Python Pyramid – Static Assets
Last Updated :
12 Dec, 2023
A lightweight web framework in Python that converts small web apps to large web apps is called Pyramid. There are various circumstances when the user needs to add some images, HTML code, CSS code, etc. along with the Python code in the web app. These are called static assets and can be added using the Pyramid in Python.
Python Pyramid – Static Assets
Syntax: config.add_static_view(name=’static’,path=’static’)
Parameters:
- name=’static’ : name or route prefix for the static view
- path=’static’ : directory on the server where the static files are stored
The ‘static’ directory is used to store the static assets. There are three static assets in Python Pyramid:
Static Asset: Image
The visual representation of something is called an image. It is very crucial to add images to the app to make it attractive. In this way, we will see how we can add static assets, and images to the web app using Pyramid.
app2.py
In this example, we created a Python file app2.py under the package pyramid1. We have defined a constructor in the app in which we have added the static view.
Python3
from wsgiref.simple_server import make_server
from pyramid.config import Configurator
from pyramid.view import view_config
@view_config (
route_name = 'hello' ,
renderer = "static/templates/home.jinja2"
)
def home(request):
return { 'greet' : 'Welcome to Geeks For Geeks!' }
if __name__ = = "__main__" :
with Configurator() as config:
config.include( 'pyramid_jinja2' )
config.add_static_view(name = 'static' ,path = 'static' )
config.add_route( 'hello' , '/' )
config.scan()
app = config.make_wsgi_app()
server = make_server( '0.0.0.0' , 6543 ,app)
server.serve_forever()
|
home.jinja2
Now, we have created a folder templates under static. In this templates folder, we have created the home.jinja2 file. In this file, we have defined the static asset image that we want to display on our web app.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >{{greet}}</ title >
</ head >
< body >
< h1 > {{greet}}</ h1 >
< img src = "static/geeksforgeeks.jpg" style = "width: 570px;" >
</ body >
</ html >
|
geeksforgeeks.png
Here, we have used the Geeks For Geeks logo (link) as an image, which we have placed in the static folder. Thus, the package will look similar to this:
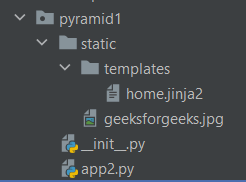
Output:
Now, run the following command in the terminal to run the app.
python app2.py
For seeing the webapp, open the browser of your choice, and type the URL, http://localhost:6543/
-660.png)
Static Asset: CSS File
The way of displaying HTML elements in known as CSS. It is very crucial to add CSS in web app for making it attractive. In this way, we will see how we can add static asset: CSS to the web app using Pyramid.
app2.py
In this example, we created a Python file app2.py under the package pyramid1. We have defined a constructor in the app in which we have added the static view.
Python3
from wsgiref.simple_server import make_server
from pyramid.config import Configurator
from pyramid.view import view_config
@view_config (
route_name = 'hello' ,
renderer = "static/templates/home.jinja2"
)
def home(request):
return { 'greet' : 'Welcome to Geeks For Geeks!' }
if __name__ = = "__main__" :
with Configurator() as config:
config.include( 'pyramid_jinja2' )
config.add_static_view(name = 'static' ,path = 'static' )
config.add_route( 'hello' , '/' )
config.scan()
app = config.make_wsgi_app()
server = make_server( '0.0.0.0' , 6543 ,app)
server.serve_forever()
|
home.jinja2
Now, we have created a folder templates under static. In this templates folder, we have created the home.jinja2 file. In this file, we have defined the static asset CSS that we want to display on our web app.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >{{greet}}</ title >
< link rel = "stylesheet" href = "static/style1.css" />
</ head >
< body >
< h1 > {{greet}}</ h1 >
</ body >
</ html >
|
style1.css
Here, we have created the CSS file style1.css in the static folder.
CSS
*{
margin : 0 ;
padding : 0 ;
box:sizing:border-box;
background-color : green ;
color : red ;
font-family : sans -ser;
}
|
The package will look similar to this:
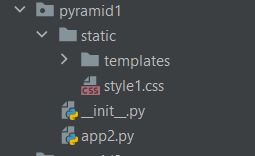
Output:
Now, run the following command in the terminal to run the app.
python app2.py
For seeing the webapp, open the browser of your choice, and type the URL, http://localhost:6543/
-660.png)
Static Asset: Javascript File
Javascipt is the most popular light-weighted programming language. It helps in making the web app reponsive. In this way, we will see how we can add static asset: javascript to the web app using Pyramid.
app2.py
In this example, we created a Python file app2.py under the package pyramid1. We have defined a constructor in the app in which we have added the static view.
Python3
from wsgiref.simple_server import make_server
from pyramid.config import Configurator
from pyramid.view import view_config
@view_config (
route_name = 'hello' ,
renderer = "static/templates/home.jinja2"
)
def home(request):
return { 'greet' : 'Welcome to Geeks For Geeks!' }
if __name__ = = "__main__" :
with Configurator() as config:
config.include( 'pyramid_jinja2' )
config.add_static_view(name = 'static' ,path = 'static' )
config.add_route( 'hello' , '/' )
config.scan()
app = config.make_wsgi_app()
server = make_server( '0.0.0.0' , 6543 ,app)
server.serve_forever()
|
home.jinja2
Now, we have created a folder templates under static. In this templates folder, we have created the home.jinja2 file. In this file, we have defined the static asset Javscript that we want to display on our web app.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >{{greet}}</ title >
< script type = "text/javascript" src = "static/function.js" ></ script >
</ head >
< body >
< h1 > {{greet}}</ h1 >
< input type = "button" onclick = "myfunction()" value = "Display" >
</ body >
</ html >
|
function.js
Here, we have created the Javascript file function.js in the static folder.
Javascript
function myfunction()
{
document.write( "Hi, I am here!" );
}
|
The package will look similar to this:
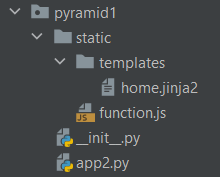
Output:
Now, run the following command in the terminal to run the app.
python app2.py
For seeing the webapp, open the browser of your choice, and type the URL, http://localhost:6543/
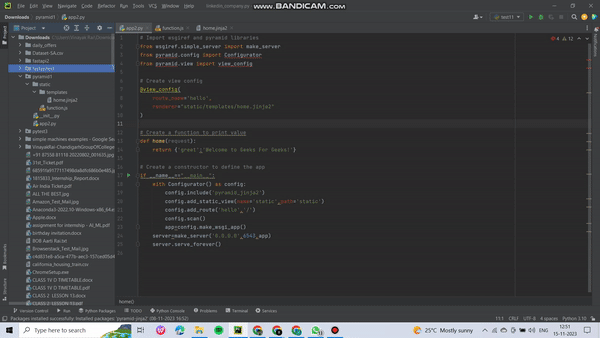
Conclusion
The static assets are used to make the web-app look attractive and reponsive. I hope after reading the above article, you will able to use the static assets in your Pyramid web app.
Share your thoughts in the comments
Please Login to comment...