How to Send FormData Objects with Ajax-requests in jQuery ?
Last Updated :
14 Jun, 2023
In this article, we will see how can we send formData objects with Ajax requests by using jQuery. To send formData, we use two methods, namely, the FormData() method and the second method is serialize() method. The implementation of Ajax to send form data enables the submission of data to the server without the need for a page refresh, which is a potent strategy applied in contemporary web-based applications to enhance the user’s interaction. The collection and transmission of form data with Ajax requests use FormData objects.
Syntax:
$.ajax({
url: "your-url",
type: "POST",
data: formDataObject,
processData: false,
contentType: false,
success: function(response){
// Handle success response
},
error: function(xhr, textStatus, error){
// Handle error response
}
});
Approach 1: The FormData() simplifies handling form data in JavaScript and jQuery by providing an easy way to construct and manage data from HTML forms, handle files and other complex form data, and automatically set the `Content-Type` header based on the content.
Example: In this example, this code shows how to use jQuery and Ajax to send form data to a server. The HTML form has four input fields (username, last name, address, and email), a submit button, and a unique ID “myForm”. The JavaScript code selects a form element by ID and sends its data as an Ajax request to “https://jsonplaceholder.cypress.io/posts” using jQuery’s $.ajax() function post method. Form data is sent using the “data” parameter of the Ajax request with disabled “processData” and “contentType” parameters. If the form is submitted, then it will show a success alert otherwise it will show an error message.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< script src =
</ script >
< style >
body {
margin: 3rem;
}
#myForm {
width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 5px;
}
label {
display: block;
margin-bottom: 10px;
}
input[type="text"],
input[type="email"] {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border: 1px solid #ddd;
border-radius: 5px;
font-size: 16px;
box-sizing: border-box;
}
button[type="submit"] {
display: block;
width: 100%;
padding: 10px;
background-color: green;
color: #fff;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: rgb(22, 138, 22);
}
</ style >
</ head >
< body >
< form id = "myForm" >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h3 >
Send FormData objects with Ajax-requests
</ h3 >
< label for = "username" >
User Name:
</ label >
< input type = "text"
name = "username" >
< label for = "name" >
Name:
</ label >
< input type = "text"
name = "name" >
< label for = "address" >
Address:
</ label >
< input type = "text"
name = "address" >
< label for = "email" >
Email:
</ label >
< input type = "email"
name = "email" >
< button type = "submit" >
Submit
</ button >
</ form >
< script >
$(document).ready(function () {
$('#myForm').submit(function (event) {
event.preventDefault();
var form = document.getElementById('myForm');
var formData = new FormData(form);
$.ajax({
method: 'POST',
data: formData,
processData: false,
contentType: false,
success: function (response) {
alert('Your form has been sent successfully.');
},
error: function (xhr, status, error) {
alert('Your form was not sent successfully.');
console.error(error);
}
});
});
});
</ script >
</ body >
</ html >
|
Output:
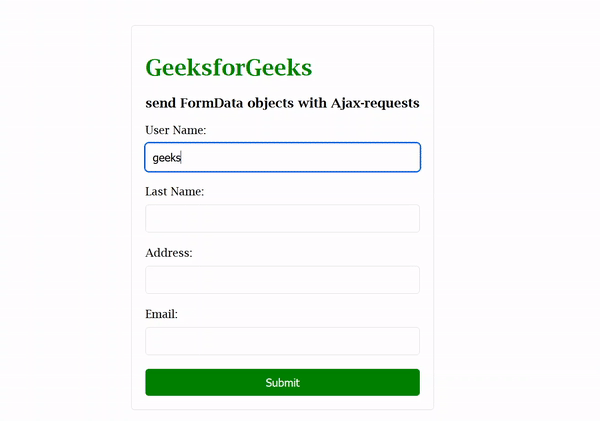
Approach 2: The jQuery method serialize() converts form elements into a string for HTTP requests. The serialize() method creates a URL-encoded query string from selected form elements in either a form element or a jQuery object.
Example: This example is very much similar but the difference is that in the previous example, we use FormData() to send a response but in this example, we use serialize() method to send the response.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< script src =
</ script >
< style >
body {
margin: 3rem;
}
#myForm {
width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 5px;
}
label {
display: block;
margin-bottom: 10px;
}
input[type="text"],
input[type="email"] {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border: 1px solid #ddd;
border-radius: 5px;
font-size: 16px;
box-sizing: border-box;
}
button[type="submit"] {
display: block;
width: 100%;
padding: 10px;
background-color: green;
color: #fff;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: rgb(22, 138, 22);
}
</ style >
</ head >
< body >
< form id = "myForm" >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h3 >
Send FormData objects with Ajax-requests
</ h3 >
< label for = "username" >User Name:</ label >
< input type = "text"
name = "username" >
< label for = "lastname" >Last Name:</ label >
< input type = "text"
name = "lastname" >
< label for = "address" >Address:</ label >
< input type = "text"
name = "address" >
< label for = "email" >Email:</ label >
< input type = "email"
name = "email" >
< button type = "submit" >Submit</ button >
</ form >
< script >
$(document).ready(function () {
$('#myForm').submit(function (e) {
e.preventDefault();
var formData = $(this).serialize();
$.ajax({
type: 'POST',
data: formData,
success: function (response) {
alert('Your form has been sent successfully.');
},
error: function (jqXHR, textStatus, errorThrown) {
alert('Your form was not sent successfully.');
}
});
});
});
</ script >
</ body >
</ html >
|
Output:
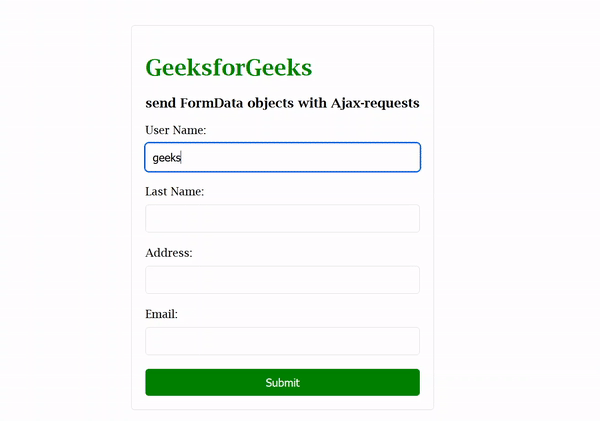
Share your thoughts in the comments
Please Login to comment...