Implementing GraphQL Queries using React Hooks & Apollo Client
Last Updated :
03 Apr, 2024
Implementing GraphQL queries with React Hooks and Apollo Client involves setting up Apollo Client in your React application, defining GraphQL queries using useQuery
hook, and accessing data from the GraphQL API in a declarative way. With Apollo Client, React Hooks like useQuery
simplifies the process of fetching and managing data from a GraphQL server, offering a more efficient and organized approach to data fetching in React applications.
Prerequisites
Steps to Implement GraphQL Queries using Hooks & Apollo
Step 1: Create a reactJS application by using this command
npx create-react-app graphql
Step 2: Navigate to project directory
cd graphql
Step 3: Install the necessary packages/libraries in your project using the following commands.
npm install @apollo/client graphql
Project Structure:
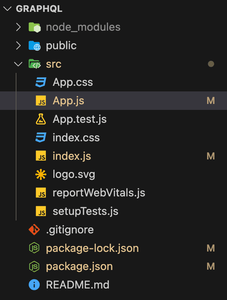
The updated dependencies in package.json file will look like:
"dependencies": {
"@apollo/client": "^3.9.6",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"graphql": "^16.8.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Approach to Implement GraphQL Queries using Hooks & Apollo:
- Create an Apollo Client instance in
apolloClient.js
with the GraphQL endpoint URL and an InMemoryCache. - Use the
gql
tag from @apollo/client
to define your GraphQL query in App.js
, such as GET_COUNTRY
in this example. - Utilize the
useQuery
hook provided by Apollo Client in App.js
to fetch data based on the defined GraphQL query. - Check for loading state and errors while the query is being executed, displaying appropriate messages or components in UI.
- Once data is fetched successfully, render the desired components with the retrieved data, ensuring a smooth and responsive user experience.
Example: Illustration to Implement GraphQL Queries with React Hooks and Apollo Client.
CSS
/* index.css */
.App-header {
font-size: 50px;
display: flex;
justify-content: center;
align-items: center;
}
Javascript
//apolloClient.js
import { ApolloClient, InMemoryCache } from '@apollo/client';
const client = new ApolloClient({
uri: 'https://countries.trevorblades.com/graphql',
cache: new InMemoryCache()
});
export default client;
JavaScript
//App.js
import './App.css';
import { gql, useQuery } from '@apollo/client';
const GET_COUNTRY = gql`
query {
country(code: "BR") {
name
currency
}
}
`;
function App() {
const { loading, error, data } = useQuery(GET_COUNTRY);
return (
<div className="App">
<header className="App-header">
{loading && <p>Loading...</p>}
{error && <p>Error: {error.message}</p>}
{data && (
<p>
{data.country.name} - {data.country.currency}
</p>
)}
</header>
</div>
);
}
export default App;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import { ApolloProvider } from '@apollo/client';
import client from './apolloClient';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<ApolloProvider client={client}>
<App />
</ApolloProvider>
</React.StrictMode>
);
Step to Run Application: Run the application using the following command from the root directory of the project
npm start
Output: Your project will be shown in the URL http://localhost:3000/
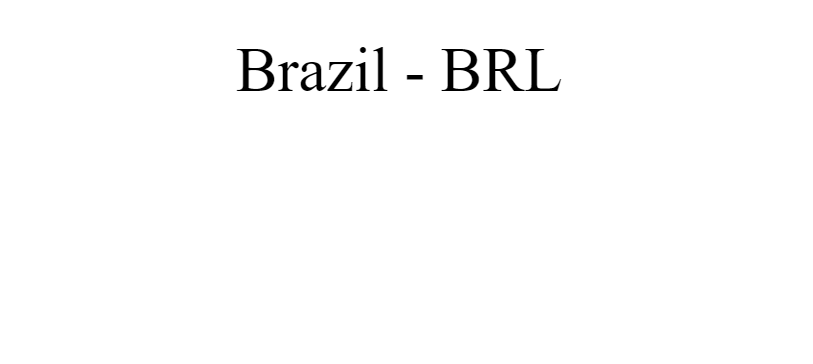
Conclusion:
In this article, we learned about GraphQL queries, and how they can be useful in querying and fetching just the data that the client wants from the server. We also learnt about how we can fetch the data and execute the GraphQL queries in react using the “useQuery” hook, and how we can display the fetched data in the UI.
Share your thoughts in the comments
Please Login to comment...