Implementation of Dark Mode in React using Redux Toolkit
Last Updated :
16 Nov, 2023
In this article we are going to implement Dark and Light mode toggle button using React JS and Redux Toolkit. Dark Mode is also known as night mode. It is just a dark theme where mostly background colors will turn into dark and text color will turn into light.
Preview of final output: Let us have a look at how the final output will look like.
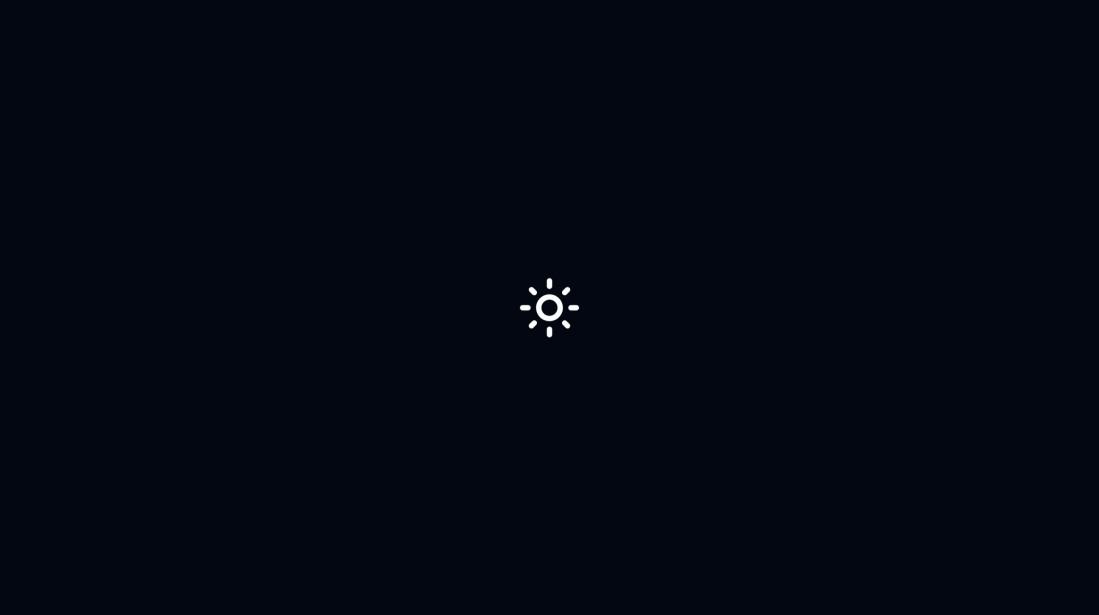
Prerequisites
Approach
Steps to implement this functionality are given below –
- Create three folders pages, reducer, slices in src directory and create Home.jsx, index.js, modeSlice in it respectively.
- Implement store inside Index.js code in the following example.
- Implement reducer in modeSlice file required code is provided in the example.
- Implement combineReducer in Index.js file present in reducer directory required code is given in the example.
- Now we will create a page (Home.jsx) where we will make two icons and toggle background color.
- Last step call Home component in App.js file.
Steps to Create & Configure React App with Redux Toolkit
Step 1: Create a react app using command “npx create-react-app app-name”.
npx create-react-app app-name
Step 2 : Install redux and redux toolkit using following commands
npm install react-redux @reduxjs/toolkit
Step 3: Add Tailwind CSS using CDN link given below in your index.html file.
<script src="https://cdn.tailwindcss.com"></script>
The updated Dependencies in package.json file will look like:
"dependencies": {
"@reduxjs/toolkit": "^1.9.7",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-icons": "^4.12.0",
"react-redux": "^8.1.3",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Project Structure:
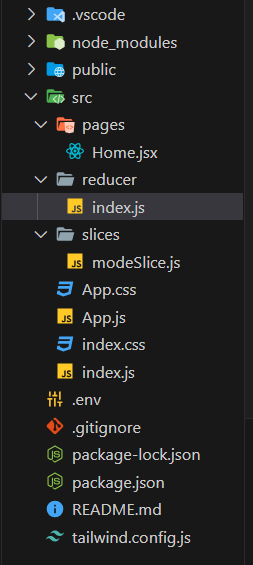
directory structure
Example: It contains implementation of above steps.
