Imagine a React.js world where you can specify exactly the data you need and it flows effortlessly tailored to your needs, and react can efficiently update the DOM as and when needed. No more under-fetching or over-fetching of data or wrestling with nested JSON structures.
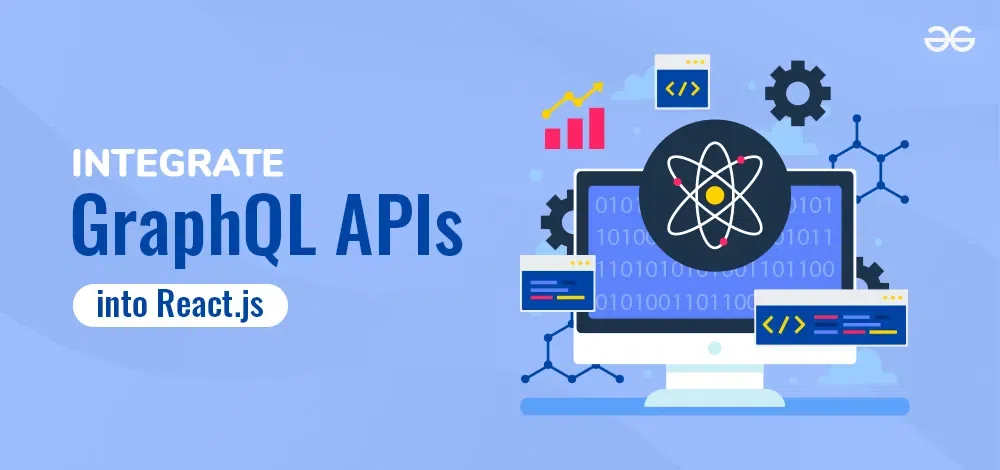
This dream becomes a reality with the use of GraphQL the rising star of API technology. This article aims to help you integrate the GraphQL API with your React project.
What is GraphQL
GraphQL is a query language and runtime for APIs (Application Programming Interfaces) that was developed by Facebook and later open-sourced in 2015. GraphQL allows clients to specify the shape and structure of the data they require, enabling efficient data fetching and reducing the need for multiple round trips to the server. This approach makes it particularly well-suited for applications with complex data requirements, such as those found in modern web and mobile apps. Additionally, GraphQL’s introspective nature allows clients to explore and understand the capabilities of the API, making it easier to work with and adapt to changing requirements.
Enhance your full stack development skills with “Full Stack Development with React & Node JS – Live” course. Join industry experts for live instruction, practical projects, and a dynamic learning experience. Enroll now to take your React and Node.js expertise to the next level!
Why GraphQL
In the traditional method of using the RESTful approach, we request specific data points which often leads to over-fetching or can sometimes lead to under-fetching. The GraphQL declarative method (we mention the fields we need from the server) of data fetching significantly improves performance over a REST API. For example, consider a webpage that displays the shelter names of pets in the shelter and we also want to get the name of the pet and its image.
USING REST, the page might need to:
- Get a list of shelters and their corresponding pet_ids from the /API/shelters endpoint
- GET each pet’s details (name, photo URL, etc.) with a separate request to /API/pets/PET_ID
We can obtain the above in a single query to a single endpoint using GraphQL, the code for which might look something like this:
query GetPetsByShelter{
shelter{
name
pets{
name
photoURL
}
}
}
Want to know more why to use GraphQL check out :Why Use GraphQL?
Requirements
Get the following installed on your local machine before we begin to code:
- Node.js (preferably version 12 or higher)
- npm (preferably version 6 and can be higher)
- Any code editor of your choice such as Visual Studio Code.
- React.js: Basic familiarity with React.js and its concepts.
- GraphQL API: Have access to a GraphQL API.Â
How to Integrate GraphQL APIs into Your React.js Projects
There are generally three ways to integrate GraphQL APIs into a React.js Project. These are:
Note:
- Libraries like Apollo Client simplify many aspects of working with GraphQL in React applications, however, you can use fetch API or external libraries such as Axios to perform the same.
- Step 3 is an additional step for the configuration of the Apollo Client which is not needed in Fetch API or Axios.
