Remove all consecutive duplicates from the string
Last Updated :
22 Aug, 2023
Given a string S, The task is to remove all the consecutive duplicate characters of the string and return the resultant string.
Note: that this problem is different from Recursively remove all adjacent duplicates. Here we keep one character and remove all subsequent same characters.
Examples:
Input: S= “aaaaabbbbbb”
Output: ab
Input: S = “geeksforgeeks”
Output: geksforgeks
Input: S = “aabccba”
Output: abcba
Remove all consecutive duplicates from the string using sliding window:
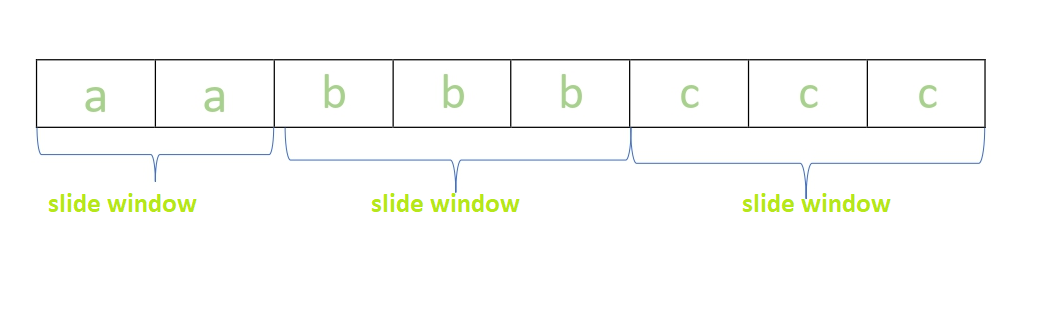
Image Contributed by SR.Dhanush
Approach:
1) Input String S.
2) Initialize two pointer i, j and empty string new_elements.
3) Traverse the String Using j.
4) Compare the elements s[i] and s[j].
(i) if both elements are same skip .
(ii) if both elements are not same then append into new_elements set and slide over the window.
5) After Traversing return result.
C++14
#include <iostream>
#include <string>
using namespace std;
string deleteConsecutiveStrings(string s)
{
int i = 0;
int j = 0;
string newElements = "" ;
while (j < s.length()) {
if (s[i] == s[j]) {
j++;
}
else if (s[j] != s[i] || j == s.length() - 1) {
newElements += s[i];
i = j;
j++;
}
}
newElements += s[j - 1];
return newElements;
}
int main()
{
string s = "geeks for geeks is best" ;
cout << "Input : " << s << endl;
cout << "Output : " << deleteConsecutiveStrings(s) << endl;
return 0;
}
|
Java
public class ConsecutiveStrings {
public static String deleteConsecutiveStrings(String s)
{
int i = 0 ;
int j = 0 ;
String newElements = "" ;
while (j < s.length()) {
if (s.charAt(i) == s.charAt(j)) {
j++;
}
else if (s.charAt(j) != s.charAt(i)
|| j == s.length() - 1 ) {
newElements += s.charAt(i);
i = j;
j++;
}
}
newElements += s.charAt(j - 1 );
return newElements;
}
public static void main(String[] args)
{
String s = "geeks for geeks is best" ;
System.out.println( "Input : " + s);
System.out.println( "Output : "
+ deleteConsecutiveStrings(s));
}
}
|
Python3
def delete_consecutive_strings(s):
i = 0
j = 0
new_elements = ''
while (j< len (s)):
if ( s[i] = = s[j] ):
j + = 1
elif ((s[j]! = s[i]) or (j = = len (s) - 1 )):
new_elements + = s[i]
i = j
j + = 1
new_elements + = s[j - 1 ]
return new_elements
s = 'geeks for geeks is best'
print ( 'Input :' , s)
print ( 'Output :' ,delete_consecutive_strings(s))
|
C#
using System;
public class Gfg
{
public static string deleteConsecutiveStrings( string s)
{
int i = 0;
int j = 0;
string newElements = "" ;
while (j < s.Length)
{
if (s[i] == s[j])
{
j++;
}
else if (s[j] != s[i] || j == s.Length - 1)
{
newElements += s[i];
i = j;
j++;
}
}
newElements += s[j - 1];
return newElements;
}
public static void Main()
{
string s = "geeks for geeks is best" ;
Console.WriteLine( "Input : " + s);
Console.WriteLine( "Output : " + deleteConsecutiveStrings(s));
}
}
|
Javascript
function delete_consecutive_strings(s) {
let i = 0;
let j = 0;
let new_elements = '' ;
while (j < s.length) {
if (s[i] == s[j]) {
j++;
} else if ((s[j] != s[i]) || (j == s.length - 1)) {
new_elements += s[i];
i = j;
j++;
}
}
new_elements += s[j - 1];
return new_elements;
}
let s = 'geeks for geeks is best' ;
console.log( 'Input :' , s);
console.log( 'Output :' , delete_consecutive_strings(s));
|
Output
Input : geeks for geeks is best
Output : geks for geks is best
Time Complexity: O(N)
Auxiliary Space: O(1)
Remove all consecutive duplicates from the string using recursion:
Approach:
If the string is not empty compare the adjacent characters of the string. If they are the same then shift the characters one by one to the left. Call recursion on string S otherwise, call recursion from S+1 string.
