What are queries in React Testing Library ?
Last Updated :
22 Mar, 2024
In this article, we explain the concept of queries in the React Testing Library. Ensuring that components behave as expected when developing applications with React is crucial. One powerful tool for testing React components is the React Testing Library. At the heart of the React Testing Library lies the concept of queries.
What is React Testing Library ?
React Testing Library is a testing utility for React that allows developers to write tests that resemble how users interact with applications. It focuses on testing components in a way that promotes best practices and encourages developers to write more robust and maintainable tests.
What are Queries?
In the context of React Testing Library, queries are functions that are used to search for elements rendered by React components. These functions emulate how users interact with the UI, making them a valuable asset for testing user interactions and behaviors.
Features:
React Testing Library offers several features that make it a valuable tool for testing React applications:
- User-Centric Testing: Emphasizes testing components based on how users interact with the UI, leading to more reliable tests that closely resemble real-world scenarios.
- Simple API: Provides a straightforward API for querying elements within components, making it easy to write and maintain tests.
- Accessibility Testing: Includes queries like getByRole() that facilitate testing for accessibility features, ensuring components are semantically correct and accessible to all users.
Steps to implement Testing in React App:
Step 1: create a new React application using Create React App
npx create-react-app app
cd app
Step 2: Install React Testing Library and any additional testing dependencies:
npm install --save-dev @testing-library/react @testing-library/jest-dom
Step 3: add MyComponent.js and MyComponent.test.js in the src directory.
Example: Below is the code example:
JavaScript
// MyComponent.js
import React from 'react';
const MyComponent = ({ onClick }) => {
return (
<div>
<button onClick={onClick}>
Click Me
</button>
</div>
);
};
export default MyComponent;
JavaScript
// MyComponent.test.js
import React from 'react';
import {
render,
screen,
fireEvent
} from '@testing-library/react';
import MyComponent from './MyComponent';
// Test to ensure that the button renders with the correct text
test('renders a button with correct text', () => {
render(<MyComponent />);
const buttonElement =
screen.getByRole('button', { name: /click me/i });
expect(buttonElement).toBeInTheDocument();
});
// Test to ensure that clicking the button triggers the provided onClick function
test('clicking button triggers onClick function', () => {
// Mock function to pass as onClick prop
const onClickMock = jest.fn();
// Render MyComponent with the mocked onClick function
render(<MyComponent onClick={onClickMock} />);
// Find the button element
const buttonElement =
screen.getByRole('button', { name: /click me/i });
// Simulate a click on the button
fireEvent.click(buttonElement);
// Expect the onClickMock function to have been called once
expect(onClickMock).toHaveBeenCalledTimes(1);
});
Step 4: Execute your tests using a test runner like Jest
npm test
Output:
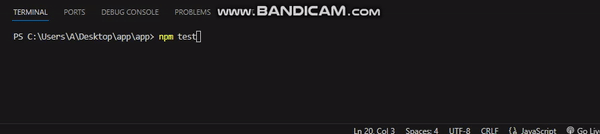
Conclusion:
Queries are fundamental to React Testing Library as they enable developers to search for elements within rendered components, facilitating effective testing of user interactions and behaviors
Share your thoughts in the comments
Please Login to comment...