Implementing Infinite Scrolling with React Hooks
Last Updated :
02 May, 2024
Infinite Scroll is a crucial aspect of many web applications, ensuring that users have access to infinite content while keeping the user experience in mind. In this article, we’ll explore how to implement infinite scroll flows in a React application using React Hooks.
Prerequisites:
Infinite Scroll Using React Components and React Hooks
React infinite scroll component provides a simple way to add infinite scroll functionality to your React applications. With React Hooks, managing the use states becomes more straightforward and efficient. This guide will cover setting up react-infinite-scroll, integrating it with a React application using Hooks, and handling related tasks.
Approach:
To implement infinite scrolling with React Hooks follow these steps:
- Create a React Application.
- Install Dependencies: Install the necessary packages using npm or yarn.
- Install react infinite scroll component.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app react-infinite-scroll
Step 2: After creating your project folder i.e. react-infinite-scroll, move to it using the following command:
cd react-infinite-scroll
Step 3: Install the necessary package in your application using the following command.
npm i react-infinite-scroll-component
Step 4: After setting up react environment on your system, we can start by creating an App.js file and create a directory by the name of components in which we will write our desired function.
Project Structure:
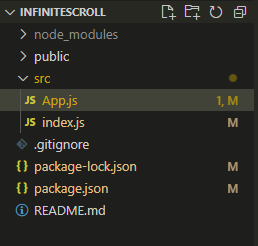
Project Structure
The updated dependencies in package.json file will look like:
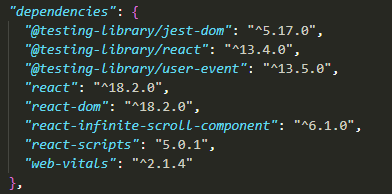
dependencies
Implementation:
- We have an empty react.js project that’s showing nothing right now . For the infinite scroll we will be using react infinite scroll component npm package
- In our project once that is installed we can go ahead and import infinite scroll from react infinite score component and start using it.
import "./App.css";
import InfiniteScroll from "react-infinite-scroll-component"
function App() {
return (
<div className="App">
<InfiniteScroll >
<p><b>INFINITE SCROLL</b></p>
</InfiniteScroll>
</div>
);
}
export default App;
- Inside that we will be rendering our content. so for that we will use a state and add some array content in that so that we can loop on that and add some content that is scrollable. So let’s add a state ,we can say dataSource and setDataSource is equal to useState and by by default it may be empty. Here we have created a few elements using an array. Now here in inside the infiniteScroll we will loop on that Array. so we can say dataSource.map and it will be giving us the item and the index. Inside it we can return anything here such as posts or videos or reals or blogs etc.. So for now we will return the div and inside that we can say “Your Content here” followed by the div number.
- InfiniteScroll need a dataLength so we can say data’s its dataLength is equal to length of dataSource.
function App() {
const [dataSource,setDataSource] = useState(Array.from({length:20}))
return (
<div className="App">
<InfiniteScroll dataLength={dataSource.length}>
<p><b>INFINITE SCROLL</b></p>
{dataSource.map((item,index)=>{
return <div>Your Content Here {index+1}</div>
})}
</InfiniteScroll>
</div>
);
}
- Save it. Here we go it has added the div’s. Let’s add some styling on Div’s. You can play around the styling as you like.
//App.css
.App {
/* Styles for the main App container */
display: flex;
flex-direction: column;
}
.container-box {
/* Styles for the individual content boxes */
border: 1px solid green;
margin: 10px;
padding: 10px;
}
- So we have 20 records i.e. div 1 to div 20. Right now if we scroll ,it’s not adding other contents or we can say it’s not an infinite score currently. Now we will add some more Props in InfiniteScroll component.
