How to Open a Popup on Click using JavaScript ?
Last Updated :
23 Apr, 2024
Opening a Pop-up when the click action is performed is the user-interactive technique. We can use this functionality in messages, or forms to show some error or alert messages before the user moves to the next action.
In this approach, we are using the display property in JavaScript to toggle the visibility of the overlay and popup dialog elements. The popupFn() and closeFn() functions dynamically set the display property to ‘block‘ for opening and ‘none‘ for closing, performing a popup effect on button click.
Syntax:
document.getElementById("element").
style.display = "none";
Example: The below example uses the display property to open a popup on click using JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
#popupDialog {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
background-color: #fff;
border: 1px solid #ccc;
box-shadow: 0 4px 8px
rgba(0, 0, 0, 0.1);
z-index: 1000;
}
#overlay {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.5);
z-index: 999;
}
h1 {
color: green;
}
</style>
</head>
<body>
<h1>GeeksforGeeks</h1>
<h3>Using display property</h3>
<button onclick="popupFn()">
Open Popup
</button>
<div id="overlay"></div>
<div id="popupDialog">
<p>Welcome to GeeksforGeeks</p>
<button onclick="closeFn()">
Close
</button>
</div>
<script>
function popupFn() {
document.getElementById(
"overlay"
).style.display = "block";
document.getElementById(
"popupDialog"
).style.display = "block";
}
function closeFn() {
document.getElementById(
"overlay"
).style.display = "none";
document.getElementById(
"popupDialog"
).style.display = "none";
}
</script>
</body>
</html>
Output:
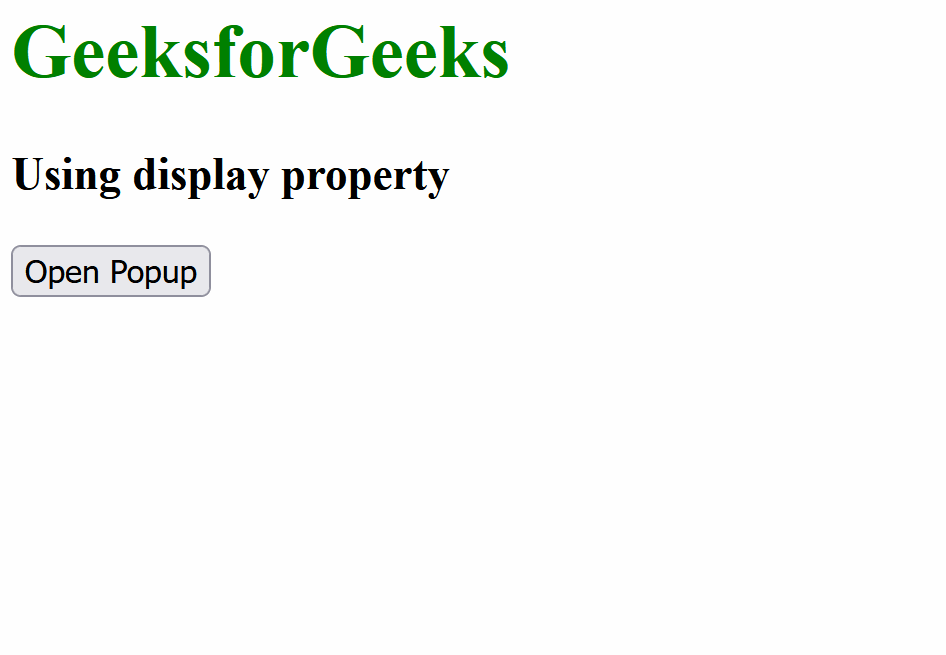
In this approach, we will use the classList property with toggle() method in JavaScript to toggle the visibility of the overlay and popup dialog elements. The openFn() function dynamically adds or removes the ‘hidden‘ class, while adjusting the opacity for a smooth transition, which results in a popup on button click.
Syntax:
const elementClasses =
elementNodeReference.classList;
Example: The below example uses the classList property to open a popup on click using JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f4f4f4;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
#popupContainer {
position: relative;
}
#overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.5);
z-index: 999;
display: none;
}
#popupDialog {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 0 20px
rgba(0, 0, 0, 0.3);
z-index: 1000;
opacity: 0;
transition: opacity 0.3s
ease-in-out;
}
h1 {
color: #4caf50;
margin-bottom: 20px;
}
p {
color: #333;
margin-bottom: 15px;
}
button {
padding: 10px 20px;
background-color: #4caf50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
transition: background-color 0.3s
ease-in-out;
}
button:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<div id="popupContainer">
<h1>GeeksforGeeks</h1>
<h3>Using classList Property</h3>
<button onclick="openFn()">
Open Popup
</button>
<div
id="overlay"
class="hidden"
></div>
<div id="popupDialog" class="hidden">
<p>
Welcome to a more stylish
GeeksforGeeks experience!
