Managing Local Storage & Session Storage using React Hooks
Last Updated :
28 Mar, 2024
To manage Loacal Storage and Session Storage, we can use hooks like useEffect and useState provided by React. Managing local or session storage is a repetitive task, so it is a good practice to create a custom hook that manages the storage properly.
In this article, I’ll cover the whole process of managing storage with the best industry standard practice.
To see some working examples we need to first setup our react app. . To setup a basic React App, refer to Folder Structure for a React JS Project article.
Now, we can create custom hooks. So, There are basically three operations that we need to perform:
- Insert the key-value pair
- Update the value with key
- Get the value by key
1. useLocalStorage():
Create a state that will store either the data from the local storage if present otherwise it will store initial value.Then everytime, when key or value changes we will the new key-value pair in the local storage. That’s it.
Example: Below is the code example for useLocalStorage():
JavaScript
// App.js
import React,
{
useState,
useEffect
} from 'react';
// Custom hook to manage localStorage
const UseLocalStorage = (key, initialValue) => {
const [value, setValue] = useState(() => {
const storedValue = localStorage.getItem(key);
return storedValue ?
JSON.parse(storedValue) :
initialValue;
});
useEffect(() => {
localStorage.setItem(key, JSON.stringify(value));
}, [key, value]);
return [value, setValue];
};
// Example usage
const App = () => {
const [localStorageValue, setLocalStorageValue] =
UseLocalStorage('myLocalStorageKey', 'default');
return (
<div>
<p>Local Storage Value: {localStorageValue}</p>
<button onClick={
() =>
setLocalStorageValue('new value')}>
Change Local Storage Value
</button>
</div>
);
};
export default App;
Output:
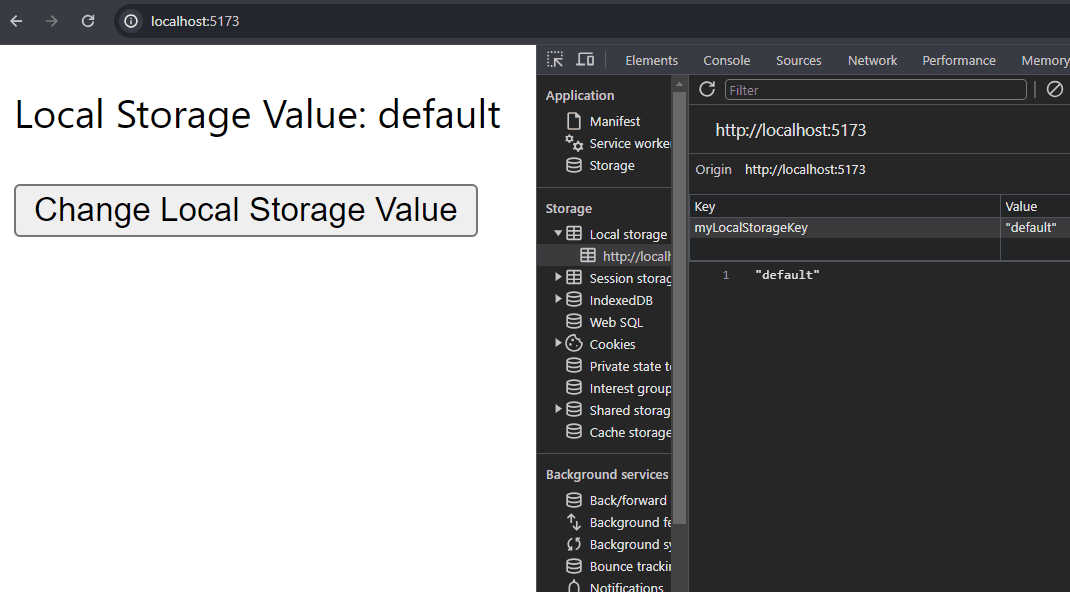
useLocalStorage()
2. useSessionStorage():
Create a state that will store either the data from the session storage if present otherwise it will store initial value.Then everytime, when key or value changes we will the new key-value pair in the session storage. That’s it.
Example: Below is the code example for useSessionStorage():
JavaScript
// App.js
import React,
{
useState,
useEffect
} from 'react';
// Custom hook to manage sessionStorage
const UseSessionStorage = (key, initialValue) => {
const [value, setValue] = useState(() => {
const storedValue = sessionStorage.getItem(key);
return storedValue ? JSON.parse(storedValue) : initialValue;
});
useEffect(() => {
sessionStorage.setItem(key, JSON.stringify(value));
}, [key, value]);
return [value, setValue];
};
// Example usage
const App = () => {
const [sessionStorageValue, setSessionStorageValue] =
UseSessionStorage('mySessionStorageKey', 'default');
return (
<div>
<p>Session Storage Value: {sessionStorageValue}</p>
<button
onClick={
() =>
setSessionStorageValue('new value')
}>
Change Session Storage Value
</button>
</div>
);
};
export default App;
Output:
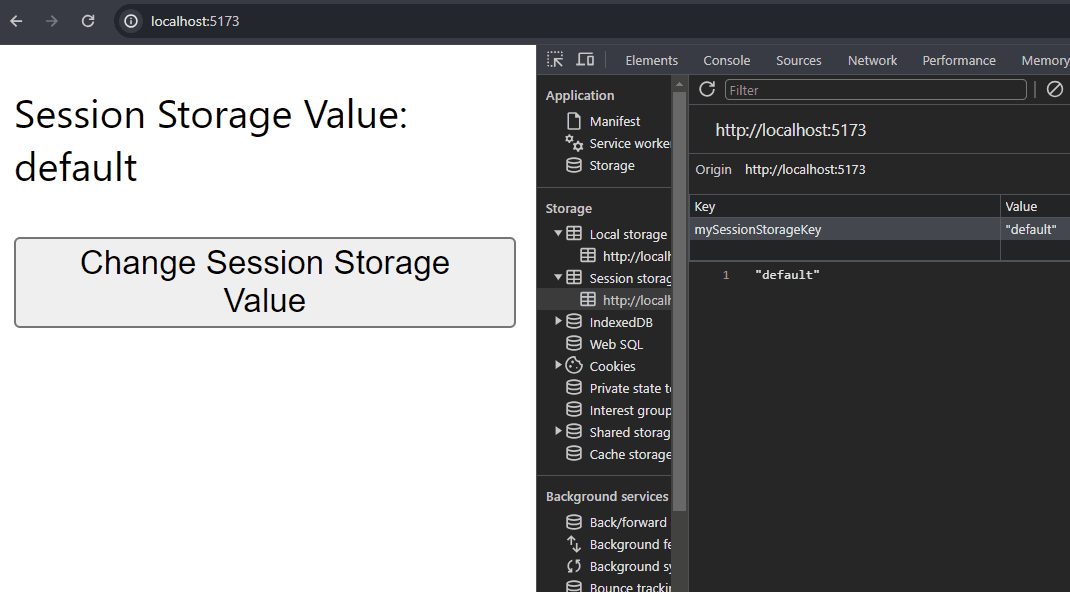
Session Storage
Share your thoughts in the comments
Please Login to comment...