Design patterns are typical solutions to common problems in software design. Rather than being a code solution, they are general concepts you can implement in your software to expect certain behavior from it. Design patterns are not specific to any programming language or technology, but they can be implemented in different ways depending on the specific technology being used.
Design patterns are used in web development to create applications that are maintainable, efficient, and scalable. They provide a way to structure code and data in a way that is both easy to understand and maintain.
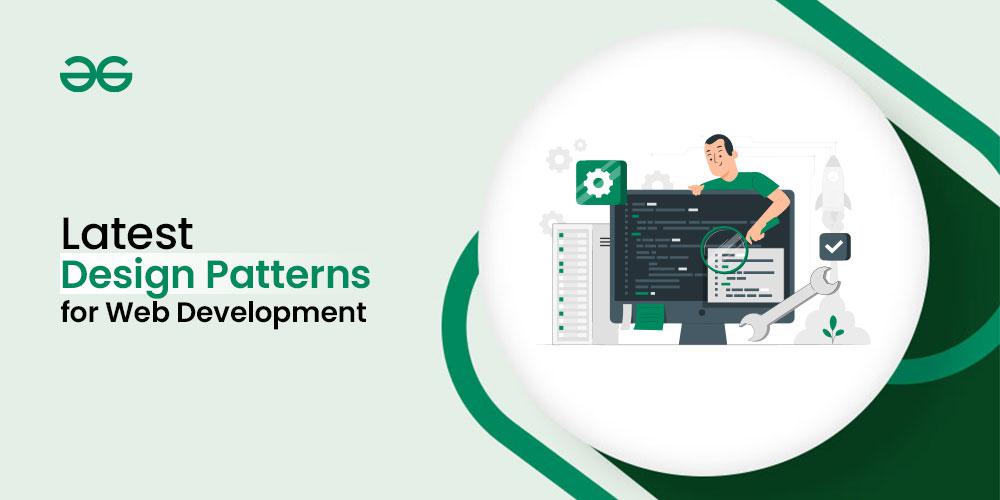
Important Topics for the Latest Design Patterns for Web Development
Types of Design Patterns
There are 3 types of design patterns depending on their behavior.
- Creational patterns: Provide a structure to create objects easily, provide certain flexibility on its creation, and reuse existing code.
- Structural patterns: Provide a structure that helps assemble objects and classes into larger structures.
- Behavioral patterns: Assign responsibilities between objects and take care of communication.
The Model-View-Controller (MVC) pattern is a software architecture pattern that separates an application into three parts: the model, the view, and the controller. The model is responsible for managing the data, the view is responsible for displaying the data, and the controller is responsible for handling user input and updating the model and view accordingly. This pattern promotes the separation of concerns and improves code organization.
Although this Design Pattern was originally used for desktop applications, it has become increasingly popular for all sorts of web applications as it helps to easily build the application design.
Example: Popular web frameworks like Ruby on Rails and Laravel utilize the MVC pattern.
.jpg)
The MVC pattern helps you break up the frontend and backend code into separate components. This way, it’s much easier to manage and make changes to either side without them interfering with each other.
2. Model View Presenter(MVP)
The Model-View-Presenter (MVP) design pattern is a derivation of the Model-View-Controller (MVC) architectural pattern, and is used mostly for building user interfaces.
The MVP pattern is a UI presentation pattern based on the concepts of the MVC pattern. However, it doesn’t specify how to structure the whole system. It only dictates how to structure the view.This pattern separates responsibilities across four components in general. Firstly the view is responsible for rendering UI elements. Secondly, the view interface is used to loosely couple the presenter from its view.
Finally, the presenter interacts with the view and model, and the model is responsible for business behaviors and state management. MVP is a user interface architectural pattern engineered to facilitate automated unit testing and improve the separation of concerns in presentation logic.
This pattern divides an application into three major aspects: Model, View, and Presenter.
- Model: The Model represents a set of classes that describes the business logic and data. It also defines business rules for data means how the data can be changed and manipulated.
- View: View is a component which is directly interacts with user like XML, Activity, fragments. It does not contain any logic implemented.
- Presenter: The Presenter receives the input from users via View, then process the user’s data with the help of Model and passing the results back to the View. Presenter communicates with view through interface. Interface is defined in presenter class, to which it pass the required data.
MVVM pattern supports two-way data binding between View and View-Model. This allows automatic propagation of changes, inside the state of View-Model to the View. Generally, the View-Model utilizes the observer pattern to inform changes in the View-Model to the Model.
