Connectivity and Composition Patterns | Design Patterns for Cloud Native Applications
Last Updated :
03 Jan, 2024
In cloud-native applications, the architectural design becomes a critical aspect, defining the success of the entire solution. Connectivity and composition patterns are fundamental design principles that dictate how different components within a cloud-native application communicate and collaborate. Let’s delve deeper into the significance of these patterns.
Important Topics for Connectivity and Composition Patterns
1. Connectivity Patterns
Connectivity patterns are the fundamental principles that guide how services within a cloud-native application communicate.
These patterns are crucial for establishing efficient and reliable communication channels between various components.
2. Point-to-Point Communication
In the context of cloud-native applications, the point-to-point communication pattern serves as a straightforward interaction model between two services. Imagine it as a private conversation between specific entities where information is exchanged without the involvement of other services. This pattern is particularly well-suited for scenarios demanding simplicity and immediacy.
Example:
When one service needs specific data or functionality from another service directly, the point-to-point communication pattern ensures a focused and efficient exchange, akin to a direct dialogue between two entities.
2.1 Diagrammatic Representation of Above Problem:
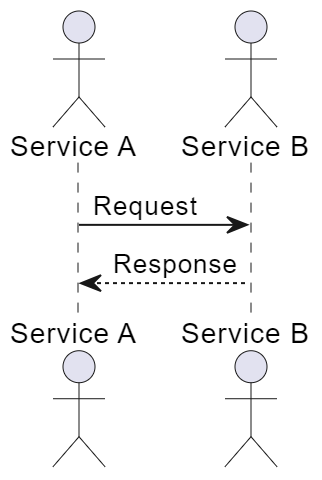
Python
/ / Service A
const data = await requestServiceBForData();
/ / Service B
async function requestServiceBForData() {
/ / Process and provide data
return data;
}
|
3. Publish-Subscribe Pattern
Contrasting with the direct nature of point-to-point communication, the publish-subscribe pattern introduces a more dynamic and flexible messaging model. Here, services take on the roles of publishers and subscribers.
Publishers broadcast messages into the system without any predefined knowledge of who will receive or “subscribe” to these messages. This decoupling of communication adds a layer of adaptability, allowing multiple services to express interest in specific types of information.
Picture it as a broadcasting system where information flows freely, and subscribers choose what channels they want to tune into. The publish-subscribe pattern excels in scenarios where diverse services need access to certain types of data without direct dependencies.
3.1 Digrammatic Representation of above Problem:
.jpg)
Python
/ / Service A - Publisher
publishMessage( 'eventTopic' , eventData);
/ / Service B - Subscriber
subscribeToEvent( 'eventTopic' , (data) = > {
/ / Process received data
});
|
4. Request-Reply Pattern
In situations where immediate feedback is crucial, the request-reply pattern comes into play. Here, a service initiates communication by sending a request to another service and then patiently awaits a response before proceeding further.
This synchronous communication model ensures that there is a direct and timely exchange of information.
Diagrammatic Representation of Request-Reply Pattern
.jpg)
In the above Diagram,
- Think of it as a conversation where one party poses a question, waits for the answer, and then continues based on that response.
- The request-reply pattern is vital in scenarios where services depend on real-time or near-real-time interactions, such as when a service needs specific data that must be retrieved promptly from another service.
- It establishes a clear and immediate line of communication, enabling seamless and time-sensitive exchanges between services in a cloud-native environment.
Python
/ / Service A
const response = await sendRequestToServiceB(requestData);
/ / Service B
async function sendRequestToServiceB(data) {
/ / Process data and provide a response
return response;
}
|
5. Composition Patterns
Composition patterns focus on orchestrating multiple services to perform complex operations. These patterns enable the creation of sophisticated functionalities by combining smaller, specialized services.
5.1 Aggregator Pattern
The aggregator pattern serves as a pivotal mechanism for consolidating data from multiple services, presenting a unified and comprehensive response.
Diagrammatic Representation of Aggregator Pattern
.png)
In the above diagram,
- Think of it as a master conductor orchestrating a harmonious symphony where each service plays its unique part, contributing essential information. This pattern proves invaluable in scenarios where a holistic view is required, and data must be gathered from diverse sources.
- For instance, in an e-commerce application, the aggregator pattern could be employed to compile product information from various services, ensuring that the user receives a unified presentation of details such as pricing, availability, and reviews.
- By seamlessly merging data from different sources, the aggregator pattern enhances efficiency and provides users with a consolidated and user-friendly experience.
Python
/ / Aggregator Service
const aggregatedData = await aggregateDataFromServices();
/ / Service A
async function getDataFromServiceA() {
/ / Retrieve and process data
return data;
}
/ / Service B
async function getDataFromServiceB() {
/ / Retrieve and process data
return data;
}
/ / Aggregator
async function aggregateDataFromServices() {
const dataA = await getDataFromServiceA();
const dataB = await getDataFromServiceB();
/ / Combine and return aggregated data
return { dataA, dataB };
}
|
5.2 Chain of Responsibility Pattern
The chain of responsibility pattern introduces a flexible way to handle requests in a sequential and organized manner.
.png)
In the above Diagram,
- Imagine a relay race where a baton (request) is passed along a chain of runners (handlers), with each runner having the option to process the baton or pass it to the next runner.
