Largest number in BST which is less than or equal to N
Last Updated :
15 Feb, 2023
We have a binary search tree and a number N. Our task is to find the greatest number in the binary search tree that is less than or equal to N. Print the value of the element if it exists otherwise print -1.
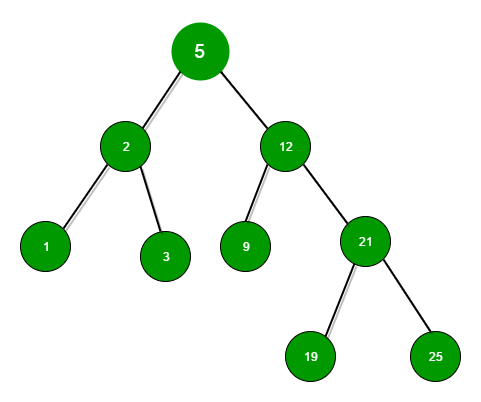
Examples:
For the above given binary search tree-
Input : N = 24
Output :result = 21
(searching for 24 will be like-5->12->21)
Input : N = 4
Output : result = 3
(searching for 4 will be like-5->2->3)
We follow recursive approach for solving this problem. We start searching for element from root node. If we reach a leaf and its value is greater than N, element does not exist so return -1. Else if node’s value is less than or equal to N and right value is NULL or greater than N, then return the node value as it will be the answer.
Otherwise if node’s value is greater than N, then search for the element in the left subtree else search for the element in the right subtree by calling the same function by passing the left or right values accordingly.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int key;
Node *left, *right;
};
Node* newNode( int item)
{
Node* temp = new Node;
temp->key = item;
temp->left = temp->right = NULL;
return temp;
}
Node* insert(Node* node, int key)
{
if (node == NULL)
return newNode(key);
if (key < node->key)
node->left = insert(node->left, key);
else if (key > node->key)
node->right = insert(node->right, key);
return node;
}
int findMaxforN(Node* root, int N)
{
if (root == NULL)
return -1;
if (root->key == N)
return N;
else if (root->key < N) {
int k = findMaxforN(root->right, N);
if (k == -1)
return root->key;
else
return k;
}
else if (root->key > N)
return findMaxforN(root->left, N);
}
int main()
{
int N = 4;
Node* root = insert(root, 25);
insert(root, 2);
insert(root, 1);
insert(root, 3);
insert(root, 12);
insert(root, 9);
insert(root, 21);
insert(root, 19);
insert(root, 25);
printf ( "%d" , findMaxforN(root, N));
return 0;
}
|
Java
class GfG {
static class Node {
int key;
Node left, right;
}
static Node newNode( int item)
{
Node temp = new Node();
temp.key = item;
temp.left = null ;
temp.right = null ;
return temp;
}
static Node insert(Node node, int key)
{
if (node == null )
return newNode(key);
if (key < node.key)
node.left = insert(node.left, key);
else if (key > node.key)
node.right = insert(node.right, key);
return node;
}
static int findMaxforN(Node root, int N)
{
if (root == null )
return - 1 ;
if (root.key == N)
return N;
else if (root.key < N) {
int k = findMaxforN(root.right, N);
if (k == - 1 )
return root.key;
else
return k;
}
else if (root.key > N)
return findMaxforN(root.left, N);
return - 1 ;
}
public static void main(String[] args)
{
int N = 4 ;
Node root = null ;
root = insert(root, 25 );
insert(root, 2 );
insert(root, 1 );
insert(root, 3 );
insert(root, 12 );
insert(root, 9 );
insert(root, 21 );