- next Prop: The “next” will be called when user has reached to the end of the scroll and it will call our this next function where we will be adding more content inside our state.
next = {fetchMoreData}
Fetch more data is defined as:
const fetchMoreData=()=>{
//MAKING THE API CALL HERE
setTimeout(()=>{
setDataSource(dataSource.concat(Array.from({length:10})))
}, 500);
}
- Here we will be making API calls to our servers , based on the pagination . so on the first page we are fetching 20 records and on the next page we are fetching next 10 records. So based on that we will be making the API calls here.
- For this article to mimic the behavior we will be using the setTimeout(). we can say after 500 milliseconds make an API call . Here we will be setting the dataSource or we can say updating the dataSource and what we’ll be doing here is we can say as soon as user will reaches to the bottom they will call this fetchMoreData() function and inside that we are waiting for 500 milliseconds and after that we will be updating the data source so that it will increase the dataLength or the content inside the infiniteScroll by 10 more records. also there is another prop to make the API calls that is “hasMore”. We will need to set it to tell the infiniteScroll that we have some more data so tell us when the user is on the bottom of the page. For that we will again use a state hasMore and set hasMore to true via using state. We know that we have some more data inside .
- So now if we scroll and do to the bottom it is adding more more records right now.
- We are going to the bottom so we can show the loading indicator that as well. That will be inside the loader prop. Here we can return any component, so for simplicity we will return a p tag saying “loading…”
- As soon as we reach to the bottom of the scroll it shows loading and then after 500 millisecond it added more records.
Example: To demonstrate implementing infinite scrolling with react Hooks.
CSS
//src/ App.css
.App {
/* Styles for the main App container */
display: flex;
flex-direction: column;
}
.container-box {
/* Styles for the individual content boxes */
border: 1px solid green;
margin: 10px;
padding: 10px;
}
JavaScript
//src/ App.js
import { useState } from "react";
import "./App.css";
import InfiniteScroll from "react-infinite-scroll-component"
function App() {
const [dataSource, setDataSource] = useState(Array.from({ length: 20 }))
const [hasMore, setHasMore] = useState(true)
const fetchMoreData = () => {
//MAKING THE API CALL HERE
setTimeout(() => {
setDataSource(dataSource.concat(Array.from({ length: 10 })))
}, 500);
}
return (
<div className="App">
<InfiniteScroll dataLength={dataSource.length}
next={fetchMoreData}
hasMore={hasMore}
loader={<p>Loading...</p>}
>
<p><b>INFINITE SCROLL</b></p>
{dataSource.map((item, index) => {
return <div key={index}
className="container-box" >Your Content Here {index + 1}</div>
})}
</InfiniteScroll>
</div>
);
}
export default App;
JavaScript
//src/ index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
Syntanx to run the application:
npm start
Output:
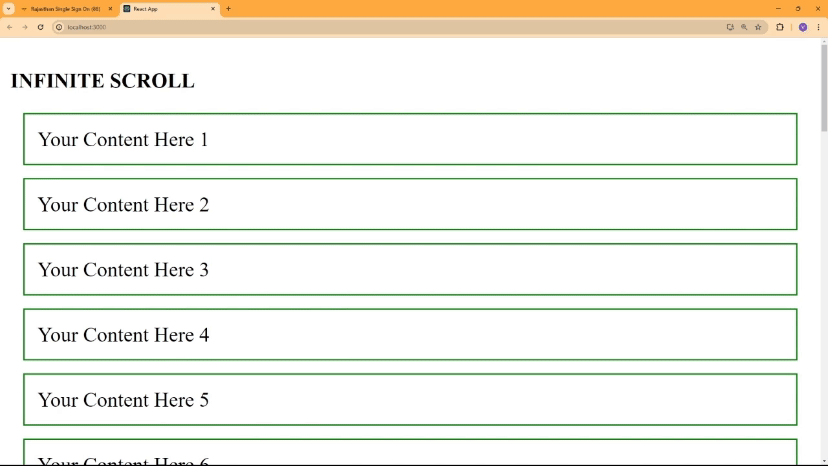
Share your thoughts in the comments
Please Login to comment...