- This pattern accommodates a dynamic and extensible approach to request handling. Each handler encapsulates a specific set of logic or responsibility, and the request traverses through these handlers until it reaches one that can effectively process it.
- This sequential passing of the request allows for modular and scalable processing. For example, in a payment processing system, the chain of responsibility pattern might be employed to handle different stages of payment verification and authorization.
- If one handler cannot process the request, it seamlessly passes it to the next in line, ensuring a streamlined and adaptable approach to request processing.
Below is the implementation of the above pattern:
Python
/ / Handler Interface
class Handler {
constructor(successor = null) {
this.successor = successor;
}
handleRequest(request) {
if (this.successor) {
this.successor.handleRequest(request);
}
}
}
/ / Concrete Handlers
class ConcreteHandlerA extends Handler {
handleRequest(request) {
/ / Process request or pass to the next handler
super .handleRequest(request);
}
}
class ConcreteHandlerB extends Handler {
handleRequest(request) {
/ / Process request or pass to the next handler
super .handleRequest(request);
}
}
/ / Client
const handlerChain = new ConcreteHandlerA(new ConcreteHandlerB());
handlerChain.handleRequest(request);
|
5.3 Workflow Pattern
The workflow pattern acts as the choreographer of cloud-native application operations, defining a structured series of connected steps or activities to accomplish a specific goal.
Example:
- Picture it as a carefully choreographed dance where each step seamlessly flows into the next, creating a cohesive performance.
- This pattern is particularly beneficial for orchestrating complex tasks that involve the coordinated execution of multiple services. In a healthcare application, for instance, the workflow pattern could be utilized to manage the patient admission process.
- It defines a systematic sequence of steps, such as patient registration, medical history verification, and insurance validation, ensuring that each activity is executed in the predefined order.
- The workflow pattern brings order and predictability to intricate processes, facilitating the systematic execution of services in cloud-native applications.
A very simple example for demonstration of this workflow pattern is given below :
Python
/ / Workflow Service
async function executeWorkflow() {
await executeStep1();
await executeStep2();
await executeStep3();
}
/ / Individual Services
async function executeStep1() {
/ / Perform step 1 tasks
}
async function executeStep2() {
/ / Perform step 2 tasks
}
async function executeStep3() {
/ / Perform step 3 tasks
}
|
6. Technologies for Implementing Service Composition Patterns
Now, let’s explore some of the technologies commonly used to implement service composition patterns.
6.1 Kubernetes
Kubernetes stands as a robust and highly efficient container orchestration platform, acting as the maestro for containerized applications.
How Kubernetes is helpfull for Cloud Native Application
- It introduces automation into the deployment, scaling, and management of these containerized applications, marking it as a pivotal technology for orchestrating services in a cloud-native environment.
- Picture Kubernetes as a seasoned conductor directing a symphony of containers, ensuring seamless coordination and harmony in the deployment and scaling of applications.
- Its ability to automate complex tasks simplifies the management of services, making it a cornerstone technology for container orchestration in cloud-native architectures.
6.2 Docker
Docker, renowned in the realm of containerization, provides a transformative solution for applications, enhancing their portability and scalability. Containers, the core of Docker’s innovation, encapsulate services along with their dependencies, ensuring consistent performance across diverse environments.
How Docker is helpfull for Cloud Native Application
- Think of Docker as a versatile and standardized packaging system, allowing applications to be bundled with everything they need to run, from code to libraries.
- This encapsulation ensures that an application operates uniformly regardless of the underlying infrastructure.
- Docker’s contribution to the world of cloud-native applications lies in its ability to simplify the deployment and scaling of services while maintaining a high level of consistency and efficiency.
6.3 Apache Kafka
Apache Kafka emerges as a distributed event streaming platform, playing a pivotal role in implementing crucial communication patterns like publish-subscribe and message queue.
How Apache Kafka is helpfull for Cloud Native Application
- Visualize Apache Kafka as a central nervous system for data streaming, where events flow seamlessly and reliably between services. It excels in providing a foundation for real-time data streaming, making it an indispensable technology for scenarios requiring efficient and fault-tolerant communication.
- In the cloud-native landscape, Apache Kafka serves as the backbone for building resilient and scalable architectures, facilitating the seamless exchange of information between services.
6.4 GraphQL
GraphQL, a dynamic query language for APIs, introduces a paradigm shift in how services communicate. It facilitates efficient and flexible communication by allowing clients to request precisely the data they need, minimizing over-fetching of information.
How GraphQL is helpfull for Cloud Native Application
- Imagine GraphQL as a personalized concierge for data requests, where clients specify their data requirements, and GraphQL efficiently fetches and delivers only the requested information.
- This level of granularity in data retrieval enhances the efficiency of communication between services, making GraphQL a preferred choice for optimizing data transfer in cloud-native architectures.
- Its flexibility and adaptability contribute to a more streamlined and responsive interaction between services in modern application development.
7. Conclusion
Connectivity and composition patterns are indispensable in the cloud-native application landscape. They empower architects and developers to build scalable and flexible systems. Leveraging these patterns, along with appropriate technologies, ensures that our cloud-native applications can adapt to evolving requirements with ease. As we embark on our cloud-native journey, we should consider the connectivity and composition patterns that best align with our application’s needs.
Share your thoughts in the comments
Please Login to comment...