How to Set a Column Value to NULL in SQL Server
Last Updated :
12 Apr, 2024
In the world of database management, SQL Server is a leading and extensively utilized system. A fundamental task within SQL Server is manipulating data within tables, and setting a column value to NULL is a common operation. Whether it’s for maintaining data integrity, performing updates, or meeting specific business logic requirements, efficiently executing this action is crucial.
In this article, we’ll delve into setting column values to NULL in SQL Server. We’ll cover three methods: utilizing UPDATE statements, employing CASE statements for conditional updates, and concatenating values using CONCAT.
Understanding NULL in SQL Server
SQL Server uses NULL as the representation of the absence of a value in a column. It is not the same as an empty string or a null value. A column with NULLs allows a column to have NULL values. Knowing this paraphrases the idea that setting a column to NULL means getting rid of the current value and leaving it empty.
- Setting a Column Value to NULL Using UPDATE
- Using CASE Statements for Conditional Updates
- Concatenating Column Values Using CONCAT
Set up an Environment
We have built an EmployeeDetails table with four columns (EmployeeID, FirstName, LastName, and Department). EmployeeID is the primary key. It then populates four sample records into the table.
-- Creating the EmployeeDetails table
CREATE TABLE EmployeeDetails (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Department VARCHAR(50)
);
-- Inserting sample data into the EmployeeDetails table
INSERT INTO EmployeeDetails (EmployeeID, FirstName, LastName, Department)
VALUES
(101, 'John', 'Doe', 'Sales'),
(102, 'Jane', 'Smith', 'Marketing'),
(103, 'Michael', 'Johnson', 'HR'),
(104, 'Emily', 'Williams', 'IT');
Output:
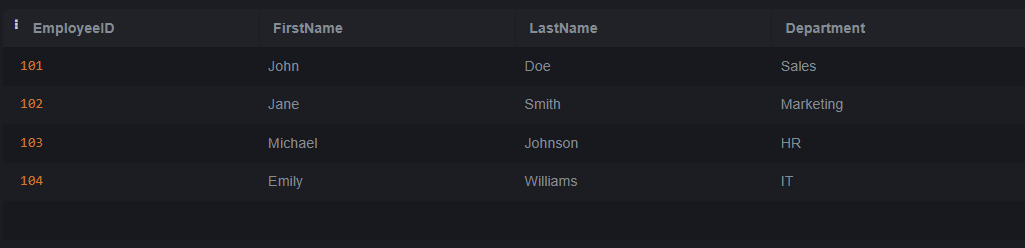
1. Setting a Column Value to NULL Using UPDATE
This method involves the UPDATE statement to null a particular column value for a given row of the tables. It would be used if you were obliged to either clear or nullify certain column values.
-- The Department is to be updated to null instead of the employee's EmployeeID 101
UPDATE EmployeeDetails
SET Department = NULL
WHERE EmployeeID = 101;
-- Displaying updated data
SELECT * FROM EmployeeDetails;
Output:
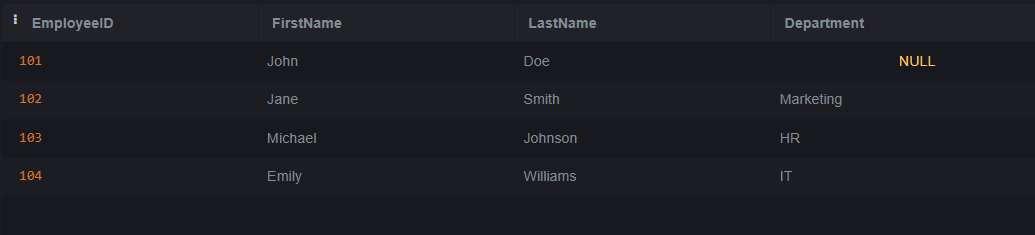
Explanation: After executing this method the Department value for that employee with EmployeeID 101 is null. The rest of the data remains the same. It erases the department data associated with that particular employee.
2. Using CASE Statements for Conditional Updates
Case statements within SQL statements provide the ability to execute conditional logic. In this approach, column values are edited whenever the specified condition is met, thus giving a freedom to edit different data columns based on different criteria.
-- We will update the Department Table with the employeeID.
UPDATE EmployeeDetails
SET Department =
CASE
WHEN EmployeeID = 102 THEN ''Finance''
ELSE Department
END;
-- Displaying updated data
SELECT * FROM EmployeeDetails;
Output:
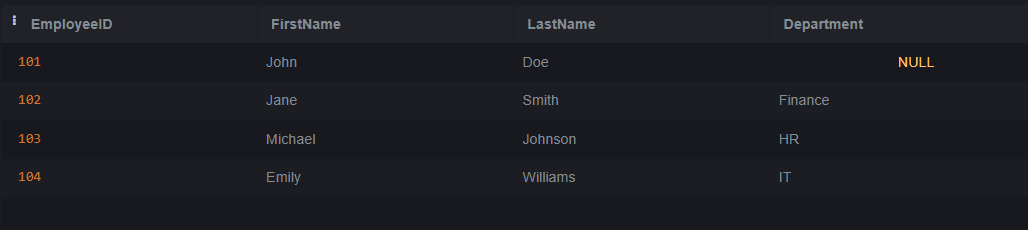
Explanation: With the use of this technique, Department value is updated as “Finance” for the employee with id 102, and the other employee’s department values remain without any changes. That shows how conditional update can be carried out according to the conditions you specify.
3. Concatenating Column Values Using CONCAT
Concatenating column values allows you to combine multiple columns into a single string value. This method is useful for generating composite fields or enhancing data presentation.
-- Creating another column for FullName with the help of FirstName and LastName strings.
ALTER TABLE EmployeeDetails
ADD FullName VARCHAR(100);
-- Finalizing FullName by concatenating two names.
UPDATE EmployeeDetails
SET FullName = CONCAT(FirstName, ' ', LastName);
-- Displaying updated data
SELECT * FROM EmployeeDetails;
Output:
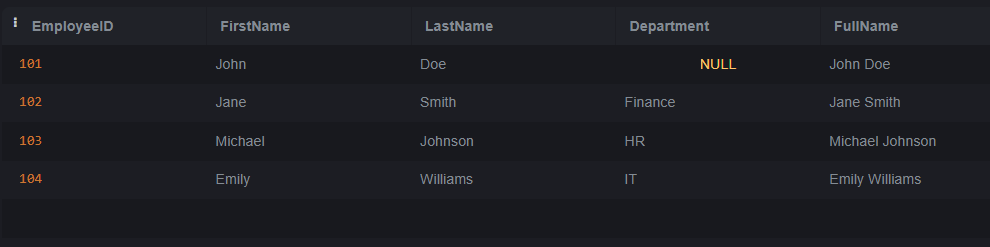
Explanation: The result of the method is the addition of the column named FullName in the table called EmployeeDetails and its value is the concatenating the FirstName and LastName columns. Now, the FullName column for each employee features their full name, thus enhancing the data capabilities by combining these values.
Conclusion
The process of managing column values in SQL Server may pose certain challenges requiring specific solutions. The utilization of UPDATE statements, CASE expressions, and string operations like CONCAT allows developers to effectively modify column values that meet the data quality and performance requirements. Comprehending these techniques and caveats equips database administrators and application developers with the capabilities to deal with the column values within the SQL Server databases efficiently.
Share your thoughts in the comments
Please Login to comment...