How to Open a Popup on Hover using JavaScript ?
Last Updated :
18 Mar, 2024
In JavaScript, to perform the functionality of opening the popup on hover, we can dynamically manipulate the DOM by responding to the mouse events.
The below approaches can be used to accomplish this task.
Using mouseover and mouseout events
In this approach, we are using the JavaScript events mouseover and mouseout to toggle the display of the popup content. The mouseover event is used to display the popup while mouseout is used to hide the same.
Syntax:
element.addEventListener(event, listener, useCapture);
Example: The below example uses JavaScript Events to open and hide a popup on hover using JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 50px;
}
.popup-container {
color: green;
display: inline-block;
position: relative;
}
.popup-content {
display: none;
position: absolute;
top: 100%;
left: 50%;
transform: translateX(-50%);
padding: 10px;
background-color: #f1f1f1;
border: 1px solid #ccc;
border-radius: 5px;
}
</style>
</head>
<body>
<h3>
Using mouseover and mouseout JavaScript Events
</h3>
<div class="popup-container" id="popupContainer">
Hover me to see popup
<div class="popup-content" id="popupContent">
Hey Geek, <br />
Welcome to GeeksforGeeks!!
</div>
</div>
<script>
const popupContainer =
document.getElementById('popupContainer');
const popupContent =
document.getElementById('popupContent');
popupContainer.addEventListener
('mouseover', function () {
popupContent.style.display = 'block';
});
popupContainer.addEventListener
('mouseout', function () {
popupContent.style.display = 'none';
});
</script>
</body>
</html>
Output:
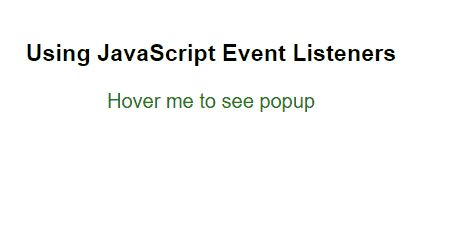
Using onmouseenter and onmouseleave events
In this approach, we are using the onmouseenter and onmouseleave events directly in JavaScript to control the display of a popup on hover. The script sets the display property of the popup content to ‘block‘ on mouse enter and ‘none‘ on mouse leave.
Syntax:
object.onmouseenter = function(){myScript};
object.onmouseleave = function(){myScript};
Example: The below example uses onmouseenter and onmouseleave events to open a popup on hover using JavaScript.
HTML
<!DOCTYPE html>
<head>
<title>Example 2</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 50px;
}
.popup-container {
display: inline-block;
position: relative;
}
.popup-content {
display: none;
position: absolute;
top: 120%;
left: 50%;
transform: translateX(-50%);
padding: 15px;
background-color: #ebdb00;
color: green;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
opacity: 0;
transition: opacity 0.3s ease-in-out,
top 0.3s ease-in-out;
}
.popup-container:hover
.popup-content {
top: 100%;
opacity: 1;
}
</style>
</head>
<body>
<h3>
Using onmouseenter and
onmouseleave Attributes
</h3>
<div class="popup-container" id="popupContainer">
Hover me to see popup
<div class="popup-content">
Hey Geek,
<strong>
Welcome to GeeksforGeeks!
</strong>
</div>
</div>
<script>
const popupContainer =
document.getElementById('popupContainer');
const popupContent =
document.querySelector('.popup-content');
popupContainer.onmouseenter =
function () {
popupContent.style.display = 'block';
};
popupContainer.onmouseleave =
function () {
popupContent.style.display = 'none';
};
</script>
</body>
</html>
Output:
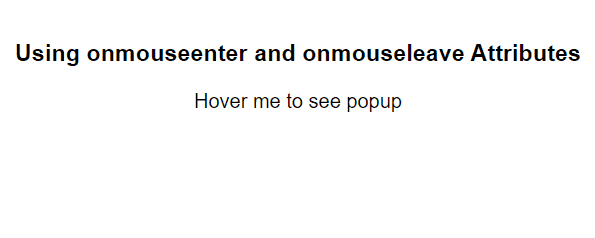
Share your thoughts in the comments
Please Login to comment...