Follow the below steps to implement the idea:
- If the string is empty, return.
- Else compare the adjacent characters of the string. If they are the same then shift the characters one by one to the left. Call recursion on string S
- If they are not same then call recursion from S+1 string.
Illustration:
The recursion tree for the string S = aabcca is shown below.
aabcca S = aabcca
/
abcca S = abcca
/
bcca S = abcca
/
cca S = abcca
/
ca S = abca
/
a S = abca (Output String)
/
empty string
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void removeDuplicates( char * S)
{
if (S[0] == '\0' )
return ;
if (S[0] == S[1]) {
int i = 0;
while (S[i] != '\0' ) {
S[i] = S[i + 1];
i++;
}
removeDuplicates(S);
}
removeDuplicates(S + 1);
}
int main()
{
char S1[] = "geeksforgeeks" ;
removeDuplicates(S1);
cout << S1 << endl;
char S2[] = "aabcca" ;
removeDuplicates(S2);
cout << S2 << endl;
return 0;
}
|
Java
import java.io.*;
class GFG {
public static String
removeConsecutiveDuplicates(String input)
{
if (input.length() <= 1 )
return input;
if (input.charAt( 0 ) == input.charAt( 1 ))
return removeConsecutiveDuplicates(
input.substring( 1 ));
else
return input.charAt( 0 )
+ removeConsecutiveDuplicates(
input.substring( 1 ));
}
public static void main(String[] args)
{
String S1 = "geeksforgeeks" ;
System.out.println(removeConsecutiveDuplicates(S1));
String S2 = "aabcca" ;
System.out.println(removeConsecutiveDuplicates(S2));
}
}
|
Python3
def removeConsecutiveDuplicates(s):
if len (s) < 2 :
return s
if s[ 0 ] ! = s[ 1 ]:
return s[ 0 ] + removeConsecutiveDuplicates(s[ 1 :])
return removeConsecutiveDuplicates(s[ 1 :])
s1 = 'geeksforgeeks'
print (removeConsecutiveDuplicates(s1))
s2 = 'aabcca'
print (removeConsecutiveDuplicates(s2))
|
C#
using System;
class GFG {
public static string
removeConsecutiveDuplicates( string input)
{
if (input.Length <= 1)
return input;
if (input[0] == input[1])
return removeConsecutiveDuplicates(
input.Substring(1));
else
return input[0]
+ removeConsecutiveDuplicates(
input.Substring(1));
}
public static void Main(String[] args)
{
string S1 = "geeksforgeeks" ;
Console.WriteLine(removeConsecutiveDuplicates(S1));
string S2 = "aabcca" ;
Console.Write(removeConsecutiveDuplicates(S2));
}
}
|
Javascript
<script>
function removeConsecutiveDuplicates(input)
{
if (input.length<=1)
return input;
if (input[0]==input[1])
return removeConsecutiveDuplicates(input.substring(1));
else
return input[0] +
removeConsecutiveDuplicates(input.substring(1));
}
let S1 = "geeksforgeeks" ;
document.write(removeConsecutiveDuplicates(S1)+ "<br>" );
let S2 = "aabcca" ;
document.write(removeConsecutiveDuplicates(S2)+ "<br>" );
</script>
|
Time complexity: O(N2)
Auxiliary Space: O(N)
Remove all consecutive duplicates from the string using Iterative approach:
The idea is to check if current character is equal to the next character or not . If current character is equal to the next character we’ll ignore it and when it is not equal we will add it to our answer. At last add the last character.
Follow the below steps to implement the idea:
- Create an str string to store the result
- Iterate from 0 to string length – 2.
- If the current character is not equal to next character then add it to answer string.
- Else continue.
- return str.
