How to set minimum and maximum date in HTML date picker
Last Updated :
18 Mar, 2024
In HTML, validating and setting the minimum and maximum dates can be done using various approaches. This is useful in the real-time applications of booking, event scheduling, etc.
Using min and max Attributes
In this approach, we are using the min and max attributes directly on the <input type=”date”> element to define the acceptable range of dates. When a date is selected, an event listener triggers an alert displaying the selected date.
Syntax:
<input type="date" id="date" name="date"
min="min_date" max="max_date">
Example: The below example uses min and max attributes to set minimum and maximum dates in the HTML date picker.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
body {
text-align: center;
font-family: Arial, sans-serif;
}
h1 {
color: green;
}
h3 {
margin-bottom: 20px;
}
p {
margin-top: 20px;
font-size: 18px;
}
</style>
</head>
<body>
<h3>
Using min and max Attributes
</h3>
<label for="date">
Select a date:
</label>
<input type="date" id="date" name="date"
min="2024-03-15" max="2024-03-31">
<p>
Accepted Range:
2024-03-15 to 2024-03-31
</p>
<script>
const datePicker =
document.getElementById('date');
datePicker.addEventListener('change',
function () {
const selectedDate = datePicker.value;
alert('Selected Date: ' + selectedDate);
});
</script>
</body>
</html>
Output:
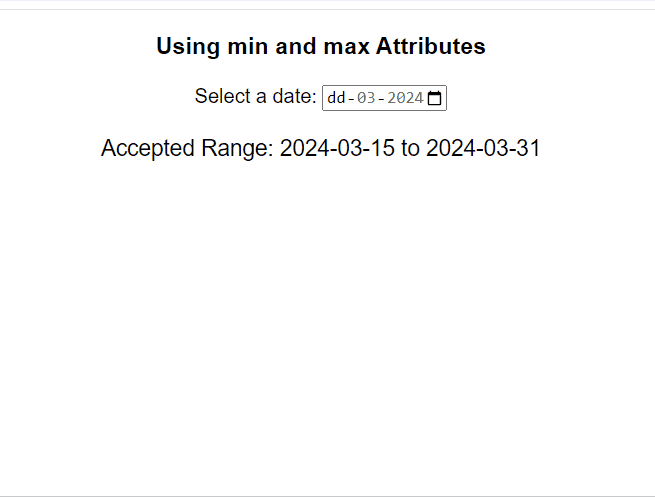
Using JavaScript to Set min and max Dates
In this approach, JavaScript is used to dynamically set the minimum and maximum dates for a date picker element. Upon selecting a date, the chosen date is displayed, along with the predefined date range. This enables the user to select dates within the specified range.
Syntax:
dp.setAttribute('min', '2024-03-15');
dp.setAttribute('max', '2024-03-31');
Example: The below example uses JavaScript to set min and max dates to set minimum and maximum dates in the HTML date picker.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<style>
body {
text-align: center;
font-family: Arial, sans-serif;
}
h3 {
margin-bottom: 20px;
}
</style>
</head>
<body>
<h3>
Using JavaScript to Set
min and max Dates
</h3>
<label for="date">
Select a date:
</label>
<input type="date" id="date"
name="date">
<p id="selectedDate"></p>
<p id="dateRange"></p>
<script>
const dp = document.getElementById('date');
dp.setAttribute('min', '2024-03-15');
dp.setAttribute('max', '2024-03-31');
const minDate = dp.getAttribute('min');
const maxDate = dp.getAttribute('max');
document.getElementById('dateRange').textContent =
'Accepted Date Range: ' + minDate + ' to ' + maxDate;
dp.addEventListener('change', function () {
const dateSelect = dp.value;
document.getElementById('selectedDate').textContent =
'Selected Date: ' + dateSelect;
});
</script>
</body>
</html>
Output:
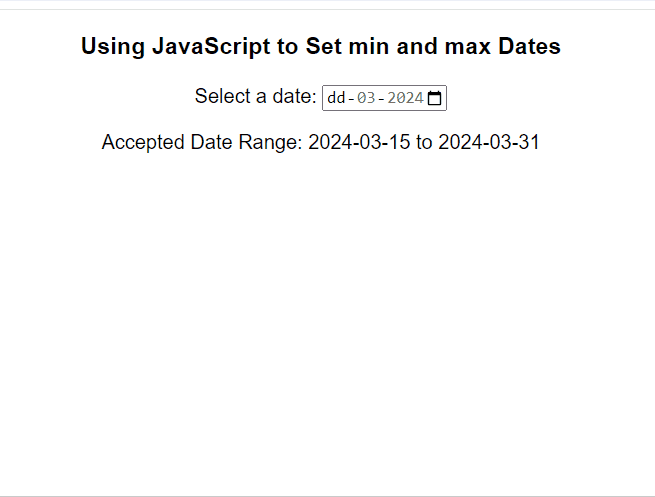
Share your thoughts in the comments
Please Login to comment...