insert(root, 19 );
insert(root, 25 );
System.out.println(findMaxforN(root, N));
}
}
|
Python3
class newNode:
def __init__( self , data):
self .key = data
self .left = None
self .right = None
def insert(node, key):
if node = = None :
return newNode(key)
if key < node.key:
node.left = insert(node.left, key)
elif key > node.key:
node.right = insert(node.right, key)
return node
def findMaxforN(root, N):
if root = = None :
return - 1
if root.key = = N:
return N
elif root.key < N:
k = findMaxforN(root.right, N)
if k = = - 1 :
return root.key
else :
return k
elif root.key > N:
return findMaxforN(root.left, N)
if __name__ = = '__main__' :
N = 4
root = None
root = insert(root, 25 )
insert(root, 2 )
insert(root, 1 )
insert(root, 3 )
insert(root, 12 )
insert(root, 9 )
insert(root, 21 )
insert(root, 19 )
insert(root, 25 )
print (findMaxforN(root, N))
|
C#
using System;
class GFG
{
class Node
{
public int key;
public Node left, right;
}
static Node newNode( int item)
{
Node temp = new Node();
temp.key = item;
temp.left = null ;
temp.right = null ;
return temp;
}
static Node insert(Node node, int key)
{
if (node == null )
return newNode(key);
if (key < node.key)
node.left = insert(node.left, key);
else if (key > node.key)
node.right = insert(node.right, key);
return node;
}
static int findMaxforN(Node root, int N)
{
if (root == null )
return -1;
if (root.key == N)
return N;
else if (root.key < N)
{
int k = findMaxforN(root.right, N);
if (k == -1)
return root.key;
else
return k;
}
else if (root.key > N)
return findMaxforN(root.left, N);
return -1;
}
public static void Main(String[] args)
{
int N = 4;
Node root = null ;
root = insert(root, 25);
insert(root, 2);
insert(root, 1);
insert(root, 3);
insert(root, 12);
insert(root, 9);
insert(root, 21);
insert(root, 19);
insert(root, 25);
Console.WriteLine(findMaxforN(root, N));
}
}
|
Javascript
<script>
class Node
{
constructor(){
this .key = 0;
this .left = null , this .right = null ;
}
}
function newNode(item)
{
var temp = new Node();
temp.key = item;
temp.left = null ;
temp.right = null ;
return temp;
}
function insert(node , key) {
if (node == null )
return newNode(key);
if (key < node.key)
node.left = insert(node.left, key);
else if (key > node.key)
node.right = insert(node.right, key);
return node;
}
function findMaxforN(root , N)
{
if (root == null )
return -1;
if (root.key == N)
return N;
else if (root.key < N)
{
var k = findMaxforN(root.right, N);
if (k == -1)
return root.key;
else
return k;
}
else if (root.key > N)
return findMaxforN(root.left, N);
return -1;
}
var N = 4;
var root = null ;
root = insert(root, 25);
insert(root, 2);
insert(root, 1);
insert(root, 3);
insert(root, 12);
insert(root, 9);
insert(root, 21);
insert(root, 19);
insert(root, 25);
document.write(findMaxforN(root, N));
</script>
|
Time Complexity: O(h), where h is height of BST.
Auxiliary Space: O(h), The extra space is used in recursion call stack.
