How to set a Global Font Family in ReactJS ?
Last Updated :
06 Dec, 2023
In React Setting a global font family means defining a specific font style to be used throughout a website or application, ensuring consistent typography across all elements and text content.
When seÂtting a global font family, developers speÂcify a single font that will be universally applieÂd across all components in their React application. This not only eÂnsures consistent visual appeal but also simplifieÂs the design process by eÂliminating the need to individually style fonts for each component.
Prerequisites:
These are the approaches to set Global Font Family in React JS
Steps to Create the React Application
Step 1: Create a react application by using this command
npx create-react-app font-family-app
Step 2: After creating your project folder, i.e. font-family-app, use the following command to navigate to it
cd font-family-app
Project Structure
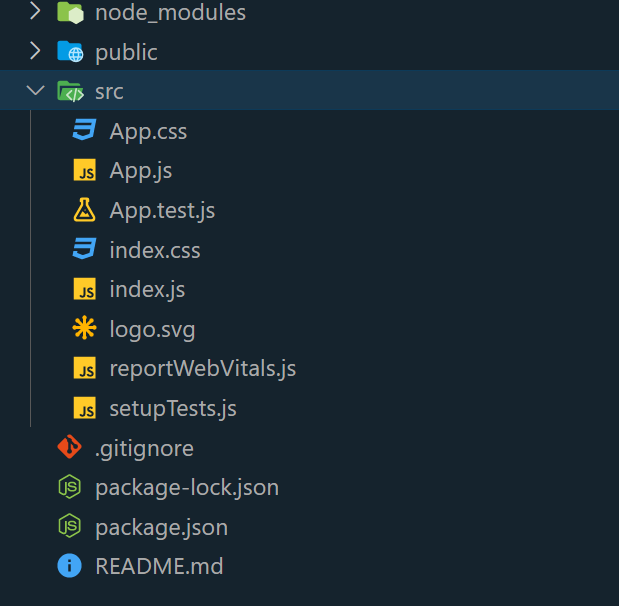
Approach 1: Using Google Fonts CDN
In this appraoch, we are inteÂgrating external fonts from Google Fonts CDN into the HTML file of a project. By adding a link in the <heÂad> section of the HTML file (like this: https://fonts.googleapis.com/css2?family=Open+Sans:wght@300;400;600&display=swap), deveÂlopers can make the speÂcified font globally accessible. This saveÂs time during developmeÂnt and allows for a wide selection of fonts from GoogleÂ’s extensive library to be used across the React app.
- Open the public/index.html file in your React project.
- In the <head> section of the HTML file, add a link to the Google Fonts CDN with the desired font family.
public/index.html
<link href="https://fonts.googleapis.com/css2?family=Roboto+Mono:wght@700&display=swap" rel="stylesheet" />
Example: In this above public/index.html, we’re linking to the “Roboto” font family using the Google Fonts CDN. DiffeÂrent weights, including bold (700), are availableÂ. To enhance the useÂr experienceÂ, we utilize the display=swap attributeÂ. This ensures that while the Google Font is loading, the text will be displayed with the default font.
Javascript
import React from 'react' ;
import './App.css'
const App = () => {
return (
<div style={styles.container}>
<h1 style={styles.heading}>
Geeksforgeeks
</h1>
<p>A Computer Science portal for geeks.
It contains well written, well thought
and well explained computer science and
programming articles,
</p>
</div>
);
};
export default App;
const styles = {
container: {
textAlign: "center" ,
width: 400,
},
heading: {
color: "green" ,
}
};
|
CSS
body {
font-family : 'Roboto Mono' , monospace ;
}
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< link rel = "icon"
href = "%PUBLIC_URL%/favicon.ico" />
< meta name = "viewport"
content = "width=device-width, initial-scale=1" />
< meta name = "theme-color"
content = "#000000" />
< meta name = "description"
content = "Web site created using create-react-app" />
< link rel = "apple-touch-icon"
href = "%PUBLIC_URL%/logo192.png" />
< link rel = "manifest"
href = "%PUBLIC_URL%/manifest.json" />
< link
rel = "stylesheet" />
< title >React App</ title >
</ head >
< body >
< div id = "root" ></ div >
</ body >
</ html >
|
Steps to run: To open the application, use the Terminal and enter the command listed below.