MVVM (Model- View- ViewModel) is separated into three components:
- View: View is described in XAML, and it must not have any logic in code behind it is bound in ViewModel by using data binding.
- Model: The model is mainly used for representing data in the method, which is simply delicate by WPF.
- ViewModel: ViewModel is the model for presenting the application or, we can say, the abstraction of view. It depicts the data applicable to the view and represents the activities for the view, generally with commands.
The bi-directional data binding or the two way data binding between the view and the View-Model ensures that the models and properties in the View-Model is in sync with the view. The MVVM design pattern is well suited in applications that need support for bi-directional data binding.
Singleton is a creational design pattern that ensures only a single instance of a class exists throughout an application and provides access to that instance from anywhere in the codebase. It is useful in situations where multiple instances of a class are undesirable or a single point of control or coordination is required. Typical examples include logging systems, database connection managers, caches, and thread pools, among others.
Example: A database connection manager in a web application can be implemented using the Singleton pattern.
- Use this pattern when you need to ensure control access to a resource that might return inconsistencies if it is changed by two different objects at the same time. (Ex. Databases, state of an object)
- The singleton pattern only allows a class or object to have a single instance and it uses a global variable to store that instance. You can use lazy loading to make sure that there is only one instance of the class because it will only create the class when you need it.
Advantages of the Singleton Design Pattern
- Single Instance: The Singleton Pattern guarantees that a class has only one instance throughout the application. This can be useful in scenarios in which multiple instances must be avoided, such as when managing shared resources or configurations.
- Global Access: Because a Singleton instance is globally accessible, it is possible to access its methods and properties from anywhere in the application. This eliminates the need to pass objects’ instances or manage complex dependencies.
- Lazy Initialization: The Singleton pattern supports lazy initialization, which means that the instance is created only when it is accessed for the first time. This can improve performance by deferring instantiation until it is required.
- Thread Safety: The Singleton implementation can be made thread-safe, allowing multiple threads to access the instance without causing concurrency issues. This is particularly critical in multi-threaded applications.
Real-world examples of Singleton Design Pattern
- Logger
- Database Connection
- Cache Manager
In object-oriented programming, the Factory Method is a Creational Design Pattern that allows us to create objects without specifying the exact class that will be instantiated. Instead of creating objects directly, we use a factory method to create and return them.
This Design Pattern promotes code reuse and loose coupling between classes making our code more modular and easier to maintain. It enables us to create objects without knowing the implementation details of our classes.
Example: Creating different types of payment gateways in an e-commerce application using the Factory pattern.
The Factory Method pattern consists of four main components:
- Product
- Concrete Product
- Creator
- Concrete Creator
The Product is the interface or abstract class that defines the methods that the ConcreteProduct must implement. The ConcreteProduct is the class that implements the Product interface. The Creator is the abstract class that declares the Factory Method. The ConcreteCreator is the class that implements the Factory Method and returns the ConcreteProduct.
Advantages of the Factory Method Design Pattern
- Loose coupling: The Factory Method Design Pattern promotes loose coupling between classes. The Creator class doesn’t need to know the exact class that will be instantiated. This makes the code more modular and easier to maintain.
- Encapsulation: The Factory Method Design Pattern encapsulates the object creation process. This makes it easier to modify the creation process without affecting the rest of the code.
- Code reuse: The Factory Method Design Pattern promotes code reuse by allowing multiple classes to share a common interface.
- Extensibility: The Factory Method Design Pattern is extensible. It allows new ConcreteProduct classes to be added without modifying the existing code.
The Factory Method pattern is useful when
- We don’t know the exact class of object that will be created at runtime.
- We want to encapsulate the object creation process.
- We want to promote loose coupling between classes.
- We want to promote code reuse.
A Decorator Pattern says that just “attach a flexible additional responsibilities to an object dynamically”. In other words, The Decorator Pattern uses composition instead of inheritance to extend the functionality of an object at runtime. Decorator pattern allows a user to add new functionality to an existing object without altering its structure. This type of design pattern comes under structural pattern as this pattern acts as a wrapper to existing class.This pattern creates a decorator class which wraps the original class and provides additional functionality keeping class methods signature intact.
Example:
Adding logging functionality to specific methods of a web service using the Decorator pattern.
Advantages of the Decorator Design Pattern
- It provides greater flexibility than static inheritance.