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
import App from "./App" ;
import "./index.css" ;
import { Provider } from "react-redux" ;
import rootReducer from "./reducer/index.js" ;
import { configureStore } from "@reduxjs/toolkit"
const store = configureStore({
reducer: rootReducer,
})
const root = ReactDOM.createRoot(document.getElementById( "root" ));
root.render(
<React.StrictMode>
<Provider store={store}>
<App />
</Provider>
</React.StrictMode>
);
|
Javascript
import { createSlice } from "@reduxjs/toolkit" ;
const initialState = {
darkMode: localStorage.getItem( "darkMode" )
? JSON.parse(localStorage.getItem( "darkMode" )) : false
}
const modeSlice = createSlice({
name: "mode" ,
initialState: initialState,
reducers: {
setMode(state, value) {
state.darkMode = value.payload
localStorage.setItem( 'darkMode' , JSON.stringify(state.darkMode));
}}})
export const { setMode } = modeSlice.actions;
export default modeSlice.reducer;
|
Javascript
import { combineReducers } from "@reduxjs/toolkit" ;
import modeReducer from "../slices/modeSlice"
const rootReducer = combineReducers({
mode: modeReducer
})
export default rootReducer
|
Javascript
import React from "react" ;
import { useDispatch , useSelector } from "react-redux" ;
import { MdOutlineDarkMode } from "react-icons/md" ;
import { MdOutlineLightMode } from "react-icons/md" ;
import { setMode } from "../slices/modeSlice" ;
const Home = () => {
const dispatch = useDispatch();
const { darkMode } = useSelector(
(state) => state.mode
);
return (
<div>
<section className={`h-screen
flex
items-center
justify-center
text-2xl
cursor-pointer
${darkMode?
"text-richblack-100 "
: "text-richblack-700" }`}
onClick={() => {dispatch(setMode(!darkMode)
)}}>
{darkMode ? (
<MdOutlineLightMode size={ "100px" }/>
) : (
<MdOutlineDarkMode size={ "100px" }/>)}
</section>
</div>
);
};
export default Home;
|
Javascript
import Home from "./pages/Home" ;
import { useSelector } from "react-redux" ;
function App() {
const { darkMode } = useSelector((state) => state.mode);
return (
<div className={`transition-all
duration-500
ease- in -out
${darkMode ?
"bg-black " :
"bg-white" }`}>
<Home />
</div>
)}
export default App;
|
Steps to Run the Application:
Step 1: Type following command in your terminal.
npm start
Step 2: Locate the given URL in your browser.
http://localhost:3000/
Output
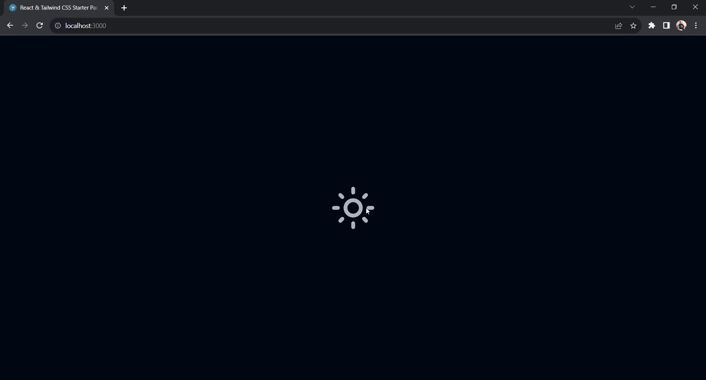
output
Share your thoughts in the comments
Please Login to comment...