Generate sitemaps for SEO using Jinja templates
Last Updated :
29 Dec, 2023
In this article, we will guide you step-by-step on How to generate sitemaps for SEO using Jinja templates in Python. Emphasizing the critical importance of a well-organized sitemap for SEO, we will provide a detailed example, demonstrating how Jinja templates can be employed to create dynamic sitemaps. This tutorial aims to empower you with the knowledge to enhance your website’s visibility by ensuring efficient scanning and indexing by search engines.
What are Sitemaps?
Sitemaps play a crucial role in optimizing a website for search engines. They provide search engines with a structured overview of a website’s content, making the process of crawling and indexing more efficient. In essence, a sitemap is an XML file containing a comprehensive list of a website’s URLs, accompanied by additional metadata such as the date of the last modification.
The benefits of sitemaps extend beyond facilitating efficient crawling; they also aid search engines in understanding the organizational structure of a site. By providing a clear map of the website’s architecture, sitemaps enhance the search engine’s ability to prioritize and comprehend the relevance of different pages.
How Search Engines Use Sitemaps:
Crawling Efficiency: Sitemaps assist search engine bots in discovering and crawling all relevant pages on a website promptly. This is particularly beneficial for large websites or those with complex structures.
Indexing Accuracy: The metadata included in a sitemap, such as the last modification date, helps search engines prioritize and index the most up-to-date content. This ensures that users receive the latest and most relevant search results.
Structural Understanding: Sitemaps provide a blueprint of the website’s organizational hierarchy. This helps search engines comprehend the relationships between different pages and prioritize content based on its importance and relevance.
What are Jinja Templates?
Jinja templates are a templating engine used in Python for dynamically generating text-based output, such as HTML, XML, or other markup languages. Developed primarily for web frameworks like Flask, Jinja allows developers to embed dynamic content within static templates. The templating process involves using placeholders enclosed in double curly braces ({{ }}) within the template, which is later replaced with actual values during rendering.
Generate Sitemaps for SEO Using Jinja Templates
This Python code shows how to make an XML sitemap using Jinja templates. First, it brings in the necessary tools, especially the Template class from Jinja2. Then, it uses a pretend set of data with URLs and the dates they were last modified. The Jinja template sets up the structure of the XML sitemap and uses special markers to fill in the actual details when it’s put together. The code creates a Jinja template and fills it in with the example data, resulting in the XML content for the sitemap. The generated sitemap is printed out, and it’s also saved to a file named “sitemap.xml” using the UTF-8 format. The script wraps up by confirming that the sitemap was made successfully. If you want to use your own URLs and modification dates, just swap out the example data, and if you prefer a different file location, you can change the file path.
Python3
from jinja2 import Template
urls = [
]
template_str =
template = Template(template_str)
sitemap_content = template.render(entries = urls)
print (sitemap_content)
with open ( "sitemap.xml" , "w" , encoding = "utf-8" ) as f:
f.write(sitemap_content)
print ( "Sitemap generated successfully!" )
|
Output
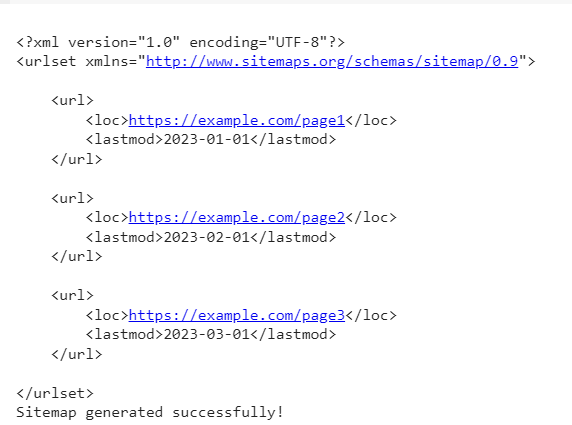
Conclusion
In Conclusion, mastering the use of Jinja templates in Python empowers developers to dynamically generate SEO-friendly sitemaps. By efficiently organizing URLs and metadata in XML format, this approach enhances a website’s visibility and aids search engines in effectively indexing its content. Understanding and implementing this process is crucial for optimizing web content and facilitating its discoverability in search engine results.
Share your thoughts in the comments
Please Login to comment...