Python Falcon – CORS
Last Updated :
19 Mar, 2024
Cross-Origin Resource Sharing (CORS) is an additional security measure implemented by modern browsers to prevent unauthorized requests between different domains. When developing a web API with Falcon, it’s common and recommended to implement a CORS policy to allow cross-origin requests securely. In this article, we will see Python Falcon CORS, its advantages, and also examples.
What is Python Falcon-CORS?
Python Falcon, a minimalist web framework for building APIs, offers the flexibility to implement a CORS policy effortlessly. By default, Falcon’s CORS support is disabled, necessitating explicit configuration to enable it. Leveraging Falcon’s built-in features, developers can enable CORS by simply passing a flag to the application initializer. To import the Python Falcon-CORS use the below command
from falcon_cors import CORS
Advantages
- Simplicity: Falcon – CORS provides a straightforward interface for configuring CORS policies, reducing the complexity of implementation.
- Flexibility: Developers can easily customize CORS policies according to their specific requirements, tailoring access controls for different endpoints.
- Compatibility: As part of the Falcon ecosystem, Falcon-CORS seamlessly integrates with Falcon APIs, ensuring compatibility and optimal performance.
Python Falcon – CORS Examples
Below, are the example of how to use Python Falcon CORS in Python:
- Basic CORS Configuration
- Custom CORS Configuration
- Fine-Tuned CORS Configuration
Configuring CORS in Python Falcon
Basic CORS configuration in Falcon offers a simple approach to enabling cross-origin resource sharing. Below, code demonstrates setting up CORS in a Python Falcon application. It involves creating a Falcon application instance, configuring CORS middleware to allow all origins, defining a Falcon resource with a simple message, adding a route for the resource, and running the application on localhost:8002.
Python3
import falcon
from falcon_cors import CORS
# Create a Falcon application instance
app = falcon.App()
# Configure CORS middleware
cors = CORS(allow_all_origins=True)
app.add_middleware(cors.middleware)
# Define your Falcon resource classes and routes
class MyResource:
def on_get(self, req, resp):
resp.media = {'message': 'Hello, CORS!'}
app.add_route('/hello', MyResource())
# Run the Falcon application
if __name__ == '__main__':
from wsgiref import simple_server
httpd = simple_server.make_server('localhost', 8002, app)
print('Serving on localhost:8002...')
httpd.serve_forever()
Output
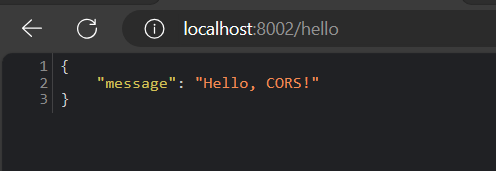
Custom CORS Configuration in Python Falcon
Directly passing the “falcon.CORSMiddleware” middleware to the application allows customization of the CORS policy applied. Below, cdoe uses a customized CORS configuration in a Python Falcon application. It creates a Falcon application instance, configures CORS middleware to allow requests only from ‘http://localhost:3000‘ with all HTTP methods and headers permitted, defines a Falcon resource class that returns a greeting message.
Python3
import falcon
from falcon_cors import CORS
# Create a Falcon application instance
app = falcon.App()
# Configure CORS middleware with custom settings
cors = CORS(
allow_origins_list=['http://localhost:3000'],
allow_all_methods=True,
allow_all_headers=True
)
app.add_middleware(cors.middleware)
# Define a Falcon resource class
class GreetingResource:
def on_get(self, req, resp):
resp.media = {'message': 'Welcome to the Falcon CORS example!'}
# Add a route to the resource
app.add_route('/policy', GreetingResource())
# Run the Falcon application
if __name__ == '__main__':
from wsgiref import simple_server
httpd = simple_server.make_server('localhost', 8000, app)
print('Serving on localhost:8000...')
httpd.serve_forever()
Output
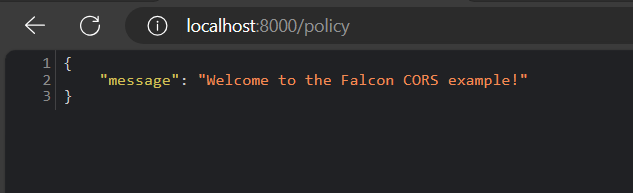
Fine-Tuned CORS Configuration in Python Falcon
Fine-grained CORS configuration in Falcon allows developers to finely tune cross-origin resource sharing policies. Below code shows a finely configured CORS setup in a Python Falcon application. It initializes a Falcon application instance, configures CORS middleware with specific settings such as allowed origins, methods, headers, exposed headers, and allows credentials for all origins. A Falcon resource class is defined to demonstrate a simple example, and a route is added for this resource
Python3
import falcon
from falcon_cors import CORS
# Create a Falcon application instance
app = falcon.App()
# Configure CORS middleware with fine-grained settings
cors = CORS(
allow_origins_list=['http://localhost:3000', 'http://localhost:8080'],
allow_methods_list=['GET', 'POST'],
allow_headers_list=['Content-Type', 'Authorization'],
expose_headers_list=['X-Custom-Header'],
allow_credentials_all_origins=True
)
app.add_middleware(cors.middleware)
# Define a Falcon resource class
class CustomHeaderResource:
def on_get(self, req, resp):
resp.media = {'message': 'Custom header example'}
# Add a route to the resource
app.add_route('/custom-header', CustomHeaderResource())
# Run the Falcon application
if __name__ == '__main__':
from wsgiref import simple_server
httpd = simple_server.make_server('localhost', 8000, app)
print('Serving on localhost:8000...')
httpd.serve_forever()
Output
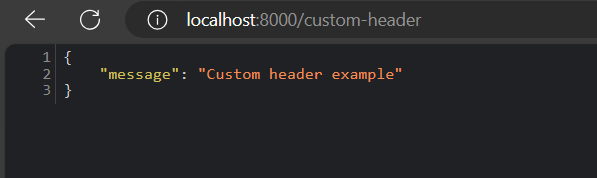
Conclusion
In conclusion , Python Falcon’s CORS support provides a reliable technique for securing cross-origin communication in web APIs. By enabling CORS in Falcon apps, developers can reduce security risks associated with illegal requests while also promoting interoperability across domains. Falcon’s simple configuration and customization options enable developers to enforce tight CORS standards.
Share your thoughts in the comments
Please Login to comment...