Python Pyramid – Message Flashing
Last Updated :
15 Mar, 2024
In this article, we will explore the concept of Python flashing through a practical example. We’ll be creating a registration page where, upon entering the correct information, a success message will be displayed. Conversely, if there are errors in the provided details, an error message will be shown on the display screen.
What is Python Pyramid – Message Flashing?
In Python Pyramid, message flashing is a technique for temporarily storing and displaying messages across different web requests. It’s useful for providing feedback to users after actions like form submissions. The framework’s `pyramid_flash_messages` module facilitates this by allowing messages to be stored in the session and retrieved in subsequent requests. Typically, a flash message is set, the user is redirected to another page, and the message is displayed there.
Create Python Pyramid – Message Flashing
To implement Message Flashing in Python using the Pyramid framework, adhere to the following steps:
Step 1: Create a Virtual Environment
Create a virtual environment using the following commands:
pip install virtualenv
python -m venv env
Activate the virtual environment using the command:
.\env\Scripts\activate
After activating the Virtual Environment execute the below command to install all the dependencies.
pip install -r requirements.txt
Below are the list of packages, it should be placed inside the requirements.txt file.
pyramid
pyramid_jinja2
flash
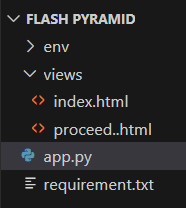
Step 2: Implement the Logic
app.py : In this example the Python code uses the Pyramid framework to create a web application handling user registration. The `registration` view function validates a form with email and mobile number fields using regular expressions. Successful submissions store user data securely in a session, while validation failures trigger error messages. The application is configured with Jinja2 for rendering and launched on a development server at ‘0.0.0.0:6543’. Overall, it showcases a concise implementation of web registration functionality with Pyramid.
Python
from wsgiref.simple_server import make_server
from pyramid.config import Configurator
from pyramid.renderers import render_to_response
from pyramid.view import view_config
from pyramid.session import SignedCookieSessionFactory
import re
@view_config(route_name="register", renderer="index.html")
def registration(request):
if request.method == "POST":
name = request.POST["name"]
email = request.POST["email"]
mobile = request.POST["mobile"]
# For Pattern Matching, Input Validation
emailPattern = re.compile(r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$")
mobilePattern = re.compile(r"^[0-9]{10}$")
if name != "" and emailPattern.match(email) and mobilePattern.match(mobile):
request.session["name"] = request.POST["name"]
request.session["email"] = request.POST["email"]
# For Flash Message
request.session.flash("Success!")
return render_to_response(
"./views/proceed.html", {"error": "Success!"}, request=request
)
else:
# For Flash Message
request.session.flash("Please fill in all fields properly!")
return render_to_response(
"./views/index.html",
{"error": "Please fill in all fields properly!"},
request=request,
)
else:
return render_to_response("./views/index.html", {}, request=request)
@view_config(route_name="proceed", renderer="proceed.html")
def proceed(request):
return render_to_response("./views/proceed.html", {}, request=request)
my_session = SignedCookieSessionFactory("mySession")
if __name__ == "__main__":
with Configurator() as config:
# Initialize Session Factory
config.set_session_factory(my_session)
# Add Jinja2 Template Renderer
config.include("pyramid_jinja2")
config.add_jinja2_renderer(".html")
# Add Routes and Views
config.add_route("register", "/")
config.add_view(registration, route_name="register")
config.add_route("proceed", "/proceed.html")
config.add_view(proceed, route_name="proceed")
app = config.make_wsgi_app()
server = make_server("0.0.0.0", 6543, app)
server.serve_forever()
Step 3: Creating GUI
./views/index.html : In below code the HTML code creates a registration form webpage using Pyramid and Jinja2 templating. The form includes fields for name, email, and mobile number, styled with Bootstrap 5.0.2. Upon submission, data is sent via the “post” method. The page displays server-side flashed messages in a responsive layout, enhancing the user experience.
HTML
<!DOCTYPE html>
{% include package="pyramid_jinja2" %}
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Registration Form</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin="anonymous">
</head>
<body>
<br>
<div>
{% if request.session.peek_flash() %}
<div id="flash" class="container jumbotron">
{% for message in request.session.pop_flash() %}
<center><h1>{{ message }}</h1></center>
{% endfor %}
</div>
{% endif %}
</div>
<br>
<div class="container jumbotron">
<div class="row">
<div class="col-md-6 col-md-offset-3 form-container">
<h2>Registration Form</h2>
<p>Fill up the form with correct values.</p>
<form role="form" method="post" id="reused_form" action="">
<div class="row">
<div class="col-sm-12 form-group">
<label for="name">Name:</label>
<input type="text" class="form-control" id="name" name="name" maxlength="50">
</div>
</div>
<div class="row">
<div class="col-sm-12 form-group">
<label for="email">Email:</label>
<input type="email" class="form-control" id="email" name="email" maxlength="50">
</div>
</div>
<div class="row">
<div class="col-sm-12 form-group">
<label for="mobile">Mobile No.:</label>
<input type="tel" class="form-control" id="mobile" name="mobile" maxlength="50">
</div>
</div>
<br>
<div class="row">
<div class="col-sm-12 form-group">
<center>
<button type="submit" class="btn btn-lg btn-success btn-block" id="btnContactUs">Register</button>
</center>
</div>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
./views/proceed.html : This HTML code defines a webpage with a registration form. It includes the Pyramid framework’s Jinja2 package for template rendering. The page displays flash messages (temporary messages) stored in the session. If there are any flash messages, it creates a jumbotron container to show each message with a “Back” button linking to the home page.
HTML
<!DOCTYPE html>
{% include package="pyramid_jinja2" %}
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Registration Form</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin="anonymous">
</head>
<body>
<br>
<div>
{% if request.session.peek_flash() %}
<div id="flash" class="container jumbotron">
{% for message in request.session.pop_flash() %}
<center><h1>{{ message }}</h1></center>
{% endfor %}
<center><a href="/"><button type="button" class="btn btn-lg btn-success btn-block"
id="btnContactUs">Back</button></a></center>
</div>
{% endif %}
</div>
</body>
</html>
Step 3: Run the Server
For run the server run below command and open link.
python .\script_name.py
https:\\localhost:6543
Output:
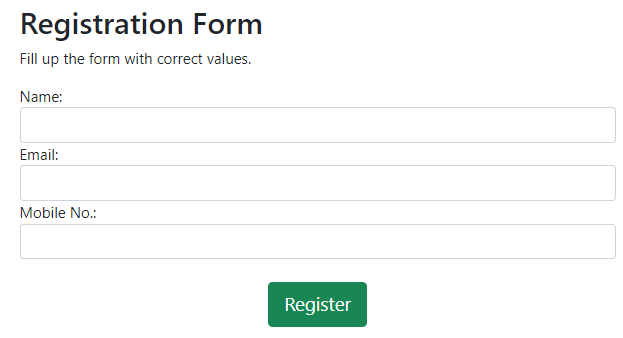
Video Demonstration
Conclusion
In conclusion, Python Pyramid’s message flashing provides a powerful and flexible mechanism for displaying brief informational messages to users, enhancing the overall user experience. By leveraging the pyramid_flash
module, developers can easily incorporate flash messages into their web applications, allowing for seamless communication with users regarding the success or failure of various actions.
Share your thoughts in the comments
Please Login to comment...