How to Use Embedded JavaScript (EJS) as a Template Engine in Express JS ?
Last Updated :
13 Mar, 2024
Embedded JavaScript (EJS) is a simple templating language that helps us to generate HTML markup with plain JavaScript. It’s commonly used with Node.js to create dynamic web pages. It also helps to incorporate JavaScript into HTML pages.
Approach to use EJS as Template Engine in Express JS:
- Install EJS: First, you need to install EJS in your Node.js project.
- Set up your Node.js project: Create a Node project. Ensure you have a directory structure set up, with your main Node.js file (e.g., app.js or server.js) and a directory for your views/templates (e.g., views/).
- Configure Express.js: If you’re using Express, you must set up your Express application to use EJS as the view engine.
- Create EJS templates: Inside the views/ directory, create your EJS template files with the .ejs extension.
Steps to create application:
Step 1: Create a project folder i.e. foldername, move to it using the following command:
cd foldername
Step 2: Initialize Your Node.js Project
npm init
Step 3: Install dependencies
npm install express, ejs
Step 4: After creating necessary files move to the folder using the following command:
cd foldername
Project Structure:
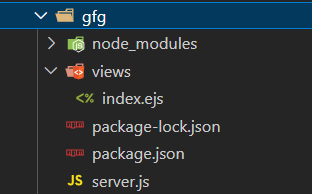
The updated dependencies in package.json file will look like.
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Example: In this example we will simply print “Hellow World” with hte help of EJS in Node.js. In this EJS file, <%= title %> and <%= message %> are placeholders that will be replaced with the values passed from the Node.js application.
HTML
//index.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><%= title %></title>
</head>
<body>
<h1><%= title %></h1>
<p><%= message %></p>
</body>
</html>
Node
//server.js
const express = require('express');
const app = express();
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Set the directory for your views/templates
app.set('views', __dirname + '/views');
console.log(__dirname + '/views');
// Define your routes
app.get('/', (req, res) => {
// Render the index.ejs file in the views directory
res.render('index', { title: 'Hello World',
message: 'Welcome to my website!!'
});
});
// Start the server
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Output:
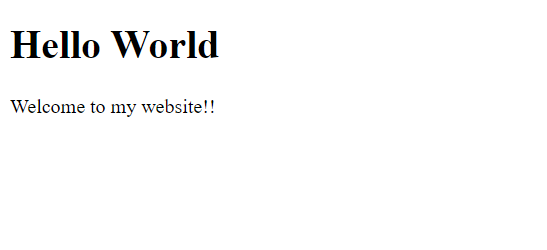
Share your thoughts in the comments
Please Login to comment...