Below is the implementation of above approach:
C++
#include <bits/stdc++.h>
using namespace std;
string removeDuplicates(string s)
{
int n = s.length();
string str = "" ;
if (n == 0)
return str;
for ( int i = 0; i < n - 1; i++) {
if (s[i] != s[i + 1]) {
str += s[i];
}
}
str.push_back(s[n - 1]);
return str;
}
int main()
{
string s1 = "geeksforgeeks" ;
cout << removeDuplicates(s1) << endl;
string s2 = "aabcca" ;
cout << removeDuplicates(s2) << endl;
return 0;
}
|
Java
import java.util.*;
class GFG {
static void removeDuplicates( char [] S)
{
int n = S.length;
if (n < 2 ) {
return ;
}
int j = 0 ;
for ( int i = 1 ; i < n; i++) {
if (S[j] != S[i]) {
j++;
S[j] = S[i];
}
}
System.out.println(Arrays.copyOfRange(S, 0 , j + 1 ));
}
public static void main(String[] args)
{
char S1[] = "geeksforgeeks" .toCharArray();
removeDuplicates(S1);
char S2[] = "aabcca" .toCharArray();
removeDuplicates(S2);
}
}
|
Python3
def removeDuplicates(S):
n = len (S)
if (n < 2 ):
return
j = 0
for i in range (n):
if (S[j] ! = S[i]):
j + = 1
S[j] = S[i]
j + = 1
S = S[:j]
return S
if __name__ = = '__main__' :
S1 = "geeksforgeeks"
S1 = list (S1.rstrip())
S1 = removeDuplicates(S1)
print ( * S1, sep = "")
S2 = "aabcca"
S2 = list (S2.rstrip())
S2 = removeDuplicates(S2)
print ( * S2, sep = "")
|
C#
using System;
class GFG {
static void removeDuplicates( char [] S)
{
int n = S.Length;
if (n < 2) {
return ;
}
int j = 0;
for ( int i = 1; i < n; i++) {
if (S[j] != S[i]) {
j++;
S[j] = S[i];
}
}
char [] A = new char [j + 1];
Array.Copy(S, 0, A, 0, j + 1);
Console.WriteLine(A);
}
public static void Main(String[] args)
{
char [] S1 = "geeksforgeeks" .ToCharArray();
removeDuplicates(S1);
char [] S2 = "aabcca" .ToCharArray();
removeDuplicates(S2);
}
}
|
Javascript
<script>
const removeDuplicates = (s) => {
let n = s.length;
let str = "" ;
if (n == 0)
return str;
for (let i = 0; i < n - 1; i++) {
if (s[i] != s[i + 1]) {
str += s[i];
}
}
str += s[n-1];
return str;
}
let s1 = "geeksforgeeks" ;
document.write(`${removeDuplicates(s1)}<br/>`)
let s2 = "aabcca" ;
document.write(removeDuplicates(s2))
</script>
|
Time Complexity: O(N)
Auxiliary Space: O(1)
Approach#4:using regular expression
Algorithm
1.Import the re module.
2.Use the re.sub() method to replace all consecutive duplicates with a single character.
3.Return the modified string.
C++
#include <iostream>
#include <regex>
using namespace std;
string remove_consecutive_duplicates(string s)
{
regex pattern( "(.)\\1+" );
return regex_replace(s, pattern, "$1" );
}
int main()
{
string s = "geeks for geeks is best" ;
cout << remove_consecutive_duplicates(s) << endl;
return 0;
}
|
Java
import java.util.regex.*;
class Main {
static String removeConsecutiveDuplicates(String s)
{
String pattern = "(.)\\1+" ;
return s.replaceAll(pattern, "$1" );
}
public static void main(String[] args)
{
String s = "geeks for geeks is best" ;
System.out.println(removeConsecutiveDuplicates(s));
}
}
|
Python3
import re
def remove_consecutive_duplicates(s):
return re.sub(r '(.)\1+' , r '\1' , s)
s = 'geeks for geeks is best'
print (remove_consecutive_duplicates(s))
|
C#
using System;
using System.Text.RegularExpressions;
public class GFG {
public static string
RemoveConsecutiveDuplicates( string s)
{
Regex pattern = new Regex( "(.)\\1+" );
return pattern.Replace(s, "$1" );
}
public static void Main( string [] args)
{
string s = "geeks for geeks is best" ;
Console.WriteLine(RemoveConsecutiveDuplicates(s));
}
}
|
Javascript
function removeConsecutiveDuplicates(s) {
const pattern = /(.)\1+/g;
return s.replace(pattern, "$1" );
}
const s = "geeks for geeks is best" ;
console.log(removeConsecutiveDuplicates(s));
|
Output
geks for geks is best
Time complexity: O(n)
Space complexity: O(n)
Share your thoughts in the comments
Please Login to comment...