How To Update Multiple Columns in MySQL?
Last Updated :
08 Apr, 2024
To update multiple columns in MySQL we can use the SET clause in the UPDATE statement. SET clause allows users to update values of multiple columns at a time.
In this article, we will learn how to update multiple columns in MySQL using UPDATE and SET commands. We will cover the syntax and examples, providing explanations to help you understand how to update multiple columns in SQL with a single query.
MySQL Update Multiple Columns
The UPDATE statement allows users to modify the values of specific columns in existing records.
Users need to identify the new values for each column in the SET clause and establish the criteria for which records to update using the WHERE clause.
Syntax
– – MySQL syntax to update multiple columns with one query
UPDATE your_table
SET
column1 = value1,
column2 = value2,
– – Additional columns and values as needed
WHERE
condition;
Process
- Identify the Table: Begin by specifying the table, referred to as your_table, which contains the records you intend to update.
- Set New Values: Within the SET clause, outline the new values for each column that requires modification. For instance, if you want to update column1 with value1 and column2 with value2, you include these assignments here. Add more columns and values as necessary.
- Specify Conditions: The WHERE clause comes into play to set conditions for the records to be updated. It’s crucial to define these conditions accurately; otherwise, all records in the table will be updated.
Update Multiple Columns in MySQL Examples
Let’s look at some examples on how to update multiple columns in MySQL.
Example 1: Update Multiple Columns in One Table
Assume you have a table named employees with columns employee_id, first_name, last_name, and salary. You want to update the salary and last name for a specific employee.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(50),
salary INT,
department VARCHAR(50)
);
-- Sample Data
INSERT INTO employees VALUES (1, 'John Doe', 50000, 'HR');
INSERT INTO employees VALUES (2, 'Jane Smith', 60000, 'IT');
INSERT INTO employees VALUES (3, 'Bob Johnson', 55000, 'Finance');
-- Before Update
SELECT * FROM employees;
-- Update Multiple Columns
UPDATE employees
SET salary = 65000, department = 'Marketing'
WHERE employee_id = 2;
-- After Update
SELECT * FROM employees;
Output:
Before Update:
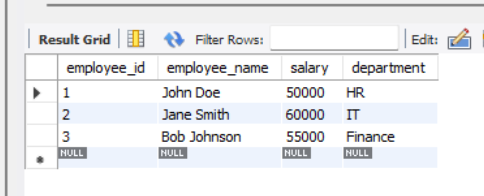
Output
After Update:
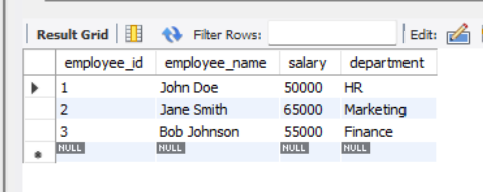
Output
Explanation: The UPDATE statement modifies the salary to 65000 and the department to ‘Marketing‘ for the employee with ID 2. The SELECT statement displays the updated employee records.
Example 2: Update Multiple Columns in Different Tables
-- Schema
CREATE TABLE orders (
order_id INT PRIMARY KEY,
order_date DATE,
customer_name VARCHAR(50)
);
CREATE TABLE order_details (
order_id INT,
product_name VARCHAR(50),
quantity INT,
total_price DECIMAL(8, 2),
FOREIGN KEY (order_id) REFERENCES orders(order_id)
);
-- Sample Data
INSERT INTO orders VALUES (1, '2024-02-01', 'John Doe');
INSERT INTO order_details VALUES (1, 'Laptop', 2, 1200.00);
-- Before Update
SELECT * FROM orders;
SELECT * FROM order_details;
-- Update Multiple Columns
UPDATE orders
JOIN order_details ON orders.order_id = order_details.order_id
SET orders.customer_name = 'Jane Smith', order_details.quantity = 3, order_details.total_price = 1800.00
WHERE orders.order_id = 1;
-- After Update
SELECT * FROM orders;
SELECT * FROM order_details;
Output:
Before Update:

Output
After Update:

Output
Explanation: The UPDATE statement modifies the customer name in the orders table to ‘Jane Smith‘ and updates the quantity and total price in the order_details table for the specified order (order_id = 1).
After the update, the SELECT statements display the updated data in both the orders and order_details tables.
Conclusion
To update multiple columns in MySQL we use the UPDATE statement with SET clause. When used with WHERE clause we can specify the columns that we need to update.
This tutorial explained how to perform multiple updates on multiple columns in a table. We also covered how to update multiple columns of different tables in MySQL. Examples with explanations provide better understanding on the concept.
Share your thoughts in the comments
Please Login to comment...