BrowserRouter in React
Last Updated :
12 Mar, 2024
React Router is a powerful library for handling routing in React applications. BrowserRouter is a type of router provided by React Router that uses HTML5 history API to keep UI in sync with the URL. It enables declarative routing in React applications by mapping specific routes to components. In this article, we will explore BrowserRouter in React Router in depth. We’ll cover its features, advantages, and how to use it effectively in React applications.
What is BrowserRouter in React Router?
The BrowserRouter
component utilizes the HTML5 history API (pushState
, replaceState
, and the popstate
event) to keep the UI in sync with the URL in the browser address bar without page reloads. It provides a declarative way to manage routing in React applications by mapping certain components to specific routes.
basename: string
The “basename” property is a string representing the base URL for all locations within your application. When your application is served from a sub-directory on the server, it’s essential to define this property with the sub-directory path. A correctly formatted basename should commence with a leading slash but should not conclude with a trailing slash.
getUserConfirmation: func
The “getUserConfirmation” property is a function utilized to confirm navigation actions within the application. By default, it relies on the standard browser window.confirm method. However, you can customize this behavior by providing your function.
This function is invoked whenever the user initiates a navigation action, such as clicking on a link or attempting to navigate away from the current page. It prompts the user to confirm their intention before proceeding with the navigation action.
forceRefresh: bool
When set to true, the “forceRefresh” property instructs the router to utilize full page refreshes during page navigation. This functionality can be useful for replicating the behavior of traditional server-rendered applications, where full page refreshes occur between each navigation action. By enabling this option, you simulate the experience of a server-rendered app, providing a more familiar navigation flow for users accustomed to such behavior.
keyLength: number
The “keyLength” property specifies the length of the location key used internally by the router. By default, this value is set to 6 characters. Adjusting this property allows you to control the length of the keys generated for locations within the application.
children: node
The “children” property expects child elements to be rendered within the component. This property is typically used to define the content or components that will be nested inside the parent component.
Key Features:
- Declarative routing: Define routes using JSX syntax.
- Uses HTML5 history API: Allows navigation without full page refresh.
- Handles dynamic and nested routes efficiently.
- Provides access to route information via Route, Switch, and Link components.
- Supports server-side rendering.
Steps to Implement BrowserRouter in React Router :
Let’s create a simple React application with BrowserRouter:
Step 1: Install React Router
npm install react-router-dom
Step 2: Set up BrowserRouter in your application.
Javascript//Home.js
import React from 'react'
import { useNavigate } from 'react-router-dom'
const Home = () => {
const navigate=useNavigate()
const goabout=()=>{
navigate('/about')
}
return (
<div>
<h3>Welcome to home page</h3>
<button onClick={goabout}>Go to About page</button>
</div>
)
}
export default Home
Javascript//About.js
import React from 'react'
import { useNavigate } from 'react-router-dom'
const About = () => {
const navigate=useNavigate()
const gohome=()=>{
navigate(-1)
}
return (
<div>
<h3>Welcome to about us page</h3>
<button onClick={gohome}>Go to Home Page</button>
</div>
)
}
export default About
Javascript//UserDetail.js
import React from 'react'
import { useParams } from 'react-router-dom'
const UserDetail = () => {
const{userId}=useParams();
return (
<div>
<h1>User Details:</h1>
<p>User information is:{userId}</p>
</div>
)
}
export default UserDetail
Javascript//Userprofile.js
import React from 'react'
import { useNavigate } from 'react-router-dom'
const UserProfile = () => {
const navigate=useNavigate();
const logout=()=>{
navigate('/login')
}
return (
<div>
<h1>User Profile</h1>
<button onClick={logout}>Logout</button>
</div>
)
}
export default UserProfile
Javascript//useNavigation with useParams...
import React from 'react'
import { BrowserRouter as Router,Routes,Route ,Link} from 'react-router-dom'
import Home from './components/useNavigate/Home'
import About from './components/useNavigate/About'
import UserDetail from './components/useNavigate/UserDetail'
import UserProfile from './components/useNavigate/UserProfile'
const App = () => {
return (
<div>
<Router>
<nav>
<ul style={{display:'flex',gap:'20px'}}>
<li><Link to='/'>Home</Link></li>
<li><Link to='/about'>About</Link></li>
<li><Link to='/user/123'>User Details</Link></li>
<li><Link to='/profile'>User Profile</Link></li>
</ul>
</nav>
<Routes>
<Route path='/' element={<Home />}/>
<Route path='/about' element={<About />}/>
<Route path='/user/:userId' element={<UserDetail />}/>
<Route path='/profile' element={<UserProfile />}/>
</Routes>
</Router>
</div>
)
}
export default App
Output:
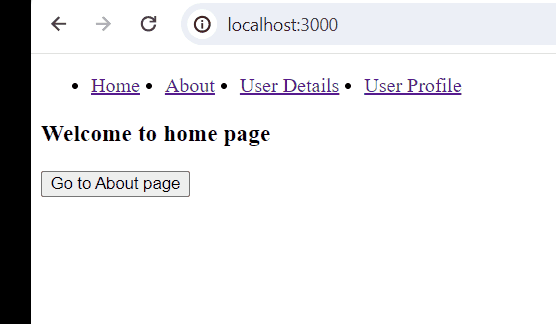
Output
Share your thoughts in the comments
Please Login to comment...