Perl | goto statement
Last Updated :
20 Jun, 2019
The goto
statement in Perl is a jump statement which is sometimes also referred to as unconditional jump statement. The goto
statement can be used to jump from anywhere to anywhere within a function.
Syntax:
LABEL:
Statement 1;
Statement 2;
.
.
.
.
.
Statement n;
goto LABEL;
In the above syntax, the goto
statement will instruct the compiler to immediately go/jump to the statement marked as LABEL. Here label is a user-defined identifier which indicates the target statement. The statement immediately followed after ‘label:’ is the destination statement.
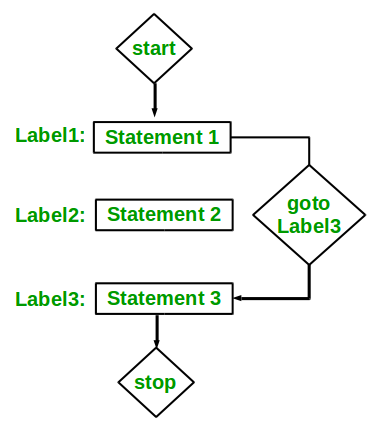
goto
statement in Perl is of three forms- Label, Expression, and Subroutine.
- Label: It will simply jump to the statement marked with the LABEL, and will continue the execution from that statement.
- Expression: In this form, there will be an expression that will return a Label name after evaluation and goto will make it jump to the labeled statement.
- Subroutine: goto will transfer the compiler to the subroutine of the given name from the currently running subroutine.
Syntax:
goto LABEL
goto EXPRESSION
goto Subroutine_Name
goto
using LABEL name: LABEL name is used to jump to a specific statement in code and will start execution from that statement. Its reach is limited though. It can work only within the scope from where it is being called.
Example:
sub printNumbers()
{
my $n = 1;
label:
print "$n " ;
$n ++;
if ( $n <= 10)
{
goto label;
}
}
printNumbers();
|
Output:
1 2 3 4 5 6 7 8 9 10
goto
using Expression: An expression can also be used to give a call to a specific label and pass the execution control to that label. This expression when passed to the goto
statement, evaluates to generate a label name, and further execution is continued from that statement defined by that label name.
Example:
$a = "lab" ;
$b = "el" ;
sub printNumbers()
{
my $n = 1;
label:
print "$n " ;
$n ++;
if ( $n <= 10)
{
goto $a . $b ;
}
}
printNumbers();
|
Output:
1 2 3 4 5 6 7 8 9 10
goto
using Subroutine: A subroutine can also be called with the use of the goto
statement. This subroutine is called from within another subroutine or individually based on its use. It holds the work that is to be performed next to the calling statement. This method can be used to call a function recursively to print a series or a range of characters.
Example:
sub label
{
print "$n " ;
$n ++;
if ( $n <= 10)
{
goto &label;
}
}
my $n = 1;
label();
|
Output:
1 2 3 4 5 6 7 8 9 10
Share your thoughts in the comments
Please Login to comment...