How to Toggle Popup using JavaScript ?
Last Updated :
03 Apr, 2024
A popup is a graphical user interface element that appears on top of the current page, often used for notifications, alerts, or additional information.
These are the following approaches to toggle a popup using JavaScript:
Using visibility property
In this approach, we are using the visibility property to control the visibility of the popup element. Initially, we set the CSS visibility property to hidden, When the toggle action is triggered a JavaScript function will be called to check the current visibility property value. If it’s visible, the function will set it to hidden to hide the popup and if it’s hidden function sets it to visible to show the popup.
Syntax:
element.style.visibility = (element.style.visibility === "visible") ? "hidden" : "visible";
Example: This example uses visibility property to toggle a popup using JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Toggle Popup</title>
<style>
body {
text-align: center;
height: 100vh;
background-color: #f4f4f4;
}
.popup {
visibility: hidden;
position: fixed;
top: 30%;
left: 50%;
transform: translate(-50%, -50%);
width: 200px;
height: 150px;
padding: 20px;
border-radius: 8px;
box-shadow:
0 0 20px rgba(0, 0, 0, 0.3);
z-index: 1000;
transition: visibility 0.3s ease;
}
button {
padding: 10px 20px;
background-color: #4CAF50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
.popup.show {
visibility: visible;
}
</style>
</head>
<body>
<button onclick="togglePopup()">Toggle Popup</button>
<div id="popup" class="popup">
<h4>Welcome to GeeksForGeeks</h4>
<p>This is a popup!</p>
</div>
<script>
function togglePopup() {
let popup = document.getElementById("popup");
popup.style.visibility =
(popup.style.visibility === "visible")
? "hidden" : "visible";
}
</script>
</body>
</html>
Output:
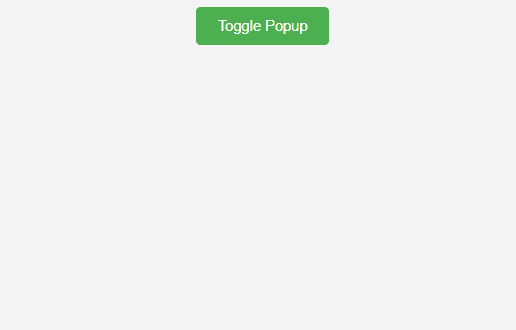
Using ClassList
In this approach we are using classList property to toggle a CSS class that controls the visibility of popup element. We will create a show class with display property set to block to show popup. When a toggle action is triggered a javascript function will be called to toggle the show class on the popup element. If the class is present, it is removed to hide the popup. If class is not present, it is added to show popup.
Syntax:
element.classList.toggle("show");
Example: This example uses ClassList property to toggle the popup using JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Toggle Popup</title>
<style>
body {
text-align: center;
height: 100vh;
background-color: #f4f4f4;
}
.popup {
display: none;
position: fixed;
top: 30%;
left: 50%;
transform: translate(-50%, -50%);
width: 200px;
height: 150px;
padding: 20px;
box-shadow:
0 0 20px rgba(0, 0, 0, 0.3);
}
button {
padding: 10px 20px;
background-color: #4CAF50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
.popup.show {
display: block;
}
</style>
</head>
<body>
<button onclick="togglePopup()">Toggle Popup</button>
<div id="popup" class="popup">
<h3>Welcome to GeeksForGeeks</h3>
<button onclick="togglePopup()">Close Popup</button>
</div>
<script>
function togglePopup() {
let popup = document.getElementById("popup");
popup.classList.toggle("show");
}
</script>
</body>
</html>
Output:
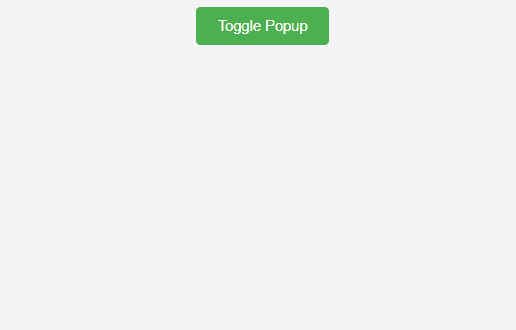
Share your thoughts in the comments
Please Login to comment...