ReactJS Profilers
Last Updated :
07 Nov, 2023
ReactJS Profilers is a tool for profiling the react components, It measures how many times the react Application is rendered and how much time the components take to be rendered. It helps to identify the parts of the application that are slow so that the developer can optimize it for better performance. It is lightweight and no third-party dependency is required.
The profiler takes two props one is an id which is a String and another one is onRender which is a callback function.
Syntax:
<Profiler id="" onRender={callback function}>
{Children}
</Profiler>
The call-back function also takes a number of arguments:
- id(String): It shows the id that we have given to the profiler while rendering a component, it is unique and helps us track the component.
- phase(String): Shows the phase of the component if it is just rendered for the first time it will show mount or if the value changes or it re-renders it will show update.
- actualDuration(Number): Time is taken to change to the update phase.
- baseDuration(Number): Most recent render time duration
- startTime(Number): When React began rendering the component
- commitTime(Number): The time at which the update has been done
- interactions(Set): A set of instructions
Steps to Create React Application:
Step 1: Initialize the react project folder, using this command in the terminal.
npm create-react-app project
Step 2: After creating your project folder(i.e. project), move to it by using the following command.
cd project
Project Structure:
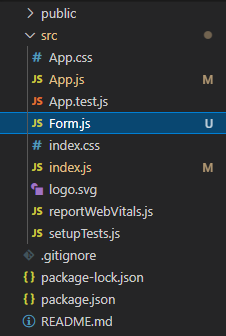
We know that fallback has different properties as well. We are making a form component that takes a string labelname to label in front of the input box.
Note: Using react-hook useState for maintaining the state text.
Example 1: This example implements sinlge profile using the React JS profiler.
Javascript
import { Profiler } from "react" ;
import Form from "./Form" ;
const callback = (
id,
phase,
actualDuration,
startTime,
baseDuration,
commitTime,
interactions
) => {
console.log(
"id " +
id +
" startTime " +
startTime +
" actualDuration " +
actualDuration +
" baseDuration " +
baseDuration +
" commitTime " +
commitTime +
" phase " +
phase +
" interactions " +
interactions
);
};
function App() {
return (
<div className= "App" >
<Profiler id= "Name" onRender={callback}>
<Form labelname= "Name " />
</Profiler>
</div>
);
}
export default App;
|
Javascript
import React, { useState } from "react" ;
const Form = (props) => {
const { labelname, ...rest } = props;
const [text, setText] = useState( "" );
return (
<div>
<label {...rest}>
{labelname} :
<input
type= "text"
value={text}
onChange={(e) =>
setText(e.target.value)
}
/>
</label>
</div>
);
};
export default Form;
|
Steps to run the application: Use this command in the terminal inside the project directory.
npm start
Output: This output will be visible on http://localhost:3000/ on the browser window.
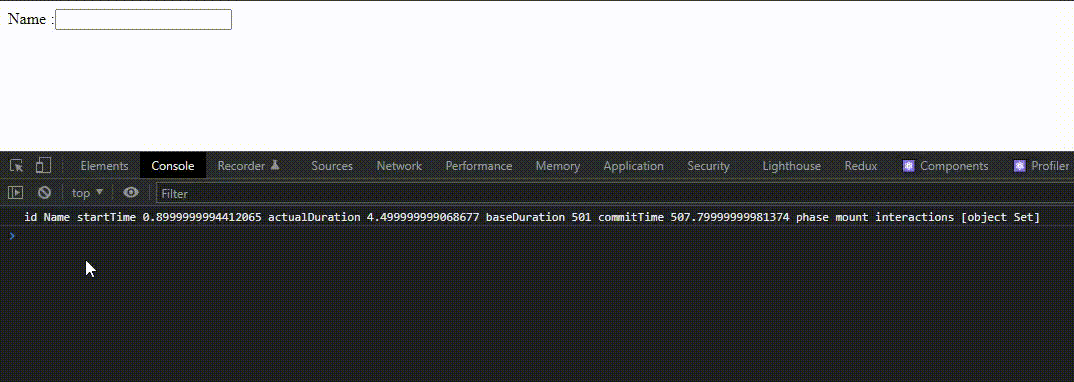
Similarily, We can have a number of profilers in a project, profilers can be even nested to measure different components within the same DOM tree.
Example 2: This example use React JS profiler to implemet multiple profilers.
Javascript
import { Profiler } from "react" ;
import Form from "./Form" ;
const callback = (
id,
phase,
actualDuration,
startTime,
baseDuration,
commitTime,
interactions
) => {
console.log(
"id " +
id +
" startTime " +
startTime +
" actualDuration " +
actualDuration +
" baseDuration " +
baseDuration +
" commitTime " +
commitTime +
" phase " +
phase +
" interactions " +
interactions
);
};
function App() {
return (
<div className= "App" >
<Profiler id= "Name" onRender={callback}>
<Form labelname= "Name " />
<Profiler id= "Number" onRender={callback}>
<Form labelname= "Number" />
</Profiler>
</Profiler>
</div>
);
}
export default App;
|
Javascript
import React, { useState } from "react" ;
const Form = (props) => {
const { labelname, ...rest } = props;
const [text, setText] = useState( "" );
return (
<div>
<label {...rest}>
{labelname} :
<input
type= "text"
value={text}
onChange={(e) =>
setText(e.target.value)
}
/>
</label>
</div>
);
};
export default Form;
|
Steps to run the application: Use this command in the terminal inside the project directory.
npm start
Output: This output will be visible on http://localhost:3000/ on the browser window.
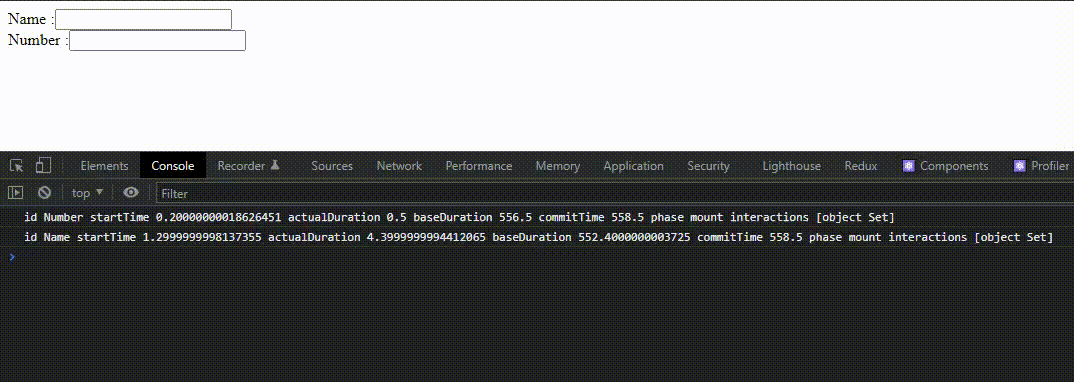
Share your thoughts in the comments
Please Login to comment...