (“/Hello-world”). When a GET request is made to this endpoint, the Hello
function is executed, returning a JSON response containing a nested structure with a “message,” “details,” and information about the author (name and email), along with a list of tags. This demonstrates how FastAPI effortlessly handles JSON serialization, allowing developers to return complex data structures as API responses.
main.py
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/Hello-world" )
def Hello():
data = {
"message" : "hello world" ,
"details" : {
"author" : {
"name" : "Tushar" ,
"email" : "tushar@example.com"
},
"tags" : [ "fastapi" , "python" , "web" ]
}
}
return data
|
Step 4: Run the Program
Now run the file using command in terminal.
uvicorn main:app --reload
After that you will something like image below in terminal

Now copy the following command and paste it to the browser to run the following program:
http://127.0.0.1:8000/Hello-world
This is basically says that your server is running at localhost:8000 ,this is the default port at which fastapi server running locally. But it will take you to the home route which we have not defined so it will show this thing.

It is just showing the default error handling you can go to your route and see that it is working. just enter this in tab localhost:8000/Hello-world.
Output:
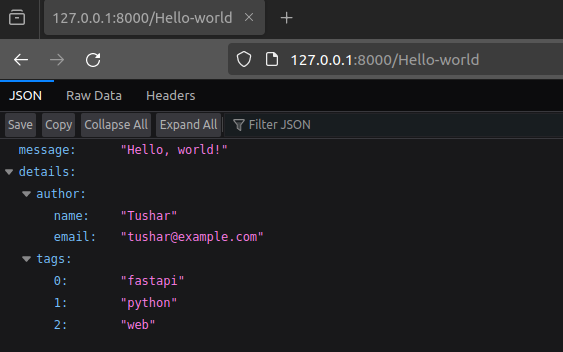
JSON Data Returned Using FastAPI
Video Demonstration
Share your thoughts in the comments
Please Login to comment...