Python Compiler Using Django
Last Updated :
11 Mar, 2024
In this article, we will explore the creation of a Python compiler using Django. Users can input their Python code into the designated code area, and upon clicking the “Run” button, the compiler will generate the corresponding output.
What is Python Compiler?
A Python compiler is a program or tool that translates human-readable Python code into machine-readable bytecode or native machine code. Unlike an interpreter that executes code line by line, a compiler processes the entire code at once, generating an intermediate representation or executable file. Python compilers play a crucial role in transforming high-level Python code into a format that a computer’s hardware can execute directly, making the code run.
Create Python Compiler Using Django
Below, is the step-by-step guide to creating a Python Compiler Using Django:
Starting the Project Folder
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp home
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
]
File Structure
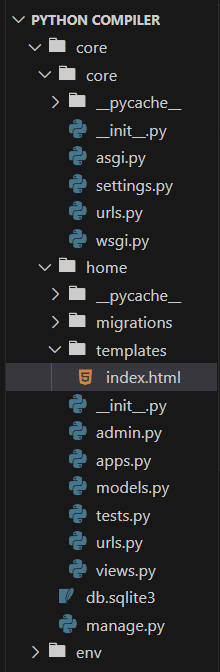
Setting Necessary Files
views.py : below,code defines a Django view for executing Python code submitted through a web form. It uses `exec` to run the code, captures the output, and handles exceptions, providing detailed error information if any. The `index` function renders an HTML template, while `runcode` processes a POST request containing Python code, executes it, and updates the web page with the code and its output.
Python3
from django.shortcuts import render
import traceback
from io import StringIO
from contextlib import redirect_stdout
def execute_code(code):
try :
output_buffer = StringIO()
with redirect_stdout(output_buffer):
exec (code)
output = output_buffer.getvalue()
except Exception as e:
output = f "Error: {str(e)}\n{traceback.format_exc()}"
return output
def index(request):
return render(request, 'index.html' )
def runcode(request):
if request.method = = "POST" :
codeareadata = request.POST[ 'codearea' ]
output = execute_code(codeareadata)
return render(request, 'index.html' , { "code" : codeareadata, "output" : output})
return HttpResponse( "Method not allowed" , status = 405 )
|
core/urls.py : below code sets up URL patterns for a Django project. It includes the admin interface at ‘/admin/’ and incorporates URLs from the ‘home’ app at the project’s root (‘/’).
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' home.urls')),
]
|
home/urls.py : below Django URL configuration defines two patterns. The empty path maps to the ‘index’ view, identified as ‘indexpage’. The ‘runcode’ path links to the ‘runcode’ view.
Python3
from django.urls import path, include
from . import views
urlpatterns = [
path('', views.index, name = "indexpage" ),
path( 'runcode' , views.runcode, name = "runcode" ),
]
|
Creating GUI
home/templates/index.html : This HTML file creates a straightforward Python compiler web page with Django. It features a CodeMirror code area, a ‘Run Code’ button, and an output section. The design is clean with a green color scheme and a GeeksforGeeks-themed header. The button triggers code execution, and the output is displayed below the input area. The page maintains a responsive layout.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1, shrink-to-fit=no" >
< title >GeeksforGeeks</ title >
< style >
body {
font-family: 'Arial', sans-serif;
background-color: #f0f4f7;
margin: 0;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
min-height: 100vh;
}
form {
width: 100%;
max-width: 800px;
background-color: #ffffff;
padding: 30px;
border-radius: 10px;
box-shadow: 0 6px 12px rgba(0, 0, 0, 0.1);
}
label {
display: block;
margin-bottom: 12px;
font-weight: bold;
color: black;
font-family: 'Times New Roman', Times, serif;
}
#codearea,
#output {
height: 300px;
font-size: 16px;
width: 100%;
border: 1px solid green;
border-radius: 5px;
padding: 12px;
margin-bottom: 24px;
resize: vertical;
}
#output {
height: 150px;
padding: 5px;
}
button {
color: green;
border: none;
padding: 10px 20px;
border-radius: 5px;
border: 1px solid green;
cursor: pointer;
font-size: 15px;
}
button:hover {
background-color: #388e3c;
color: white;
}
</ style >
</ head >
< body >
< h2 style = "font-family: 'Times New Roman', Times, serif; " > < strong style = "color: green;" >GeeksforGeeks</ strong > Python Compiler</ h2 >
< form action = "/runcode" method = "post" >
{% csrf_token %}
< div >
< label for = "codearea" >Code Area</ label >
< textarea id = "codearea" name = "codearea" rows = "10" >{{ code }}</ textarea >
</ div >
< hr >
< div >
< label for = "output" >Output</ label >
< textarea id = "output" name = "output" rows = "4" disabled>{{ output }}</ textarea >
</ div >
< button type = "submit" >Run Code</ button >
</ form >
< script >
var codeEditor = CodeMirror.fromTextArea(document.getElementById("codearea"), {
mode: "python",
lineNumbers: true,
indentUnit: 4,
extraKeys: { Tab: "indentMore", "Shift-Tab": "indentLess" },
});
</ script >
</ body >
</ html >
|
Deployment of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
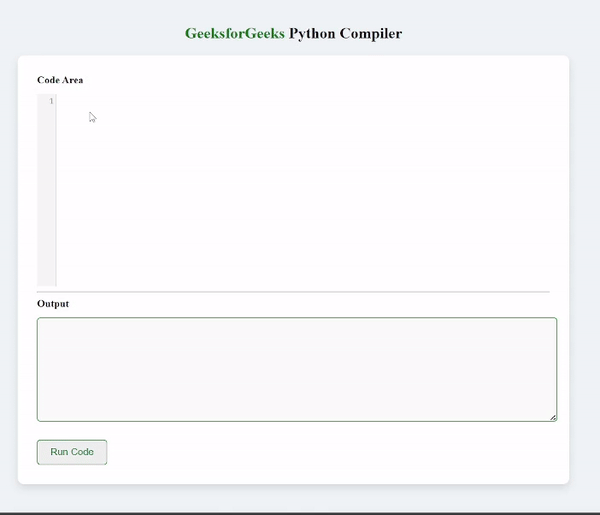
Share your thoughts in the comments
Please Login to comment...