Manipulating HTML Elements with JavaScript
Last Updated :
26 Apr, 2023
JavaScript is a powerful language that can be used to manipulate the HTML elements on a web page. By using JavaScript, we can access the HTML elements and modify their attributes, styles, and content. This allows us to create dynamic and interactive web pages.
Methods to Identify the elements to manipulate: There are several methods to identify the HTML elements that we want to manipulate using JavaScript. The most common methods are:
- Using the element’s ID: We can assign an ID to an HTML element and use document.getElementById() to access it.
- Using the element’s class name: We can assign a class name to an HTML element and use document.getElementsByClassName() to access it.
- Using the element’s tag name: We can use document.getElementsByTagName() to access all elements with a particular tag name.
Accessing the properties of the elements: Once we have identified the HTML element that we want to manipulate, we can access its properties using JavaScript. For example, to access the text content of an element, we can use the innerHTML property. Similarly, we can access the style properties of an element using the style property.
Use Event Listeners to Respond to User Interactions: We can use event listeners to respond to user interactions such as clicking a button or hovering over an element. Event listeners are functions that are executed when a particular event occurs. For example, we can use the onclick event listener to execute a function when a button is clicked.
When building a website or web application, it’s common to need to access and manipulate HTML elements using JavaScript. There are several methods available in JavaScript that allow you to do this, including getElementById(), getElementsByClassName(), and getElementsByTagName(). In this article, we’ll take a systematic approach to use these methods and show you how to access and manipulate elements in your HTML documents.
Step 1: Identify the Element You Want to Manipulate: The first step in working with HTML elements in JavaScript is to identify the element you want to manipulate. There are several ways to do this, depending on the specific element you’re trying to access. Here are some common methods:
- getElementById() Method: Use this method to access an element with a specific ID. IDs should be unique within an HTML document, so this method will always return a single element.
- getElementsByClassName() Method: Use this method to access all elements with a specific class name. Multiple elements can have the same class name, so this method will return a collection of elements.
- getElementsByTagName() Method: Use this method to access all elements with a specific tag name. Multiple elements can have the same tag name, so this method will also return a collection of elements.
Syntax:
let element = document.getElementById("my-id");
let elements = document.getElementsByClassName("myClass");
let elements = document.getElementsByTagName("p");
Step 2: Access the Element’s Properties and Methods: Once you’ve identified the element you want to manipulate, you can access its properties and methods. Properties are values that describe the state of an element, such as its innerHTML, value, or src attributes. Methods are functions that allow you to manipulate an element in some way, such as changing its innerHTML, className, or style properties. Here are some examples of how to access an element’s properties and methods:
// Accessing an element's methods
element.innerHTML = "New content!"; // changes the content of the element
element.classList.add("newClass"); // adds a new CSS class to the element
element.style.backgroundColor = "red"; // changes the background color of the element
Step 3: Use Event Listeners to Respond to User Interactions: In addition to manipulating elements directly, you can also use JavaScript to respond to user interactions, such as clicks, mouse movements, or keyboard inputs. To do this, you can use event listeners, which are functions that are triggered when a specific event occurs on an element. Here’s an example of how to add an event listener to a button element:
<button id="myButton">Click me!</button>
<script>
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
console.log("Button clicked!");
});
</script>
In this example, we use getElementById() to access a button element with the ID “myButton”. We then add an event listener to the button using the addEventListener() method. The event we’re listening for is a “click” event, which is triggered when the user clicks the button. When the event is triggered, the function we passed as the second argument is executed, which logs a message to the console.
Example 1. Id selector
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >document.getElementById()</ title >
</ head >
< body >
< h1 id = "my-id" >Hello World!</ h1 >
< script >
// Get element with id "my-id"
var elements = document.getElementById("my-id");
// Change the content of the element
elements.innerHTML = "New content!";
</ script >
</ body >
</ html >
|
Output:
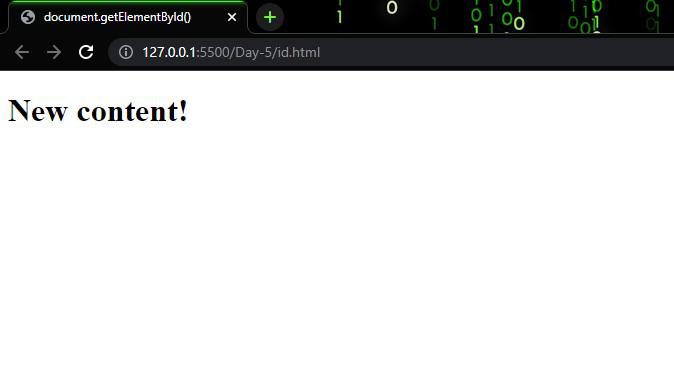
id.html
Example 2. Class Selector:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >document.getElementsByClassName()</ title >
</ head >
< body >
< p class = "myClass" >This is a paragraph.</ p >
< p class = "myClass" >This is another paragraph.</ p >
< script >
// Get all elements with class "myClass"
var elements = document.getElementsByClassName("myClass");
// Change the content of the first element
elements[0].innerHTML = "New content!";
</ script >
</ body >
</ html >
|
Output:
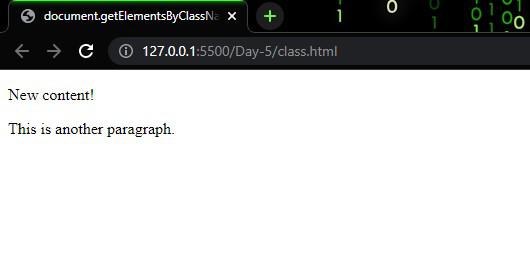
class.html
Example 3: Here is an example of how to manipulate an HTML element using JavaScript:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >DOM Manipulation</ title >
</ head >
< body >
< h1 id = "my-heading" >Hello, World!</ h1 >
< button id = "my-button" >Click me!</ button >
< script >
const heading = document.getElementById("my-heading");
const button = document.getElementById("my-button");
button.addEventListener("click", function () {
heading.innerHTML = "Button clicked";
heading.style.color = "red";
});
</ script >
</ body >
</ html >
|
Explanation: In this example, we first access the button element using the getElementById() method. We then add an event listener to the button using the addEventListener() method. The event we are listening for is the “click” event, and the function we want to execute when the event occurs is defined inline.
Output:
Conclusion: In conclusion, manipulating HTML elements with JavaScript is a powerful technique that can be used to create dynamic and interactive web pages. By using the methods discussed above, we can identify and access the HTML elements on a web page and modify their properties to create the desired behavior.
Share your thoughts in the comments
Please Login to comment...