How to persist state with Local or Session Storage in React ?
Last Updated :
23 Feb, 2024
Persisting state with localStorage
or sessionStorage
is a way to store data in the user’s web browser so that it remains available even after the page is refreshed or closed.
Persisting state with localStorage or sessionStorage:
- localStorage vs. sessionStorage:
localStorage
persists data until explicitly cleared by the user or the web application.
sessionStorage
persists data only for the duration of the page session. The data is cleared once the session ends (when the browser window is closed).
- Storing Data:
- To store data, you can use the
setItem
()
method provided by both localStorage
and sessionStorage
.
- It takes two parameters: the key (a string) and the value (a string).
localStorage.setItem('username', 'GeeksforGeeks');
- Retrieving Data:
- To retrieve data, you use the
getItem
()
method, passing the key as a parameter.
const username = localStorage.getItem('username');
- Updating Data:
- To update data, you use the setItem() method, similar to the storing data, you jus set a new value for the same key.
localStorage.setItem('username', 'GfG');
- Removing Data:
- To remove a specific item, you use the
removeItem
()
method and pass the key as a parameter.
localStorage.removeItem('username');
- Clearing All Data:
- To clear all stored data (all key-value pairs), you can use the
clear
()
method.
localStorage.clear();
- Usage Considerations:
- Remember that
localStorage
and sessionStorage
can only store strings. If you need to store objects or arrays, you will need to serialize and deserialize them using methods like JSON.stringify()
and JSON.parse()
.
To persist state using React’s useState
hook along with localStorage
, you can follow these steps:
- Initialize State from localStorage: When your component mounts, you can retrieve the initial state from
localStorage
.
- Update State and localStorage: Whenever the state changes, update both the state and
localStorage
to reflect the changes.
Example: Below is an example of persisting state with localStorage or sessionStorage.
Javascript
import React, {
useState,
useEffect
} from 'react' ;
function Counter() {
const [count, setCount] = useState(() => {
const storedCount = localStorage.getItem( 'count' );
return storedCount ? parseInt(storedCount) : 0;
});
useEffect(() => {
localStorage.setItem( 'count' , count.toString());
}, [count]);
const increment = () => {
setCount(prevCount => prevCount + 1);
};
const decrement = () => {
setCount(prevCount => prevCount - 1);
};
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
|
Output:
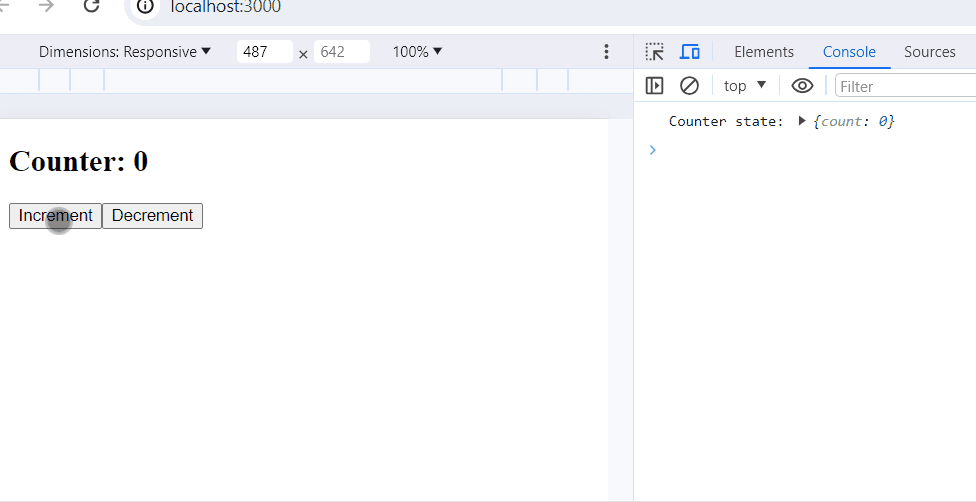
Share your thoughts in the comments
Please Login to comment...