Step 1: Set Up a React Application
Open your command prompt or terminal and type the below command. The below command will create a basic react app for us with the basic configurations to help us get started
npx create-react-app graphql-react-app
After everything’s done, go to the folder you just made.
cd graphql-react-app
Now, get things going by typing this:
npm start
Your react app’s up and running at http://localhost:3000
Step 2: Install Required Packages
To get GraphQL talking to your React app, we’ll use a handy helper called Apollo Client. It’s like a translator for GraphQL and React, making life easier.
npm install @apollo/client graphql
Step 3: Using Apollo, Configure the Apollo Client
Note: This step is only needed for the Apollo Client method, not for Fetch API or Axios.
Apollo Client is a powerful GraphQL client that works seamlessly with React, You can think of the Apollo Client as your GraphQL interpreter for React. We can configure our Apollo Client by specifying the endpointÂ
// src/index.js
import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client';
const client = new ApolloClient({
uri: 'https://your-graphql-api-endpoint.com/graphql', // Replace with your GraphQL API endpoint
cache: new InMemoryCache()
});
ReactDOM.render(
<ApolloProvider client={client}>
<App />
</ApolloProvider>,
document.getElementById('root')
);
The above code initializes ApolloClient for GraphQL with the GraphQL APIÂ endpoint and caches it. The ApolloProvider component comes under the @apollo/client package. It uses the ApolloProvider component to wrap the App component so that GraphQL functionality is available within the whole App component (meaning the Apollo Client instance will be provided to all the components in our App). Finally renders it in the root element within the HTML document.
With our Apollo Client now ready we can now use it to fetch and update data in our React App.
Step 4: Create GraphQL Queries
To write our GraphQL questions or queries use the gql tag from the graphql package. It is like writing a clear request for the data you need. Here’s an example, Let’s create a simple query to fetch a list of users
// src/queries.js
import { gql } from '@apollo/client';
export const GET_USERS = gql`
query {
users {
id
name
email
// Add other fields you need
}
}
`;
The explanation of the above code:
The gql function is imported from the @apollo/client package. The gql function is used to define gql queries, mutations, and fragments to integrate in a javascript code. While using the Apollo Client this function is used to parse the GraphQL query strings and make a query document that can be understood by the Apollo Client so this sort of brings in a tool that helps the Apollo Client understand your GraphQL questions.
Defining a GraphQL query: You write your query using the gql tag like filling out a form. GET_USERS is created as a constant with the gql tag . This query requests the data from the GraphQL API with the required fields, here it’s querying for users. With fields such as ID, name, and email, you can specify any other fields you require.
Query Structure:
- query: This word tells Apollo you are asking for information
- users: The specific data you are looking for
- fields: These are the details about the user you want such as id, name, email, etc.
Additional info: You should keep your queries separate in file queries.js to make your files cleaner and easier to use.
Step 5: Fetch Data in React Components
Apollo Client Method
Using Apollo Client to fetch data in your React application
// src/components/UserList.js
import { useQuery } from '@apollo/client';
import { GET_USERS } from '../queries';
function UserList() {
const { loading, error, data } = useQuery(GET_USERS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h2>User List</h2>
<ul>
{data.users.map(user => (
<li key={user.id}>
{user.name} - {user.email}
</li>
))}
</ul>
</div>
);
}
export default UserList;
We can use useQuery to fetch data within the react component named UserList from the GraphQL API in the above way as written in the code. The code is explained:
Importing the required dependencies:
- useQuery is imported from @apollo/client package . This hook lets you ask GraphQL questions within your react component.
- GET_USERS is the query you wrote earlier and it is imported from the ../queries file. This is the query you wrote asking for a list of users
UserList component: This part of the code creates a list to show the users. It is a functional component that utilizes the useQuery hook to execute the GET_USERS query.
The hook returns an object with properties such as loading, error, and data.
- Loading: If the answer is still on its way, the component shows a “Loading…” message.
- Errors: If something unexpected happens the error message can help us in debugging.
- When the data is successfully fetched it is rendered within the react component. The component renders a list of users with name and email. The key attribute is set to user.id for React’s list rendering optimization
Key points:
- The useQuery hook is a special tool for asking GraphQL questions within the react component
- It handles different states such as loading, error, and success to make sure the user experience is smooth.
