How to create Popup Box using HTML, CSS and JavaScript ?
Last Updated :
09 Oct, 2023
In this article, we will see how to create a pop-up box with the help of HTML, CSS, and JavaScript.
Here we will create a Popup box that is functional through the Popup button, when is clicked the Popbox appears on the screen with the title and close button.
Prerequisites
Approach
- Create the Popup structure using HTML tags, Some tags are used in this project like <h1>,<div>,<p>.
- Add the different styling properties using CSS to style your Popup structure, give padding, margins, and font size accordingly, and add some hover, and transition properties for look and feel.
- In JavaScript, first, get button elements through their id or class and then apply addEventListener on the Popup button as well as the Close button.
- “Click” event is used, popup box appears while clicking on the popup button.
- A popup box appears with “You have Subscribed” and a Close button.
- The close button is used to remove the popup box by changing the display property to none.
Project Structure
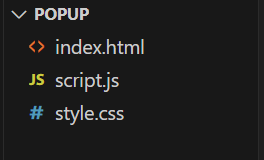
Project Structure
Example: This example implements a popup using above mentioned approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" />
< link rel = "stylesheet"
href =
< link rel = "stylesheet" href = "style.css" />
< title >Document</ title >
</ head >
< body >
< div class = "heading" >
< h1 >GeeksForGeeks</ h1 >
</ div >
< h2 >
How to create Popup Box using
HTML,CSS and JS
</ h2 >
< p id = "sub-p" >
Click below button for Popup
</ p >
< div class = "outer" >
< div class = "popup" >
< i class = "far fa-check-circle" ></ i >
< h2 >You have Subscribed</ h2 >
< br />
< button id = "closebtn" >Close</ button >
</ div >
< button id = "showbtn" >Popup</ button >
</ div >
< br />
< script src = "./script.js" ></ script >
</ body >
</ html >
|
CSS
body {
background-color : #09dbd4 50;
}
.outer {
display : flex;
justify- content : center ;
align-items: center ;
height : 400px ;
}
.heading {
display : flex;
align-items: center ;
height : 28px ;
justify- content : center ;
}
h 1 ,
h 2 {
text-align : center ;
}
h 1 {
color : green ;
background-color : white ;
display : inline ;
}
h 2 {
color : rgb ( 139 , 52 , 52 );
}
p {
text-align : center ;
font-weight : bold ;
}
.popup {
background-color : #fafafa ;
width : 366px ;
height : 222px ;
border-radius: 26px ;
text-align : center ;
display : none ;
transition: all 0.5 s ease;
transition-duration: 1 s;
}
#showbtn {
margin : 200px auto ;
}
#closebtn {
margin-top : 3px ;
}
.popup button {
margin-top : 6px ;
}
button {
background-color : rgb ( 0 , 0 , 0 );
color : white ;
border-radius: 5px ;
height : 36px ;
width : 77px ;
border : none ;
transition-duration: 0.5 s;
font-size : 17px ;
}
.far.fa-check- circle {
color : blue ;
font-size : 37px ;
margin-top : 7px ;
}
button:hover {
background-color : rgb ( 113 , 140 , 139 );
color : white ;
}
|
Javascript
let showbtn = document.getElementById( "showbtn" );
let closebtn = document.getElementById( "closebtn" );
let popup = document.querySelector( ".popup" );
let subp = document.getElementById( "sub-p" );
showbtn.addEventListener( "click" , () => {
popup.style.display = "block" ;
showbtn.style.display = "none" ;
document.body.style.backgroundColor = "#9EA9B1" ;
subp.style.display = "none" ;
});
closebtn.addEventListener( "click" , () => {
popup.style.display = "none" ;
showbtn.style.display = "block" ;
document.body.style.backgroundColor = "#09dbd450" ;
subp.style.display = "block" ;
});
|
Output:
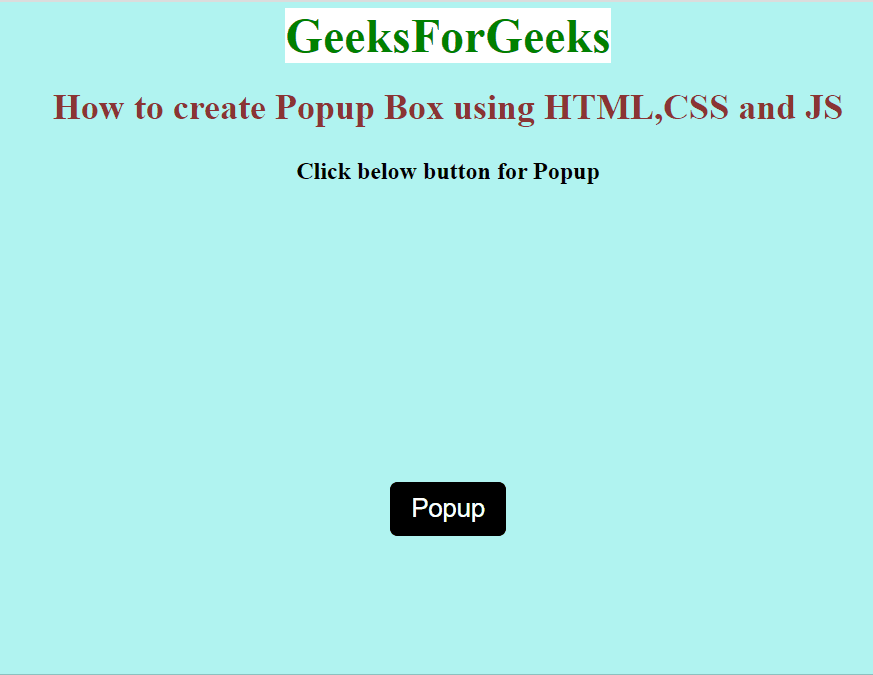
Output
Share your thoughts in the comments
Please Login to comment...