What is Redux Toolkit?
Last Updated :
06 Mar, 2024
Redux Toolkit is a powerful and efficient library that simplifies managing the state in React applications using Redux. It provides a set of tools and best practices to streamline the development of complex state logic while maintaining scalability and readability.
In this article, we will cover the description of the Redux Toolkit, various approaches, and steps to create an application and provide an example to better understand redux toolkit.
Redux Toolkit streamlines Redux development by implementing best practices, offering defaults, and simplifying code. It’s beneficial for all Redux users, whether beginners or experienced users, and can be used in new projects or gradually integrated into existing ones. While not mandatory, Redux Toolkit is highly recommended for improving code quality and maintainability.
This approach utilizes the configureStore function provided by Redux Toolkit to create a Redux store with a built-in middleware setup and additional enhancements.
Javascript
import {
configureStore
} from '@reduxjs/toolkit' ;
import todoReducer from './reducers/todoSlice' ;
const store = configureStore({
reducer: {
todos: todoReducer,
},
});
export default store;
|
createSlice():
The createSlice function allows developers to define a slice of the Redux state along with its associated actions and reducers, reducing the need for multiple files.
Javascript
import { createSlice } from '@reduxjs/toolkit' ;
const todoSlice = createSlice({
name: 'todos' ,
initialState: [],
reducers: {
addTodo: (state, action) => {
state.push({
id: Date.now(),
text: action.payload, completed: false
});
},
toggleTodo: (state, action) => {
const todo = state.find((t) =>
t.id === action.payload);
if (todo) {
todo.completed = !todo.completed;
}
},
},
});
export const { addTodo, toggleTodo } = todoSlice.actions;
export default todoSlice.reducer;
|
createAsyncThunk():
For handling asynchronous actions, Redux Toolkit introduces createAsyncThunk which simplifies the process of dispatching async actions and handling loading, success, and error states.
Javascript
import { createAsyncThunk } from '@reduxjs/toolkit' ;
const myAsyncThunk = createAsyncThunk(
'sliceName/thunkName' ,
async (arg1, arg2, ...argN) => {
}
);
|
Steps to Create Application:
Step 1: Create a new app using the following command in your terminal.
npx create-react-app redux-todo-app
Step 2: Navigate to the root directory of your application.
cd redux-todo-app
Step 3: Install Redux Toolkit and React-Redux:
npm install @reduxjs/toolkit react-redux
Project Structure:
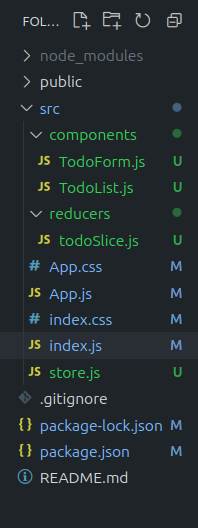
Project structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@reduxjs/toolkit": "^2.2.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example:Let’s create a simple To-Do List application using React and Redux Toolkit.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { Provider } from 'react-redux' ;
import store from './store' ;
import App from './App' ;
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById( 'root' )
);
|
Javascript
import React from 'react' ;
import TodoForm from './components/TodoForm' ;
import TodoList from './components/TodoList' ;
function App() {
return (
<div>
<h1>Redux Toolkit To-Do List</h1>
<TodoForm />
<TodoList />
</div>
);
}
export default App;
|
Javascript
import React from 'react' ;
import {
useSelector,
useDispatch
} from 'react-redux' ;
import {
toggleTodo
} from '../reducers/todoSlice' ;
const TodoList = () => {
const todos = useSelector((state) => state.todos);
const dispatch = useDispatch();
return (
<ul>
{todos.map((todo) => (
<li
key={todo.id}
style={{
textDecoration: todo.completed ?
'line-through' : 'none'
}}
onClick={() => dispatch(toggleTodo(todo.id))}
>
{todo.text}
</li>
))}
</ul>
);
};
export default TodoList;
|
Javascript
import React, {
useState
} from 'react' ;
import {
useDispatch
} from 'react-redux' ;
import {
addTodo
} from '../reducers/todoSlice' ;
const TodoForm = () => {
const [todoText, setTodoText] = useState( '' );
const dispatch = useDispatch();
const handleSubmit = (e) => {
e.preventDefault();
if (todoText.trim() !== '' ) {
dispatch(addTodo(todoText));
setTodoText( '' );
}
};
return (
<form onSubmit={handleSubmit}>
<input
type= "text"
value={todoText}
onChange={(e) =>
setTodoText(e.target.value)}
placeholder= "Add a new todo"
/>
<button type= "submit" >Add Todo</button>
</form>
);
};
export default TodoForm;
|
Start your app using the following command.
npm start
Output :
.gif)
Conclusion:
Redux Toolkit is a valuable tool for React users looking to efficiently manage state in their applications. By providing a set of utilities and best practices, Redux Toolkit significantly reduces the boilerplate code associated with Redux, resulting in cleaner and more maintainable code.
Share your thoughts in the comments
Please Login to comment...