How to Toggle between Hiding and Showing an Element using JavaScript ?
Last Updated :
09 Feb, 2024
Toggle between hiding and showing an element using JavaScript provides the feature of efficient space usage by allowing users to hide content sections they may not need to minimize distractions, leading to a cleaner and more organized layout.
Syntax
if (paragraph.style.display === 'none')
{
paragraph.style.display = "block";
}
else
{
paragraph.style.display = "none"
}
Using CSS Display Property
Make a card with the main heading, subheading, paragraph, and button. Give a specific style to all the elements. The JavaScript function togglelement()
is called when the user clicks the button. It gets the div element with the id myparagraph
. It checks the current display property using the if-else of the div. If the value is none, it changes it to block to make it visible. Refer to the CSS Display Property article for the detailed description.
Example: Illustration of toggling between hiding and showing an element using display property.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Toggle between hiding and showing an element</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width,initial-scale=1.0" >
< style >
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
.card {
width: 300px;
height: 250px;
padding: 20px;
text-align: center;
border: 3px solid #755757;
border-radius: 20px;
}
h2,
h3 {
color: #257443;
}
div {
font-size: 20px;
padding: 3px;
font-weight: 700;
color: rgb(175, 88, 95);
background-color: rgb(230, 238, 230);
}
button {
margin-top: 10px;
padding: 8px;
cursor: pointer;
border-radius: 10px;
background-color: #395e47;
color: white;
font-size: 15px;
}
</ style >
</ head >
< body >
< div class = "card" id = "myCard" >
< h2 >GeeksforGeeks</ h2 >
< h3 >Toggle between hiding and
showing an element
</ h3 >
< div id = "myparagraph" >
Welcome to GeeksforGeeks
</ div >
< button onclick = "togglelement()" >
Click
</ button >
</ div >
< script >
function togglelement() {
const paragraph = document.
getElementById('myparagraph');
if (paragraph.style.display === 'none') {
paragraph.style.display = "block";
}
else {
paragraph.style.display = "none"
}
}
</ script >
</ body >
</ html >
|
Output:
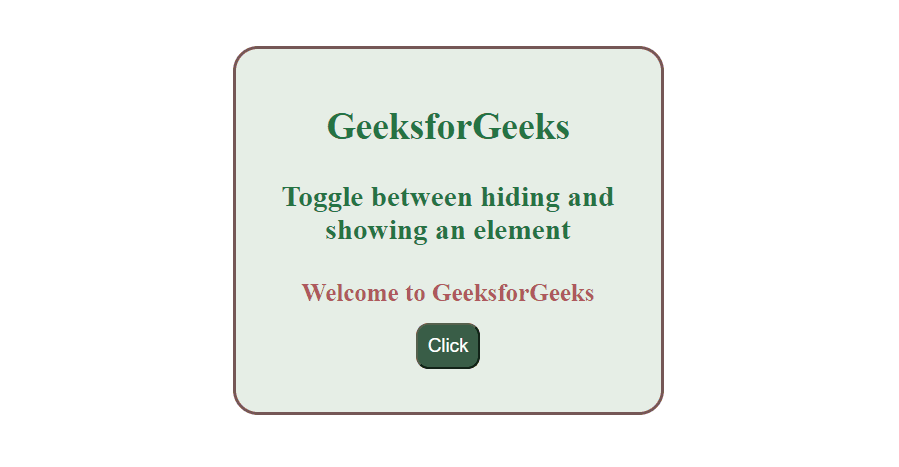
Using CSS Visibility Property
Make a card with the main heading, subheading, paragraph, and button. Give a specific style to all the elements. The JavaScript function togglelement()
is called when the user clicks the button. It gets the div element with the id myparagraph
. The toggleElement function switches the visibility of an element with the id ‘myparagraph’. It checks the current visibility style using “window.getComputedStyle” and toggles it, if hidden, it becomes visible, and vice versa. Refer to the visibility Article for the detailed description.
Example: Illustration of toggling between hiding and showing an element using visibility property.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< style >
@import url(
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
font-family: 'Poppins', sans-serif;
}
.card {
width: 300px;
padding: 20px;
text-align: center;
border: 5px solid #1e4e2e;
border-radius: 20px;
}
h2 {
color: #257443;
}
h3 {
color: blueviolet;
}
div {
font-size: 20px;
padding: 3px;
font-weight: 700;
color: rgb(22, 74, 39);
background-color: rgb(227, 238, 227);
}
button {
margin-top: 10px;
padding: 8px;
cursor: pointer;
border-radius: 10px;
background-color: #50aa72;
color: white;
font-size: 15px;
}
</ style >
</ head >
< body >
< div class = "card" id = "myCard" >
< h2 >GeeksforGeeks</ h2 >
< h3 >Hiding and showing an
element using Visibility
property
</ h3 >
< div id = "myparagraph" >
GeeksforGeeks
</ div >
< button onclick = "toggleElement()" >
Click to Toggle Text
</ button >
</ div >
< script >
function toggleElement() {
const paragraph = document
.getElementById('myparagraph');
const currentVisibility = window
.getComputedStyle(paragraph).visibility;
if (currentVisibility === 'hidden') {
paragraph.style.visibility = 'visible';
} else {
paragraph.style.visibility = 'hidden';
}
}
</ script >
</ body >
</ html >
|
Output:
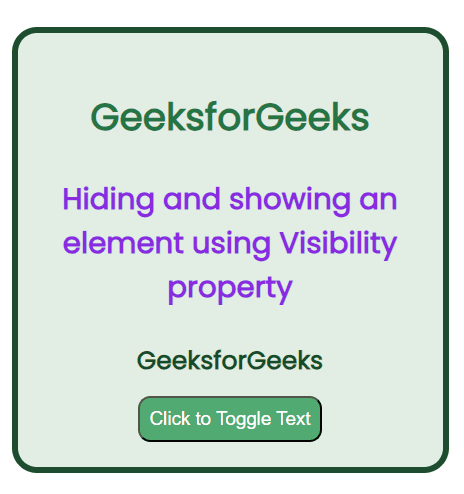
Output
Share your thoughts in the comments
Please Login to comment...