Python Tornado – Asynchronous Networking
Last Updated :
29 Apr, 2024
Traditional synchronous networking models often struggle to keep pace with the demands of modern applications. Enter Tornado-Asynchronous networking, a paradigm-shifting approach that leverages non-blocking I/O to create lightning-fast, highly scalable network applications. In this article, we will learn about Python Tornado – asynchronous networking.
What is Python Tornado – Asynchronous Networking?
Tornado is a Python web framework and asynchronous networking library that has gained significant popularity due to its ability to handle thousands of simultaneous connections with ease. At its core, Tornado utilizes an event-driven architecture, allowing it to handle I/O operations asynchronously without the need for multithreading.
Python Tornado – Asynchronous Networking
Below are the Examples of Tornado-Asynchronous Networking in Python:
- HTTP Server Example
- Asynchronous HTTP Client Example
HTTP Server Example
This example demonstrates how to create a basic HTTP server using Tornado. By leveraging Tornado’s asynchronous capabilities, the server can handle multiple concurrent requests without blocking.
Python3
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.write("Hello, Tornado!")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8888)
tornado.ioloop.IOLoop.current().start()
Output
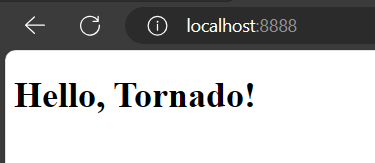
Asynchronous HTTP Client Example
Here, we define an asynchronous HTTP client using Tornado. The fetch_url function asynchronously retrieves the contents of a given URL without blocking the event loop, allowing for efficient handling of multiple requests.
Python3
import tornado.ioloop
import tornado.httpclient
async def fetch_url(url):
http_client = tornado.httpclient.AsyncHTTPClient()
response = await http_client.fetch(url)
print(response.body)
if __name__ == "__main__":
tornado.ioloop.IOLoop.current().run_sync(
lambda: fetch_url("https://example.com"))
Output:
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
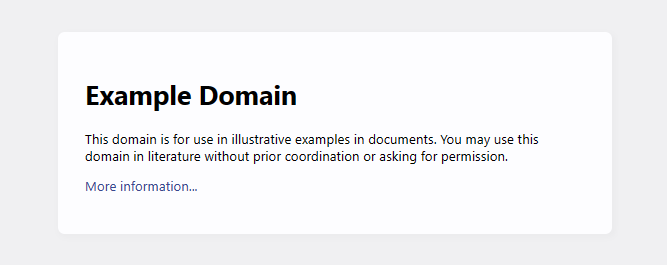
Web Example Using Tornado-Asynchronous networking
Below are the Web example of Tornado-Asynchronous networking:
File Structure
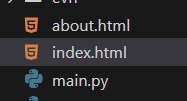
main.py :Â Below, code defines a Tornado web application with two routes: “/” handled by MainHandler and “/about” handled by AboutHandler. MainHandler renders “index.html” with a message, while AboutHandler renders “about.html”. When executed, the Tornado server listens on port 8888 and prints a message indicating its running status.
Python3
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.render("index.html", message="Hello, Tornado!",
host=self.request.host)
def post(self):
self.redirect("/about")
class AboutHandler(tornado.web.RequestHandler):
def get(self):
self.render("about.html")
def post(self):
self.redirect("/")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
(r"/about", AboutHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8888)
print("Tornado server running at http://localhost:8888")
tornado.ioloop.IOLoop.current().start()
Creating GUI
index.html : Below HTML code defines a webpage with the title “Hello Tornado!”. It includes a header displaying a message variable and a paragraph displaying the host variable. Additionally, there’s a form with a button that redirects to the “/about” route when submitted. The stylesheet “styles.css” is linked for styling purposes.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Tornado!</title>
<link rel="stylesheet" type="text/css" href="/static/styles.css">
</head>
<body>
<div class="container">
<h1>{{ message }}</h1>
<p>Host Address: {{ host }}</p>
<form action="/" method="post">
<button type="submit">Go to About</button>
</form>
</div>
</body>
</html>
about.html: Below, HTML code defines a webpage titled “About Us”. It contains a header displaying “About Us”, a paragraph describing the page content, and a form with a button that redirects to the “/about” route when submitted. The stylesheet “styles.css” is linked for styling purposes.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>About Us</title>
<link rel="stylesheet" type="text/css" href="/static/styles.css">
</head>
<body>
<div class="container">
<h1>About Us</h1>
<p>This is a simple Tornado web application.</p>
<form action="/about" method="post">
<button type="submit">Go to Home</button>
</form>
</div>
</body>
</html>
Run the Server
python main.py
Output:
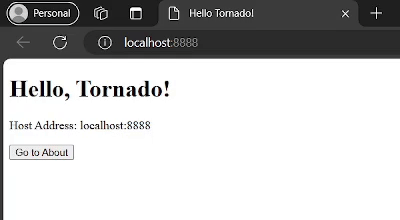
Advantages of Tornado Asynchronous Networking
The advantages of Tornado-Asynchronous networking are numerous:
- Scalability: Tornado’s event-driven architecture allows it to handle thousands of concurrent connections without sacrificing performance, making it ideal for high-traffic applications.
- Efficiency: By eliminating the need for thread-based concurrency, Tornado reduces overhead and resource consumption, resulting in faster response times and lower latency.
- Simplicity: Tornado’s straightforward API and asynchronous programming model make it easy to develop and maintain complex network applications.
- Robustness: Tornado provides built-in support for features such as websockets, HTTP/2, and secure connections, enabling developers to build robust and feature-rich applications with ease.
Share your thoughts in the comments
Please Login to comment...