Python Pyramid – View Configuration
Last Updated :
20 Mar, 2024
In Python Pyramid, configuring views is a fundamental aspect of developing web applications. Views are where the business logic of your application resides, handling incoming requests and generating responses to clients. Pyramid provides several ways to configure views, including the add_view()
method, @view_config()
decorator, and @view_defaults()
decorator. In this article, we will learn about Python Pyramid view configuration.
Python Pyramid – View Configuration
Below, are the Python Pyramid – View Configuration methods with code examples in Python:
- add_view() Method
- @view_config() Decorator
- @view_defaults() Decorator
Python Pyramid View Configuration add_view() Method
The add_view()
method is a part of the Pyramid configuration system. It allows you to register a view callable with the Pyramid framework, specifying various configuration options such as route patterns, request methods, predicates, and more. Here’s a basic example of how to use add_view()
.
In this example, below Python Pyramid code sets up two view functions, home and about, each returning a response for the homepage and about page, respectively. The Configurator defines routes for these pages (‘/’ and ‘/about’) and associates them with their corresponding view functions using add_route() and add_view().
Python3
from pyramid.config import Configurator
from wsgiref.simple_server import make_server
from pyramid.response import Response
def home(request):
return Response('Welcome to the homepage!')
def about(request):
return Response('Learn more about us here.')
if __name__ == '__main__':
with Configurator() as config:
config.add_route('home', '/')
config.add_route('about', '/about')
config.add_view(home, route_name='home')
config.add_view(about, route_name='about')
app = config.make_wsgi_app()
server = make_server('0.0.0.0', 6543, app)
print("Running on http://localhost:6543")
server.serve_forever()
Output:
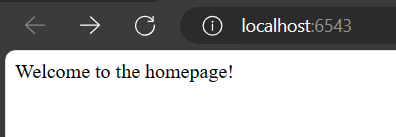
home page
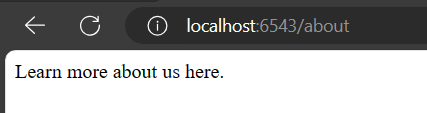
about page
Python Pyramid View Configuration @view_config() Decorator
The @view_config()
decorator provides a declarative way to configure views directly on the view callable itself. This approach is more concise and convenient than using add_view()
. Here’s how you can use @view_config()
:
In this example, below Python Pyramid code defines two view functions, home and about, using the @view_config decorator to associate each with its respective route (‘home’ and ‘about’). The Configurator sets up routes for these pages (‘/’ and ‘/about’) using add_route(), and scan() automatically discovers and configures views.
Python3
from pyramid.config import Configurator
from pyramid.response import Response
from pyramid.view import view_config
@view_config(route_name='home')
def home(request):
return Response('Welcome to the homepage!')
@view_config(route_name='about')
def about(request):
return Response('Learn more about us here.')
if __name__ == '__main__':
with Configurator() as config:
config.add_route('home', '/')
config.add_route('about', '/about')
config.scan() # Automatically discover views
app = config.make_wsgi_app()
from wsgiref.simple_server import make_server
server = make_server('0.0.0.0', 6543, app)
print("Running on http://localhost:6543")
server.serve_forever()
Output:
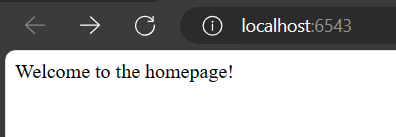
home page
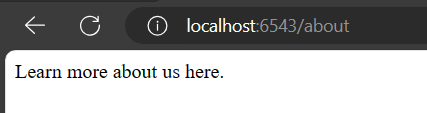
about page
Python Pyramid View Configuration @view_defaults() Decorator
@view_defaults() decorator enables specifying default view configuration options for all view callables within a class, which is especially beneficial for related views with shared configuration settings. This decorator streamlines the process of defining common configurations for multiple views within a class.
In this example, below Python Pyramid code defines a class InfoViews with @view_defaults() decorator, setting the default route name to ‘info’. Within the class, two methods, get_info() and post_info(), are configured with @view_config(), specifying GET and POST request methods respectively, each returning a Response.
Python3
from pyramid.config import Configurator
from pyramid.response import Response
from pyramid.view import view_config, view_defaults
@view_defaults(route_name='info')
class InfoViews:
def __init__(self, request):
self.request = request
@view_config(request_method='GET')
def get_info(self):
return Response('Get information')
@view_config(request_method='POST')
def post_info(self):
return Response('Post information')
if __name__ == '__main__':
with Configurator() as config:
# Add this line if you want to use Chameleon templates
config.include('pyramid_chameleon')
config.add_route('info', '/info')
config.scan()
app = config.make_wsgi_app()
from wsgiref.simple_server import make_server
server = make_server('0.0.0.0', 6543, app)
print("Running on http://localhost:6543")
server.serve_forever()
Output:
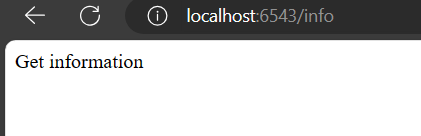
Share your thoughts in the comments
Please Login to comment...