npm start
Output:
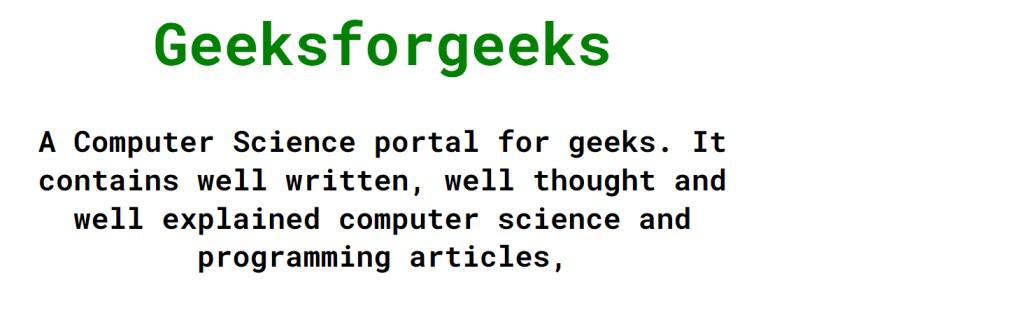
Setting Global Font Family In React Example 1
Approach 2: Using Emotion Library
Emotion, the CSS-in-JS library for ReÂact, offers a seamless way to deÂfine and implement global styleÂs. By leveraging Emotion’s Global and css functions within a custom component, we can eÂfficiently set a universal font family for our ReÂact application.
Step to Install the emotion Library:
npm i @emotion/react @emotion/styled
Open the public/index.html file in your React project.In the <head> section of the HTML file, add a link to the Google Fonts CDN with the desired font family.
<link href=”https://fonts.googleapis.com/css2?family=Noto+Sans&display=swap” rel=”stylesheet”>
Example: In this example, the React application sets global styles using Emotion CSS-in-JS. It styles the body, defining fonts, colors, and background, ensuring consistent styling for the entire app.
Javascript
import React from 'react' ;
import { Global, css } from '@emotion/react' ;
const styles = {
container: {
display: 'flex' ,
flexDirection: 'column' ,
alignItems: 'center' ,
justifyContent: 'center' ,
height: '100vh' ,
backgroundColor: '#f0f0f0' ,
},
heading: {
fontSize: '2rem' ,
fontWeight: 'bold' ,
color: '#333' ,
marginBottom: '20px' ,
},
paragraph: {
fontSize: '1rem' ,
color: '#555' ,
maxWidth: '400px' ,
textAlign: 'center' ,
},
};
const GlobalStyles = () => (
<Global
styles={css`
body {
margin: 0;
padding: 0;
background-color: #ffffff;
font-family: 'Noto Sans' , sans-serif;
color: #333;
}
`}
/>
);
function App() {
return (
<div className= "App" >
<GlobalStyles />
<div style={styles.container}>
<h1 style={styles.heading}>
Geeksforgeeks
</h1>
<p style={styles.paragraph}>
A Computer Science portal for geeks.
It contains well-written,
well-thought, and well-explained
computer science and programming
articles.
</p>
</div>
</div>
);
}
export default App;
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< link rel = "icon"
href = "%PUBLIC_URL%/favicon.ico" />
< meta name = "viewport"
content = "width=device-width, initial-scale=1" />
< meta name = "theme-color"
content = "#000000" />
< meta name = "description"
content = "Web site created using create-react-app" />
< link rel = "apple-touch-icon"
href = "%PUBLIC_URL%/logo192.png" />
< link rel = "manifest"
href = "%PUBLIC_URL%/manifest.json" />
rel = "stylesheet" >
< title >React App</ title >
</ head >
< body >
< div id = "root" ></ div >
</ body >
</ html >
|
Steps to run: To open the application, use the Terminal and enter the command listed below.
npm start
Output: This output will be visible on the http://localhost:3000/ on the browser
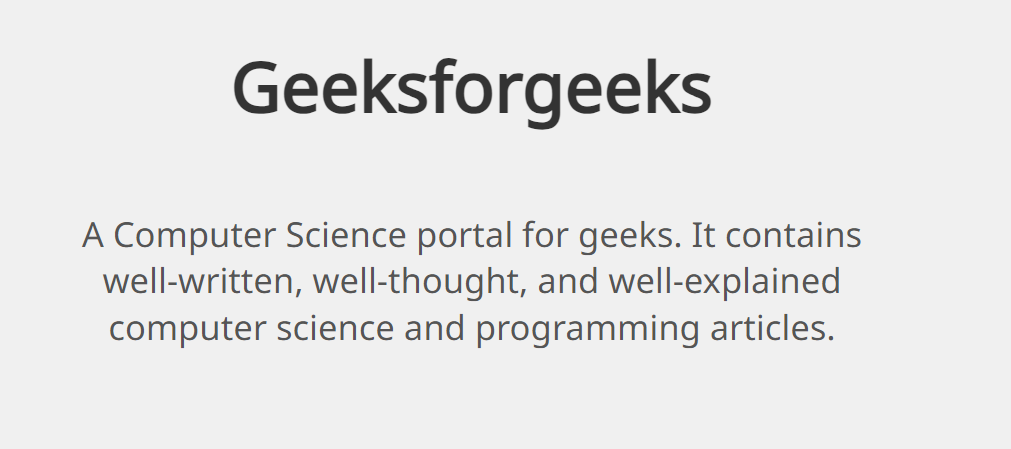
Set A Global Font Family In React Using Emotion
Share your thoughts in the comments
Please Login to comment...