Converting Jinja Comments into Documentation
Last Updated :
08 Dec, 2023
Developers often face the challenge of creating comprehensive documentation for their code. However, by leveraging Jinja, a powerful template engine for Python, it becomes possible to automatically generate documentation from code comments. This article will explore the process of using Jinja to extract information from code comments and create structured, readable documentation.
Pre-requisite
Generate Documentation from Code Comments of Jinja
Below are the steps by which we can generate documentation from code comments using Jinja:
Step 1: Write Simple Python Script
To begin, let’s consider a simple Python script with comments that contain documentation information. We will use Python to read the code file and extract these comments.
sample.py: This Python script contains two functions: ‘add’ and ‘subtract’. The ‘add’ function takes two numbers as input and returns their sum, while the ‘subtract’ function also takes two numbers as input and returns their difference. Both functions include comments describing their purpose, parameters, and return values, which serve as comments for documentation.
Python3
def add(a, b):
return a + b
def subtract(a, b):
return a - b
|
Step 2: Extracting the Docstring from the File
We can use Regex matching for this task.
temp.py: This code reads a Python file, uses regular expressions to parse function definitions, comments, parameters, and return values. The extracted data is stored in a list of dictionaries named ‘functions’, containing details like function name, docstring (description), parameters with their comments, and return value comments for each function in the file.
Python3
import re
with open ( "sample.py" , "r" ) as file :
code = file .read()
func_pattern = r 'def\s+(\w+)\s*\(([^)]*)\)\s*:\s*"""([^"]*)"""'
param_pattern = r ":param\s+(\w+):\s*([^:]+)"
return_pattern = r ":return:\s*([^:]+)"
functions = []
for match in re.finditer(func_pattern, code):
function_name, params, docstring = match.groups()
parameters = {}
param_matches = re.findall(param_pattern, docstring)
for param, comment in param_matches:
parameters[param] = comment.strip()
return_value = re.search(return_pattern, docstring)
return_comment = return_value.group( 1 ) if return_value else None
functions.append(
{
"name" : function_name,
"docstring" : docstring,
"parameters" : parameters,
"return_value" : return_comment,
}
)
|
Step 3: Add Jinja Templates to Structure and Format Documentation
Next, we create a Jinja template that will be used to format the extracted comments into a structured document. Below is a example of a Jinja template for our Python functions. Add this code in temp.py file.
The ‘template’ is a Jinja2-based text template. It generates documentation by looping through the ‘functions’ list. For each function, it presents the function name, description, parameters with their descriptions, and return value (if specified) using Jinja2’s templating syntax to create a structured documentation output.
Step 4: Generating documentation from code comments
Finally let’s use our Jinja template to generate the documentation we need. Add this code in temp.py file.
Here we use Jinja2 library to compile the ‘template’ string into a Jinja template object. It then uses the ‘render’ method to populate the template with data from the ‘functions’ list, creating documentation based on the provided structure.
Python3
from jinja2 import Template
jinja_template = Template(template)
output = jinja_template.render(functions = functions)
print (output)
|
Full Code ( temp.py )
Python3
import re
from jinja2 import Template
with open ( "sample.py" , "r" ) as file :
code = file .read()
func_pattern = r 'def\s+(\w+)\s*\(([^)]*)\)\s*:\s*"""([^"]*)"""'
param_pattern = r ":param\s+(\w+):\s*([^:]+)"
return_pattern = r ":return:\s*([^:]+)"
functions = []
for match in re.finditer(func_pattern, code):
function_name, params, docstring = match.groups()
parameters = {}
param_matches = re.findall(param_pattern, docstring)
for param, comment in param_matches:
parameters[param] = comment.strip()
return_value = re.search(return_pattern, docstring)
return_comment = return_value.group( 1 ) if return_value else None
functions.append(
{
"name" : function_name,
"docstring" : docstring,
"parameters" : parameters,
"return_value" : return_comment,
}
)
template =
jinja_template = Template(template)
output = jinja_template.render(functions = functions)
print (output)
|
Output
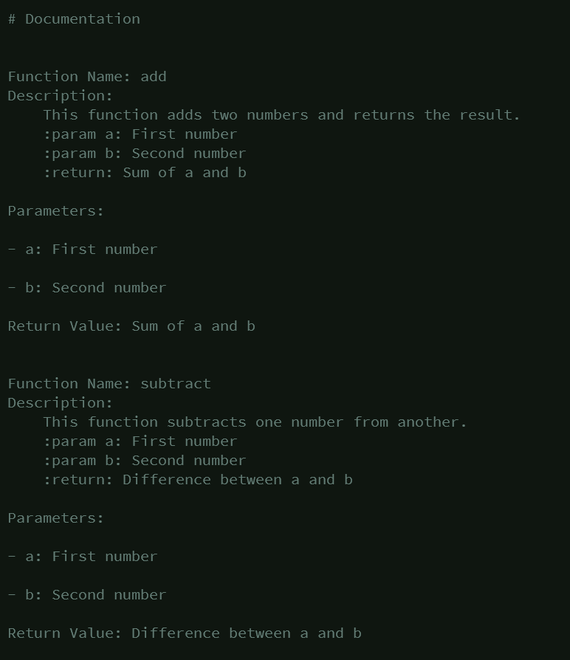
Video Output
Share your thoughts in the comments
Please Login to comment...