HTML | DOM HTML Object
Last Updated :
07 Feb, 2019
The HTML Object property in HTML DOM is used to represent or access the HTML <html> element with in the object. The <html> element is used to return the HTML document as an Element Object.
Syntax:
Property Values:
- getElementsByTagName(): It is used to return a collection of all child elements with the specified tag name.
- innerHTML: It is used to set or return the content of an element.
- getElementsById(): It is used to return a collection of all child elements with the specified Id.
Example-1: Access HTML element using document.getElementsByTagName(“HTML”)[0];
<!DOCTYPE html>
< html >
< title >
HTML | DOM HTML Object Property
</ title >
< style >
body {
text-align: center;
width: 70%;
}
h1 {
color: green;
}
h1,
h2 {
text-align: center;
}
</ style >
< body >
< h1 >GeeksforGeeks</ h1 >
< h2 > HTML Object</ h2 >
< p >Click the button to get the
HTML content of the html element.</ p >
< button onclick = "GFG()" >Click</ button >
< p id = "Geeks" ></ p >
< script >
function GFG() {
// Access html element and
return using "innerHTML"
var x =
document.getElementsByTagName(
"HTML")[0].innerHTML;
document.getElementById("Geeks").innerHTML = x;
}
</ script >
</ body >
</ html >
|
Output:
Before Click On the Button:
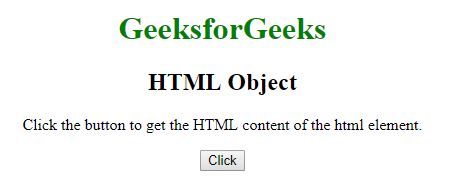
After Click On the Button:
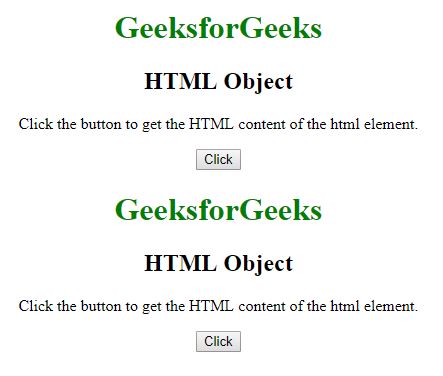
Example-2: Access html element and return element is first or second.
<!DOCTYPE html>
< html >
< title >
HTML | DOM HTML Object Property
</ title >
< style >
body {
text-align: center;
width: 70%;
}
h1 {
color: green;
}
h1,
h2 {
text-align: center;
}
</ style >
< body >
< h1 >GeeksforGeeks</ h1 >
< h2 > HTML Object</ h2 >
< p >Click the button to get the
HTML content of the html element.</ p >
< p >Using the document.documentElement</ p >
< button onclick = "GFG()" >Click</ button >
< p id = "Geeks" ></ p >
< script >
function GFG() {
// Access html element and return html
// with position value of html element.
var x =
document.documentElement.innerHTML;
document.getElementById(
"Geeks").innerHTML = "first" + x;
var y =
document.documentElement.innerHTML;
document.getElementById(
"Geeks").innerHTML = y + "second";
}
</ script >
</ body >
</ html >
|
Output:
Before Click On the Button:
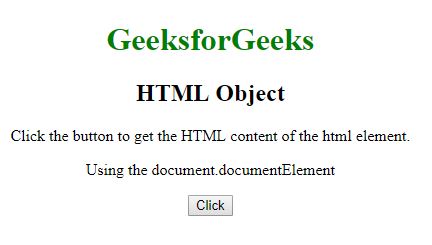
After Click On the Button:
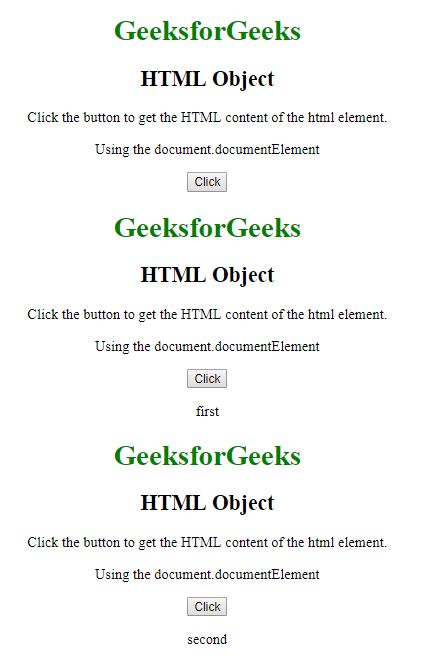
Example-3: Access html element and return all child with specified tag name.
<!DOCTYPE html>
< html >
< title >
HTML | DOM HTML Object Property
</ title >
< style >
body {
text-align: center;
width: 70%;
}
h1 {
color: green;
}
h1,
h2 {
text-align: center;
}
</ style >
< body >
< h1 >GeeksforGeeks</ h1 >
< h2 > HTML Object</ h2 >
< p >Click the button to get the
HTML content of the html element.</ p >
< p >Using the getElementsByTagName("HTML")[0]
and documentElement</ p >
< button onclick = "GFG()" >Click</ button >
< p id = "Geeks" ></ p >
< script >
function GFG() {
// access and return html element
var x =
document.getElementsByTagName(
"HTML")[0].innerHTML;
document.getElementById("Geeks").innerHTML =
"getElementsByTagName" + x;
var y =
document.documentElement.innerHTML;
document.getElementById("Geeks").innerHTML =
y + "documentElement";
}
</ script >
</ body >
</ html >
|
Output:
Before Click On the Button:
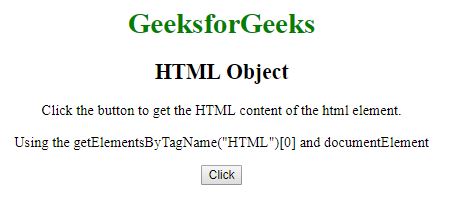
After Click On the Button:
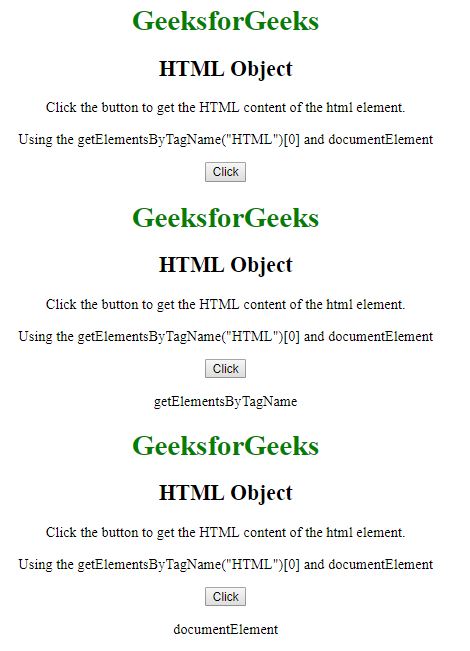
Supported Browsers: The browser supported by DOM HTML Object property are listed below:
- Google Chrome
- Internet Explorer
- Firefox
- Opera
- Safari
Share your thoughts in the comments
Please Login to comment...