How to create helper functions with EJS in Express ?
Last Updated :
05 Mar, 2024
Helper functions in EJS are JavaScrict functions that you can define and use within your templates. These functions can perform various tasks, such as formatting dates, generating dynamic content, or handling repetitive logic. By creating helper functions, you can keep your template code clean and organized, making it easier to maintain and understand.
In this article, we will explore how to create helper functions with EJS in Express.
Steps to create helper functions with EJS in Express:
Step 1: Initialize the node application using the following command.
npm init -y
Step 2: Install the required dependencies:
npm install express ejs nodemon
Folder Structure:

The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Example: Now create the required files as shown in folder structure and add the following codes.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Formatting date</ title >
</ head >
< body >
< h1 >
<%= message %>
</ h1 >
< p >Today's date is: <%= formatDate(new Date()) %>
</ p >
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'ejs' );
app.set( 'views' , __dirname + '/views' );
app.get( '/' , (req, res) => {
res.render( 'index' ,
{ message: 'Formatting date using helper function in ejs' });
});
app.locals.formatDate = (date) => {
if (!date || !(date instanceof Date)) return '' ;
const options = {
year: 'numeric' ,
month: 'long' , day: 'numeric'
};
return date.toLocaleDateString( 'en-US' , options);
};
app.listen(port, () => {
console.log(`Server is running at http:
});
|
To start the application run the following command
nodemon app.js
Output:
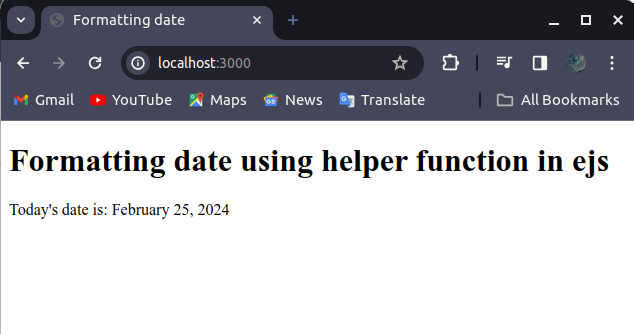
Formatting date using helper function in ejs
Share your thoughts in the comments
Please Login to comment...