Interacting with Webpage – Selenium Python
Last Updated :
18 Aug, 2022
Selenium’s Python Module is built to perform automated testing with Python. Selenium Python bindings provide a simple API to write functional/acceptance tests using Selenium WebDriver. To open a webpage using Selenium Python, checkout – Navigating links using get method – Selenium Python. Just being able to go to places isn’t terribly useful. What we’d really like to do is to interact with the pages, or, more specifically, the HTML elements within a page. First of all, we need to find one. WebDriver offers a number of ways to find elements. For example, given an element defined as:
html
< input type = "text" name = "passwd" id = "passwd-id" />
|
To find an element one needs to use one of the locating strategies, For example,
element = driver.find_element(By.ID,"passwd-id")
element = driver.find_element(By.NAME, "passwd")
element = driver.find_element(By.XPATH, "//input[@id='passwd-id']")
Also, to find multiple elements, we can use
elements = driver.find_elements(By.NAME, "passwd")
One can also look for a link by its text, but be careful! The text must be an exact match! One should also be careful when using XPATH in WebDriver. If there’s more than one element that matches the query, then only the first will be returned. If nothing can be found, a NoSuchElementException will be raised.
WebDriver has an “Object-based” API, we represent all types of elements using the same interface. This means that although one may see a lot of possible methods one could invoke when one hits IDE’s auto-complete key combination, not all of them will make sense or be valid. To check all methods, checkout Locator Strategies – Selenium Python
So after getting an element what next? One might want to enter text into a field, for example,
element.send_keys("some text")
One can simulate pressing the arrow keys by using the “Keys” class:
element.send_keys(" and some", Keys.ARROW_DOWN)
Also note, that it is possible to call send_keys on any element, which makes it possible to test keyboard shortcuts such as those used on Gmail. One can easily clear the contents of a text field or textarea with the clear method:
element.clear()
Project Example: Let us try to search for something automatically at geeksforgeeks
Program
Python3
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Firefox()
element = driver.find_element(By. ID , "gsc-i-id1" )
element.send_keys( "Arrays" )
|
Output:
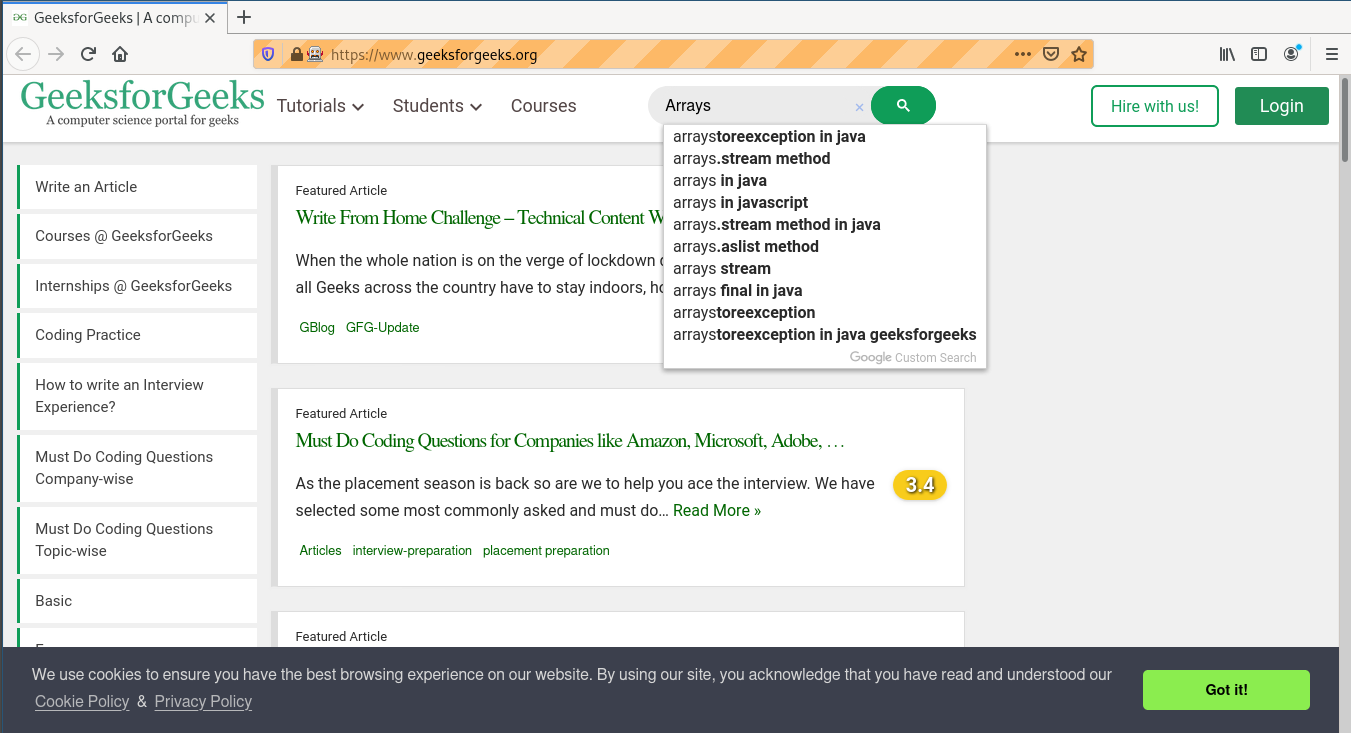
Share your thoughts in the comments
Please Login to comment...