- It enhances the extensibility of the object, because changes are made by coding new classes.
- It simplifies the coding by allowing you to develop a series of functionality from targeted classes instead of coding all of the behavior into the object.
Uses of Decorator Design Pattern
- When you want to transparently and dynamically add responsibilities to objects without affecting other objects.
- When you want to add responsibilities to an object that you may want to change in future.
- Extending functionality by sub-classing is no longer practical.
Observer pattern is used when there is one-to-many relationship between objects such as if one object is modified, its depenedent objects are to be notified automatically. Observer pattern falls under behavioral pattern category. Observer pattern uses three actor classes. Subject, Observer and Client. Subject is an object having methods to attach and detach observers to a client object. We have created an abstract class Observer and a concrete class Subject that is extending class Observer.
Example:
Implementing real-time notifications in a chat application using the Observer pattern.
The Observer defines a one-to-many relationship so that when one object changes state, the others are notified and updated automatically. Some auctions demonstrate this pattern. Each bidder possesses a numbered paddle that is used to indicate a bid. The auctioneer starts the bidding, and “observes” when a paddle is raised to accept the bid. The acceptance of the bid changes the bid price which is broadcast to all of the bidders in the form of a new bid.
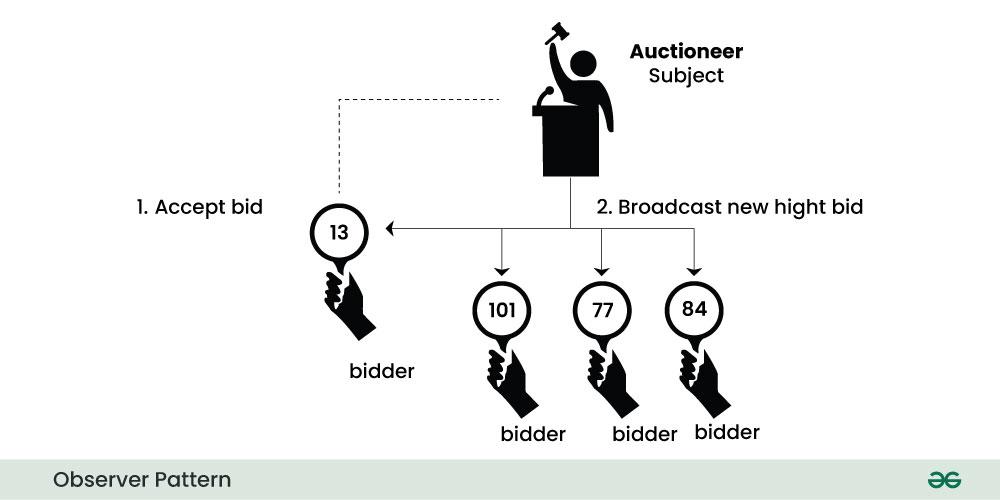
Adapter pattern works as a bridge between two incompatible interfaces. This type of design pattern comes under structural pattern as this pattern combines the capability of two independent interfaces. This pattern involves a single class which is responsible to join functionalities of independent or incompatible interfaces. A real life example could be a case of card reader which acts as an adapter between memory card and a laptop. You plugin the memory card into card reader and card reader into the laptop so that memory card can be read via laptop.
In design, adapters are used when we have a class (Client) expecting some type of object and we have an object (Adaptee) offering the same features but exposing a different interface
To use an adapter:
- The client makes a request to the adapter by calling a method on it using the target interface.
- The adapter translates that request on the adaptee using the adaptee interface.
- Client receive the results of the call and is unaware of adapter’s presence.
The adapter pattern convert the interface of a class into another interface clients expect. Adapter lets classes work together that couldn’t otherwise because of incompatible interfaces.
9. Dependency Injection Pattern
Dependency Injection is a software design pattern in which one or more dependencies (or services) are injected, or passed by reference, into a dependent object (or client) and are made part of the client’s state. The pattern separates the creation of a client’s dependencies from its own behavior, which allows program designs to be loosely coupled and to follow the inversion of control and single responsibility principles.
When to use Dependency Injection Pattern:
- When you need to remove knowledge of concrete implementation from object.
- To enable unit testing of classes in isolation using mock objects or stubs.
10. Repository Pattern
The Repository Pattern is a design pattern used in software development that provides a way to manage data access logic in a centralized location. It separates the logic that retrieves the data and maps it to the entity model from the business logic that operates on the model. The primary objective of the Repository Pattern is to simplify the data access code and achieve a cleaner architecture for the application.