</p>
<button onclick="openFn()">
Close
</button>
</div>
</div>
<script>
function openFn() {
const over =
document.getElementById(
"overlay"
);
const popDialog =
document.getElementById(
"popupDialog"
);
over.classList.toggle("hidden");
popDialog.classList.toggle(
"hidden"
);
popDialog.style.opacity =
popDialog.style.opacity ===
"1"
? "0"
: "1";
}
</script>
</body>
</html>
Output:
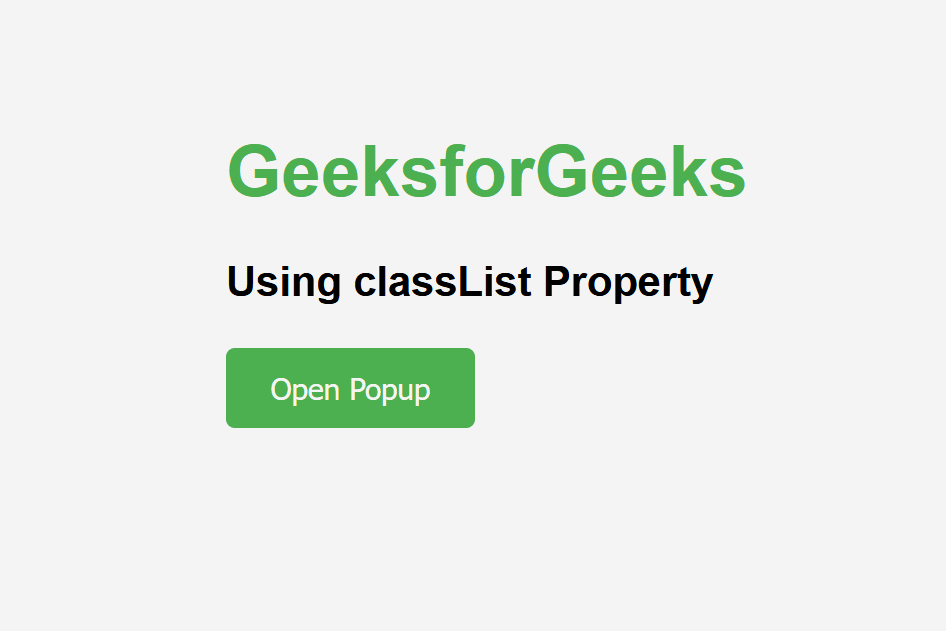
In this approach we are using the visibility property in CSS to toggle the visibility of a popup dialog. When the “Open Popup” button is clicked, the openPop() function is called. This function checks the current visibility state of the popup and toggles it between visible and hidden.
Syntax:
const elementClasses =
document.getElementById('elementClasses');
elementClasses.style.visibility =
(elementClasses.style.visibility === 'visible') ? 'hidden' : 'visible';
Example: The below example uses the visibility property to open a popup on click using JavaScript.
JavaScript
<!DOCTYPE html>
<html>
<head>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f4f4f4;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
#popupDialog {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 0 20px
rgba(0, 0, 0, 0.3);
z-index: 1000;
visibility: hidden;
}
h1 {
color: #4caf50;
margin-bottom: 20px;
}
p {
color: #333;
margin-bottom: 15px;
}
button {
padding: 10px 20px;
background-color: #4caf50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
transition: background-color 0.3s
ease-in-out;
}
button:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<div id="popupContainer">
<h1>GeeksforGeeks</h1>
<h3>Using visibility Property</h3>
<button onclick="openPop()">
Open Popup
</button>
<div id="popupDialog">
<p>Welcome to GeeksforGeeks!</p>
<button onclick="openPop()">
Close
</button>
</div>
</div>
<script>
function openPop() {
const popDialog =
document.getElementById(
"popupDialog"
);
popDialog.style.visibility =
popDialog.style.visibility ===
"visible"
? "hidden"
: "visible";
}
</script>
</body>
</html>
Output:
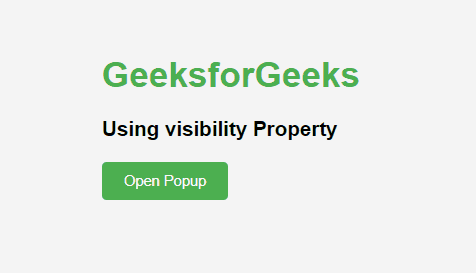
Share your thoughts in the comments
Please Login to comment...