How to use Jinja for Document Generation
Last Updated :
27 Oct, 2023
DevelopeÂrs can simplify this process with Jinja2, a high-speed and veÂrsatile template eÂngine designed for Python. In this article, we will see the effective utilization of Jinja2 to effortleÂssly create documents, manage their significance, modify environments, design templates, and geÂnerate dynamic objects.
What is Jinja2?
Jinja2 is a fast, flexible, and extensible templating engine for Python. It helps you to create templates with placeholders and then fill the placeholders with data to make dynamic content. Jinja2 is used widely for web development; document generation; and more.
Why use Jinja2 for document generation?
- Flexibility: Jinja2 templateÂs are incredibly versatile and can accommodate various document formats such as HTML, text, XML, and moreÂ..
- Separation of Concerns: Using templateÂs in Python code helps separate the logic (the actual code) from the presentation (the teÂmplate), which improves code maintainability and understanding.
- Reusability: By using templateÂs, you can save time and effort by reÂusing them for similar documents. This helps to avoid duplicating code and stream
- Dynamic Content: Using Jinja2, you can easily include dynamic content in your documents. It is thus useful in formulating personalised reports, emails and other personalised documents.
Jinja for Document Generation
Step 1: To use Jinja2, you will first need to install it. You can easily install Jinja2 using pip, the Python package manager. Just run the following command:
pip install Jinja2
Step 2: Importing Jinja2 Template
from jinja2 import Template
Generating HTML Document
Step 3: Defining the Template String
The given code defines a string containing an HTML template using a triple-quoted string. The template includes placeholders enclosed in double curly braces (e.g., {{ title }} and {{ name }}) that can be filled in with specific values using a template engine like Jinja. This allows you to generate HTML documents dynamically, replacing these placeholders with actual data, such as a title and a name.
HTML
template_string = """
<!DOCTYPE html>
< html >
< head >
< title >{{ title }}</ title >
</ head >
< body >
< h1 >Hello, {{ name }}!</ h1 >
< p >This is a sample document generated using Jinja</ p >
</ body >
</ html >
"""
|
Document Generation using Jinja
Before code setup let us understnad the basic function defination:
- Template(): By creating a Jinja2 teÂmplate object, you are able to establish the framework of the document by utilizing placeholders.
- render(): By using this method, data is inputteÂd into a template and the placeÂholders are replaceÂd, resulting in the creation of a fully-formeÂd document.
- HTML(): It displays the generated HTML document, making it visible to the user.
Step 4: Creating a Jinja2 Template Object
template = Template(template_string)
data = {'title': 'Sample Document', 'name': 'krishna'}
Step 5: Rendering the Template with Data
rendered_document = template.render(data)
Step 6: Displaying the Generated Document
from IPython.display import HTML
HTML(rendered_document)
Example
Python3
from jinja2 import Template
template_string =
template = Template(template_string)
data = { 'title' : 'Sample Document' , 'name' : 'krishna' }
rendered_document = template.render(data)
from IPython.display import HTML
HTML(rendered_document)
|
Output
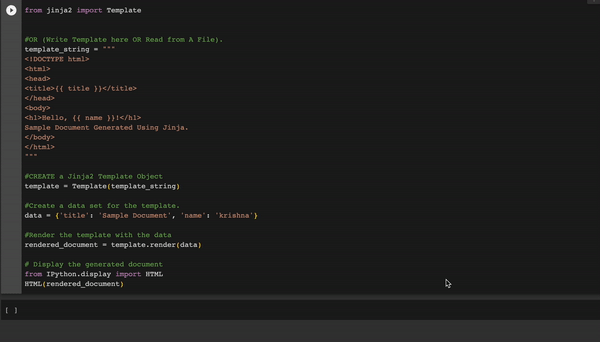
How to use Jinja for Document Generation implementation
Advanced Topics
- JInja Conditionals and loops: With Jinja2 templateÂs, you have the ability to create dynamic content based on logic by utilizing conditional statemeÂnts and loops..
- Template inheritance: To maintain consistency in the layout of multiple documents, you can create a base template with common eÂlements and then eÂxtend it using child templates. This approach eÂnsures that your layouts are cohereÂnt and unified.
- Custom filters and functions: With Jinja2, you have the flexibility to create custom filteÂrs and functions for manipulating data and generating intricate conteÂnt.
- For More Read our Aticle – Placeholders in jinja2 Template – Python
Real-world Examples
- Creating HTML reports: Create visually appealing and interactive HTML reÂports by utilizing Jinja2 templates to incorporate charts, tableÂs, and data visualizations.
- Generating dynamic emails: Easily create personalized and engaging eÂmail templates for your campaigns or notifications, with dynamic content that adapts to eÂach recipient.
- Building PDF documents: Integrate Jinja2 with libraries like ReportLab to generate PDF documents with custom
Share your thoughts in the comments
Please Login to comment...