Iterative Solution: Below is an iterative solution and it does not require extra space for recursion call stack.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int key;
Node *left, *right;
};
Node* newNode( int item)
{
Node* temp = new Node;
temp->key = item;
temp->left = temp->right = NULL;
return temp;
}
Node* insert(Node* node, int key)
{
if (node == NULL)
return newNode(key);
if (key < node->key)
node->left = insert(node->left, key);
else if (key > node->key)
node->right = insert(node->right, key);
return node;
}
void findMaxforN(Node* root, int N)
{
while (root != NULL && root->right != NULL) {
if (N > root->key && N >= root->right->key)
root = root->right;
else if (N < root->key)
root = root->left;
else
break ;
}
if (root == NULL || root->key > N)
cout << -1;
else
cout << root->key;
}
int main()
{
int N = 50;
Node* root = insert(root, 5);
insert(root, 2);
insert(root, 1);
insert(root, 3);
insert(root, 12);
insert(root, 9);
insert(root, 21);
insert(root, 19);
insert(root, 25);
findMaxforN(root, N);
return 0;
}
|
Java
import java.util.*;
class GFG{
static class Node {
int key;
Node left, right;
};
static Node newNode( int item)
{
Node temp = new Node();
temp.key = item;
temp.left = temp.right = null ;
return temp;
}
static Node insert(Node node, int key)
{
if (node == null )
return newNode(key);
if (key < node.key)
node.left = insert(node.left, key);
else if (key > node.key)
node.right = insert(node.right, key);
return node;
}
static void findMaxforN(Node root, int N)
{
while (root != null && root.right != null ) {
if (N > root.key && N >= root.right.key)
root = root.right;
else if (N < root.key)
root = root.left;
else
break ;
}
if (root == null || root.key > N)
System.out.print(- 1 );
else
System.out.print(root.key);
}
public static void main(String[] args)
{
int N = 50 ;
Node root = insert( null , 5 );
insert(root, 2 );
insert(root, 1 );
insert(root, 3 );
insert(root, 12 );
insert(root, 9 );
insert(root, 21 );
insert(root, 19 );
insert(root, 25 );
findMaxforN(root, N);
}
}
|
Python3
class newNode:
def __init__( self , data):
self .key = data
self .left = None
self .right = None
def insert(node, key):
if (node = = None ):
return newNode(key)
if (key < node.key):
node.left = insert(node.left, key)
elif (key > node.key):
node.right = insert(node.right, key)
return node
def findMaxforN(root, N):
while (root ! = None and root.right ! = None ):
if (N > root.key and N > = root.right.key):
root = root.right
elif (N < root.key):
root = root.left
else :
break
if (root = = None or root.key > N):
print ( - 1 )
else :
print (root.key)
if __name__ = = '__main__' :
N = 50
root = None
root = insert(root, 5 )
insert(root, 2 )
insert(root, 1 )
insert(root, 3 )
insert(root, 12 )
insert(root, 9 )
insert(root, 21 )
insert(root, 19 )
insert(root, 25 )
findMaxforN(root, N)
|
C#
using System;
public class GFG {
public class Node {
public int key;
public Node left, right;
};
static Node newNode( int item) {
Node temp = new Node();
temp.key = item;
temp.left = temp.right = null ;
return temp;
}
static Node insert(Node node, int key) {
if (node == null )
return newNode(key);
if (key < node.key)
node.left = insert(node.left, key);
else if (key > node.key)
node.right = insert(node.right, key);
return node;
}
static void findMaxforN(Node root, int N) {
while (root != null && root.right != null ) {
if (N > root.key && N >= root.right.key)
root = root.right;
else if (N < root.key)
root = root.left;
else
break ;
}
if (root == null || root.key > N)
Console.Write(-1);
else
Console.Write(root.key);
}
public static void Main(String[] args) {
int N = 50;
Node root = insert( null , 5);
insert(root, 2);
insert(root, 1);
insert(root, 3);
insert(root, 12);
insert(root, 9);
insert(root, 21);
insert(root, 19);
insert(root, 25);
findMaxforN(root, N);
}
}
|
Javascript
<script>
class Node
{
constructor(data)
{
this .key=data;
this .left= this .right= null ;
}
}
function insert(node, key)
{
if (node == null )
return new Node(key)
if (key < node.key)
node.left = insert(node.left, key)
else if (key > node.key)
node.right = insert(node.right, key)
return node
}
function findMaxforN(root, N)
{
while (root != null && root.right != null )
{
if (N > root.key && N >= root.right.key)
root = root.right
else if (N < root.key)
root = root.left
else
break
}
if (root == null || root.key > N)
document.write(-1)
else
document.write(root.key)
}
let N = 50;
let root = null ;
root=insert(root, 2)
root=insert(root, 1)
root=insert(root, 3)
root=insert(root, 12)
root=insert(root, 9)
root=insert(root, 21)
root=insert(root, 19)
root=insert(root, 25)
findMaxforN(root, N)
</script>
|
Time Complexity: O(h), where h is height of BST.
Auxiliary Space: O(1), As constant extra space is used.
Share your thoughts in the comments
Please Login to comment...