Next JS File Conventions: not-found.js
Last Updated :
29 Feb, 2024
NextJS is a React framework that provides several features to help you build fast and scalable web applications. One of these features is not-found.js which allows you to create a “Not Found” UI for your application which is rendered whenever the “notFound” function is thrown within a route segment.
Next JS Not Found Handling and Access Control:
In NextJS, you can use the “notFound” function to hide certain pages from certain users (for example, you might want to hide the admin page from a normal user). In those cases, the UI shown to the user can be defined inside the “not-found.js” file created inside that route segment. Also, Next.js will return a 200 HTTP status code for streamed responses, and a 404 HTTP status code for non-streamed responses.
Along with that, the root “not-found.js” file also gets rendered whenever the user goes to any unmatched URL that is not defined by the application.
Syntax:
To create the “Not Found” UI, create a “not-found.js” file inside your route segment and then export your component from that file as shown below:
// inside not-found.js
export default function NotFound(){
return <> Your Code <>
}
Props:
not-found.js
components do not accept any props. Components defined in “not-found.js” cannot receive or utilize any external data passed as props. This restriction limits their ability to dynamically adapt or display content based on external input, making them static in nature.
Data Fetching:
By default, “not-found.js” functions as a Server Component. To enable data fetching and display based on path using Client Component hooks like usePathname, mark it as async. However, if client-side hooks are essential, fetch data on the client-side instead.
Example: Below is a sample example of async function Notfound.
import Link from 'next/link'
import { headers } from 'next/headers'
export default async function NotFound() {
const headersList = headers();
const domainName = headersList.get('host');
const dataFetch = await getSiteData(domainName);
return (
<div>
<h2>Not Found: {dataFetch.name}</h2>
<p>Could not the resource requeste</p>
<p>
View
<Link href="/blog">
All your Posts are here
</Link>
</p>
</div>
)
}
Steps to Implement not-found File Convention in Next JS:
Step 1: To create your app, run the following npx command in your terminal to start the creation process:
npx create-next-app@latest
Step 2: Provide the necessary details to create your app as shown in the image below.
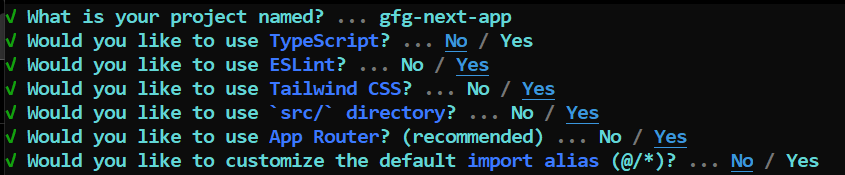
Providing the necessary details to create-next-app
Step 3: Your project would be created by now. Go inside your project directory and start the development server through the following command:
npm run dev
Project Structure:
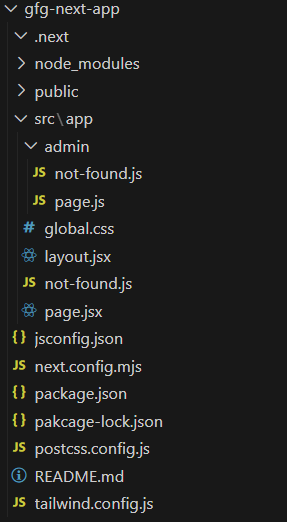
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.1.0"
},
"devDependencies": {
"autoprefixer": "^10.0.1",
"postcss": "^8",
"tailwindcss": "^3.3.0",
"eslint": "^8",
"eslint-config-next": "14.1.0"
}
Example 1: In this example, let’s create a “not-found.js” file inside the app directory which will serve whenever the user is present on an unmatched URL and provide the user with the link to go back to the home page.
Javascript
import Link from "next/link" ;
const NotFoundPage = () => {
return (
<div className= "p-4" >
<h1 className= "text-2xl font-bold
text-emerald-500" >GeeksforGeeks</h1>
<h3 className= "mt-1 text-lg
font-semibold" >not-found.js | Next.js</h3>
<p className= "mt-3" >
Sorry! Looks like you
are on a wrong page.
</p>
<div>
<Link href= "/"
className= "text-emerald-500
font-bold underline" >
Go back to home >
</Link>
</div>
</div>
);
};
export default NotFoundPage;
|
Output: Now, whenever user goes to any unmatched route, suppose “http://localhost:3000/abcd”, which is not defined by our application, then the user is shown the “NotFoundPage” UI.
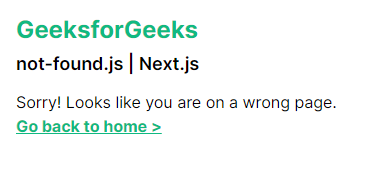
Example 1: Output of not-found.js file in Next.js whenever user goes to an unmatched URL
Example 2: In this example, let’s create an admin page which accepts a “searchParam” telling the role of the current user. If the role is not equal to “admin” then, we throw the “notFound” function on that page, and the UI inside “not-found.js” file of that route segment is shown to the user.
Javascript
import { notFound } from "next/navigation" ;
export default function Adminpage({ searchParams }) {
if (searchParams[ "role" ] !== "admin" ) {
notFound();
}
return <p>You are on admin page.</p>;
}
|
Javascript
import Link from "next/link" ;
export default function NotFoundPage() {
return (
<div className= "p-4" >
<h1 className= "text-2xl font-bold
text-emerald-500" >
GeeksforGeeks
</h1>
<h3 className= "mt-1 text-lg
font-semibold" >
not-found.js | Next.js
</h3>
<p className= "mt-3" >
Sorry! Looks like you are not
authorized to access this page.
</p>
<div>
<Link href= "/"
className= "text-emerald-500
font-bold underline" >
Go back to home >
</Link>
</div>
</div>
);
}
|
Output: Now, whenver the role of the user is not equal to “admin” (in the searchParams), (for example: in case of “http://localhost:3000/admin?role=user”), the user is shown with the “NotFoundPage” UI.
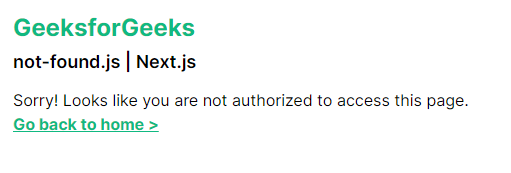
Example 2: Showing the not-found.js whenever an unauthorized user tries to visit a page
Share your thoughts in the comments
Please Login to comment...