The Repository Pattern consists of three main components:
- The Repository Interface: The Repository Interface defines the operations that can be performed on the entity model. These operations typically include Create, Read, Update, and Delete (CRUD) operations.
- The Repository Implementation: The Repository Implementation provides the actual implementation for the Repository Interface. It is responsible for performing the data access operations.
- The Entity Model: The Entity Model represents the business entities of the application. It typically consists of one or more classes that map to database tables.
The Strategy pattern encapsulates interchangeable algorithms within separate classes, allowing clients to select the desired algorithm at runtime. This pattern promotes flexibility and code reusability, as it enables the easy addition or modification of algorithms without impacting the client code.
Example:
Implementing different payment strategies (credit card, PayPal, etc.) in an online payment processing system using the Strategy pattern.
Command pattern is a data driven design pattern and falls under behavioral pattern category. A request is wrapped under an object as command and passed to invoker object. Invoker object looks for the appropriate object which can handle this command and passes the command to the corresponding object which executes the command. In command pattern there is a Command object that encapsulates a request by binding together a set of actions on a specific receiver. It does so by exposing just one method execute() that causes some actions to be invoked on the receiver.
When to use Command Pattern:
1. Assume a front-end team responsible for creating UI (User Interface) components such as button, dropdown, checkbox, etc. These components can then be used to build UI for any application.
For example:
The button component can be used in a calculator application.
2. The frontend team is unaware of the action that will be executed when a button is clicked. It is up to the application team to decide what action will be executed when a button is clicked. This is where the command design pattern comes in. With command design pattern, the executor of the command (button component) does not need to know anything about the command being executed. So, any action can be attached to a button component.
For example:
Execute add operation when + button is clicked and execute subtract operation when- butting is clicked. We implement command design pattern by creating separate command classes.
13. Middleware Pattern
The Middleware pattern makes it possible for components to interact with each other through a central point: the mediator. Instead of directly talking to each other, the mediator receives the requests, and sends them forward! In JavaScript, the mediator is often nothing more than an object literal or a function.
Instead of letting every objects talk directly to the other objects, resulting in a many-to-many relationship, the object’s requests get handled by the mediator. The Middleware processes this request, and sends it forward to where it needs to be.
13. Caching Patterns
The concept of caching is to temporarily store data that makes accessing it faster. Caching is employed to help save on the use of resources. When we retrieve data, it should be made available for future requests for the same data. Implementing this saves on the processing time required for continual retrieval of the same data.
Here are some of the commonly used caching patterns.
- Cache-Aside: In this pattern, cache works along side with the database where data will be lazy loaded into the cache. It will be best suited for read heavy data .
- Read-Through: As the name signifies, it tries to read the data from the cache and cache communicates with the database on lazy-load basis.
- Write-Through: In this technique, we write the data into the datastore through cache which means the data will be inserted/updated into the cache first followed by a datastore which helps to keep the data consistent across and best suited for write heavy requirements.
- Write-Back/Behind: In this technique, we make multiple data entries into the cache directly but not into the datastore simultaneously . Rather we queue the data which we suppose to be inserted/updated into the cache and replicate the queued data to the datastore at later stages.
- Write-Around: In this pattern, the data will be written directly into the data store without writing it to the cache. On read operation from the datastore, the same will be placed into the cache.
- Refresh-Ahead: In this pattern, cached data gets refreshed before it gets expired this helps in reducing latency since the data gets updated before it gets used. Later stages the same gets used during fetch .
The front controller design pattern means that all requests that come for a resource in an application will be handled by a single handler and then dispatched to the appropriate handler for that type of request. The front controller may use other helpers to achieve the dispatching mechanism.
Components are:
- Controller: The controller is the initial contact point for handling all requests in the system. The controller may delegate to a helper to complete authentication and authorization of a user or to initiate contact retrieval.
- View: A view represents and displays information to the client. The view retrieves information from a model. Helpers support views by encapsulating and adapting the underlying data model for use in the display.
- Dispatcher: A dispatcher is responsible for view management and navigation, managing the choice of the next view to present to the user, and providing the mechanism for vectoring control to this resource.
- Helper: A helper is responsible for helping a view or controller complete its processing. Thus, helpers have numerous responsibilities, including gathering data required by the view and storing this intermediate model, in which case the helper is sometimes referred to as a value bean.
Share your thoughts in the comments
Please Login to comment...