MVC Design Pattern
Last Updated :
19 Feb, 2024
The MVC design pattern is a software architecture pattern that separates an application into three main components: Model, View, and Controller, making it easier to manage and maintain the codebase. It also allows for the reusability of components and promotes a more modular approach to software development.
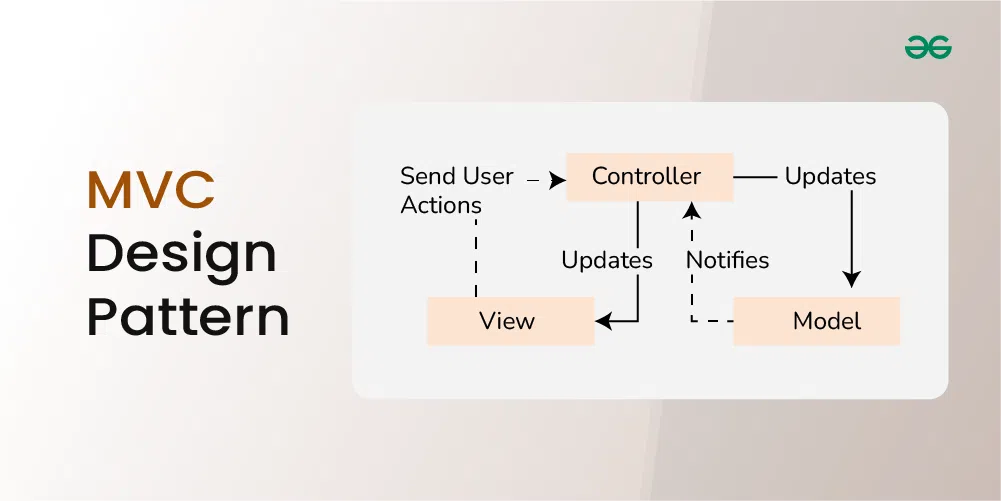
Important Topics for the MVC Design Pattern
What is the MVC Design Pattern?
The Model View Controller (MVC) design pattern specifies that an application consists of a data model, presentation information, and control information. The pattern requires that each of these be separated into different objects.
- The MVC pattern separates the concerns of an application into three distinct components, each responsible for a specific aspect of the application’s functionality.
- This separation of concerns makes the application easier to maintain and extend, as changes to one component do not require changes to the other components.Â
Components of the MVC Design Pattern
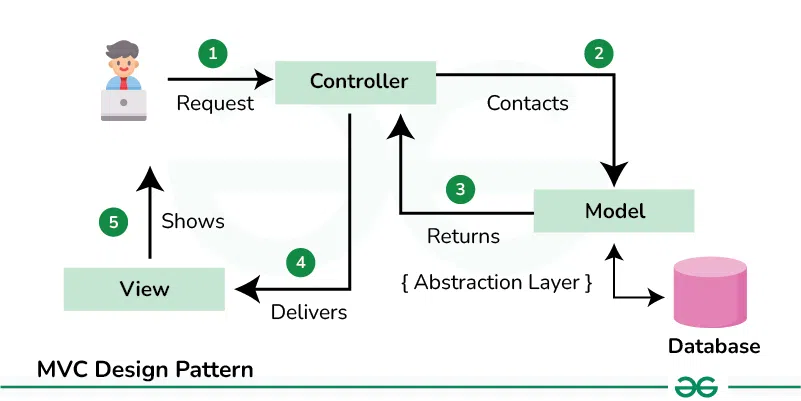
1. Model
The Model component in the MVC (Model-View-Controller) design pattern represents the data and business logic of an application. It is responsible for managing the application’s data, processing business rules, and responding to requests for information from other components, such as the View and the Controller.
2. View
Displays the data from the Model to the user and sends user inputs to the Controller. It is passive and does not directly interact with the Model. Instead, it receives data from the Model and sends user inputs to the Controller for processing.
3. Controller
Controller acts as an intermediary between the Model and the View. It handles user input and updates the Model accordingly and updates the View to reflect changes in the Model. It contains application logic, such as input validation and data transformation.
Communication between the components
This below communication flow ensures that each component is responsible for a specific aspect of the application’s functionality, leading to a more maintainable and scalable architecture
- User Interaction with View:
- The user interacts with the View, such as clicking a button or entering text into a form.
- View Receives User Input:
- The View receives the user input and forwards it to the Controller.
- Controller Processes User Input:
- The Controller receives the user input from the View.
- It interprets the input, performs any necessary operations (such as updating the Model), and decides how to respond.
- Controller Updates Model:
- The Controller updates the Model based on the user input or application logic.
- Model Notifies View of Changes:
- If the Model changes, it notifies the View.