Fetch API
Use the fetch API in a React Component:
import React, { useState, useEffect } from 'react';
import { GET_USERS } from '../queries';
function UserList() {
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch('https://your-graphql-api-endpoint.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
query: GET_USERS,
}),
});
const result = await response.json();
if (result.errors) {
throw new Error(result.errors[0].message);
}
setUsers(result.data.users);
setLoading(false);
} catch (error) {
setError(error.message);
setLoading(false);
}
};
fetchData();
}, []);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error}</p>;
return (
<div>
<h2>User List</h2>
<ul>
{users.map(user => (
<li key={user.id}>
{user.name} - {user.email}
</li>
))}
</ul>
</div>
);
}
export default UserList;
In the above code :
- GET_USERS is a query string containing the GraphQL query.
- Fetch API makes a POST request to the GraphQL API endpoint with the GraphQL in the request body.
- The component has states (loading, error, and users) to manage the asynchronous data.
- The useEffect hook ensures that the data is fetched only after the component mounts.
Axios Library
Using the axios library if we want to fetch data follow the below code:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
import { GET_USERS } from '../queries';
function UserList() {
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.post('https://your-graphql-api-endpoint.com/graphql', {
query: GET_USERS,
});
if (response.data.errors) {
throw new Error(response.data.errors[0].message);
}
setUsers(response.data.data.users);
setLoading(false);
} catch (error) {
setError(error.message);
setLoading(false);
}
};
fetchData();
}, []);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error}</p>;
return (
<div>
<h2>User List</h2>
<ul>
{users.map(user => (
<li key={user.id}>
{user.name} - {user.email}
</li>
))}
</ul>
</div>
);
}
export default UserList;
- Axios library is used to make a POST request to the GraphQL API endpoint with GraphQL query in the request body
- The component has states (loading, error, and users) to manage the asynchronous data.
- The useEffect hook ensures that the data is fetched only after the component mounts.
Step 6: Display Data in Your React App
You have to import the UserList or other components that use GraphQL API into your react application to display the fetched data.
// src/App.js
import React from 'react';
import UserList from './components/UserList';
function App() {
return (
<div>
<h1>GraphQL React App</h1>
<UserList />
</div>
);
}
export default App;
The explanation of the above code: The app.js is the entry point that controls the overall layout and structure of your app you can think of App.js as the headquarters of your React app. It’s where you decide how everything fits together.
- Importing Components: The UserList component is imported into your app.js file which has the job of fetching and showing the list of users using the GraphQL queries
- App Component: The App component acts like the main building of your app. It creates the overall structure and layout.
Inside App you use JSX (a special way to write HTML-like code in React) to design how the app will look likeÂ
- There’s a main container for everything (div).
- A big heading (h1) announces the app’s name (“GraphQL React App”).
- The UserList component displays its list of users.
Making it all work together: By placing the <UserList /> within the App component’s JSX, the UserList component will be rendered whenever the App component is rendered. This ensures that the data fetched using GraphQL queries in the UserList component will be displayed as a part of the application’s interface.
Step 7: Run Your Application
Run npm start in your terminal or command prompt to start your react application and check if it properly fetches and displays the data from the GraphQL API.
npm startÂ
Conclusion
Congratulations you have successfully integrated GraphQL API with your React js application using Apollo Client. You can now leverage the power of GraphQL in dealing with APIs and efficiently manage your react components for different APIs. The integration of GraphQL helps in creating more efficient web applications. By adapting GraphQL into your React.js projects you are using the latest popular technology in API querying.
FAQs
Why use GraphQL over traditional REST APIs?
Traditional RESTful approach often leads to overfetching or underfetching of data. GraphQL’s declarative method allows developers to request specific data points, significantly improving performance by fetching only the required information.
What are the requirements before integrating GraphQL into a React.js project?
Ensure you have Node.js (preferably version 12 or higher), npm (preferably version 6 or higher), and a code editor such as Visual Studio Code installed. Basic familiarity with React.js concepts and access to a GraphQL API are also prerequisites.
What are the three ways to integrate GraphQL APIs into a React.js project?
The three ways are using Apollo Client, React Query + Axios, and React Query + Fetch API. Apollo Client simplifies many aspects of working with GraphQL in React applications, but you can also use Fetch API or external libraries like Axios.
What is the significance of integrating GraphQL with React.js projects?
Integrating GraphQL with React.js projects provides a more efficient way of dealing with APIs. It allows developers to leverage the power of GraphQL, resulting in more effective management of React components for different APIs and creating more efficient web applications.
Share your thoughts in the comments
Please Login to comment...