- View Requests Data from Model:
- The View requests data from the Model to update its display.
- Controller Updates View:
- The Controller updates the View based on the changes in the Model or in response to user input.
- View Renders Updated UI:
- The View renders the updated UI based on the changes made by the Controller.
Example of the MVC Design Pattern
Below is the code of above problem statement using MVC Design Pattern:
Let’s break down into the component wise code:
.webp)
1. Model (Student class)
Represents the data (student’s name and roll number) and provides methods to access and modify this data.
Java
class Student {
private String rollNo;
private String name;
public String getRollNo() {
return rollNo;
}
public void setRollNo(String rollNo) {
this .rollNo = rollNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this .name = name;
}
}
|
2. View (StudentView class)
Represents how the data (student details) should be displayed to the user. Contains a method (printStudentDetails
) to print the student’s name and roll number.
Java
class StudentView {
public void printStudentDetails(String studentName, String studentRollNo) {
System.out.println( "Student:" );
System.out.println( "Name: " + studentName);
System.out.println( "Roll No: " + studentRollNo);
}
}
|
3. Controller (StudentController class)
Acts as an intermediary between the Model and the View. Contains references to the Model and View objects. Provides methods to update the Model (e.g., setStudentName
, setStudentRollNo
) and to update the View (updateView
).
Java
class StudentController {
private Student model;
private StudentView view;
public StudentController(Student model, StudentView view) {
this .model = model;
this .view = view;
}
public void setStudentName(String name) {
model.setName(name);
}
public String getStudentName() {
return model.getName();
}
public void setStudentRollNo(String rollNo) {
model.setRollNo(rollNo);
}
public String getStudentRollNo() {
return model.getRollNo();
}
public void updateView() {
view.printStudentDetails(model.getName(), model.getRollNo());
}
}
|
Complete code for the above example
Below is the complete code for the above example:
Java
class Student {
private String rollNo;
private String name;
public String getRollNo() {
return rollNo;
}
public void setRollNo(String rollNo) {
this .rollNo = rollNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this .name = name;
}
}
class StudentView {
public void printStudentDetails(String studentName, String studentRollNo) {
System.out.println( "Student:" );
System.out.println( "Name: " + studentName);
System.out.println( "Roll No: " + studentRollNo);
}
}
class StudentController {
private Student model;
private StudentView view;
public StudentController(Student model, StudentView view) {
this .model = model;
this .view = view;
}
public void setStudentName(String name) {
model.setName(name);
}
public String getStudentName() {
return model.getName();
}
public void setStudentRollNo(String rollNo) {
model.setRollNo(rollNo);
}
public String getStudentRollNo() {
return model.getRollNo();
}
public void updateView() {
view.printStudentDetails(model.getName(), model.getRollNo());
}
}
public class MVCPattern {
public static void main(String[] args) {
Student model = retriveStudentFromDatabase();
StudentView view = new StudentView();
StudentController controller = new StudentController(model, view);
controller.updateView();
controller.setStudentName( "Vikram Sharma" );
controller.updateView();
}
private static Student retriveStudentFromDatabase() {
Student student = new Student();
student.setName( "Lokesh Sharma" );
student.setRollNo( "15UCS157" );
return student;
}
}
|
Output
Student:
Name: Lokesh Sharma
Roll No: 15UCS157
Student:
Name: Vikram Sharma
Roll No: 15UCS157
|
Advantages of the MVC Design Pattern
- Separation of Concerns: MVC separates the different aspects of an application (data, UI, and logic), making the code easier to understand, maintain, and modify.
- Modularity: Each component (Model, View, Controller) can be developed and tested separately, promoting code reusability and scalability.
- Flexibility: Since the components are independent, changes to one component do not affect the others, allowing for easier updates and modifications.
- Parallel Development: Multiple developers can work on different components simultaneously, speeding up the development process.
- Code Reusability: The components can be reused in other parts of the application or in different projects, reducing development time and effort.
Disadvantages of the MVC Design Pattern
- Complexity: Implementing the MVC pattern can add complexity to the code, especially for simpler applications, leading to overhead in development.
- Learning Curve: Developers need to understand the concept of MVC and how to implement it effectively, which may require additional time and resources.
- Overhead: The communication between components (Model, View, Controller) can lead to overhead, affecting the performance of the application, especially in resource-constrained environments.
- Potential for Over-Engineering: In some cases, developers may over-engineer the application by adding unnecessary abstractions and layers, leading to bloated and hard-to-maintain code.
- Increased File Count: MVC can result in a larger number of files and classes compared to simpler architectures, which may make the project structure more complex and harder to navigate.
Share your thoughts in the comments
Please Login to comment...